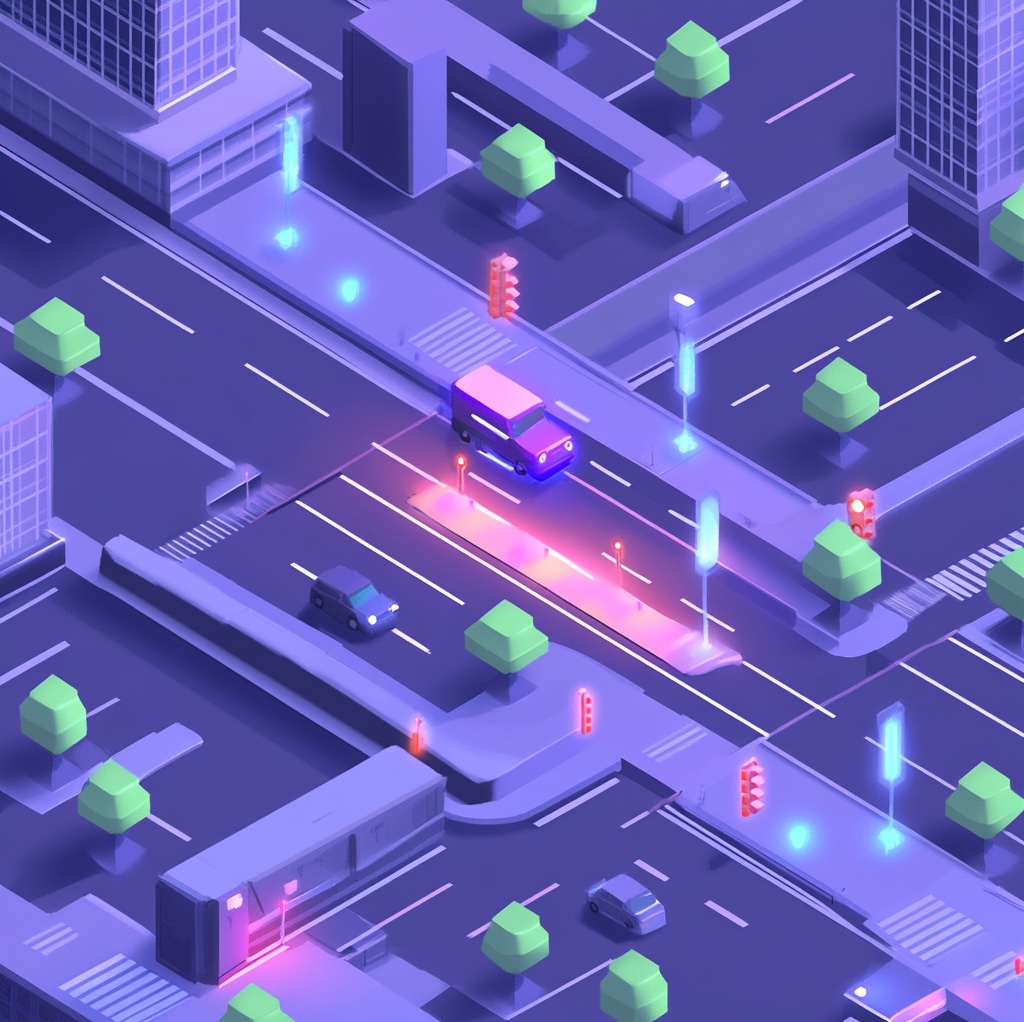
Using the Intersection Observer API in React for Dynamic Lazy Loading
Optimize performance and enhance user experience with intelligent lazy loading.
Aug 06, 2024 - 14:54 • 5 min read
When it comes to modern web development, performance and user experience are key. As applications become increasingly complex and resource-heavy, strategies that can effectively improve page loading times are essential. One of the most effective methods is lazy loading, which defers loading mode of assets until it's absolutely necessary. Enter the Intersection Observer API, a powerful tool for implementing lazy loading that many React developers may overlook.
Understanding the Intersection Observer API
The Intersection Observer API allows you to asynchronously observe the visibility of a target element in relation to an ancestor element or to the top-level document’s viewport. This means you can efficiently load images, script files, or components only when they are about to become visible in the user’s viewport, substantially enhancing application performance.
It allows you to create a callback function that runs whenever the target element intersects with the root, which is quite handy for operations like lazy loading and implementing infinite scrolling features. The API is supported in all modern browsers, making it a safe bet for your projects.
Setting Up the Intersection Observer in a React Component
First, let’s create a basic component that will utilize the Intersection Observer API for lazy loading an image. This is a practical implementation that illustrates the API’s use in a React functional component:
import React, { useEffect, useRef, useState } from 'react';
const LazyLoadImage = ({ src, alt }) => {
const [isVisible, setIsVisible] = useState(false);
const imgRef = useRef();
useEffect(() => {
const observer = new IntersectionObserver((entries) => {
entries.forEach((entry) => {
if (entry.isIntersecting) {
setIsVisible(true);
observer.unobserve(imgRef.current);
}
});
});
observer.observe(imgRef.current);
return () => {
if (imgRef.current) observer.unobserve(imgRef.current);
};
}, []);
return (
<div ref={imgRef} style={{ minHeight: '200px' }}>
{isVisible ? <img src={src} alt={alt} /> : <div>Loading...</div>}
</div>
);
};
export default LazyLoadImage;
Explanation of the Code
In this example:
- We have a basic
LazyLoadImage
component that takessrc
andalt
as props. - We create a state variable
isVisible
to manage whether the image should be shown. - We use
useRef
to access the DOM element we want to observe. - In the
useEffect
, we create a newIntersectionObserver
that will watch the image. Once the image enters the viewport, we setisVisible
to true and stop observing the element to prevent unnecessary calls.
Best Practices Here are some best practices when working with the Intersection Observer API in your React applications:
- Unobserve: Always ensure you unobserve your elements in the cleanup function of your effect to avoid memory leaks.
- Debouncing: Consider debouncing your callback function if it’s going to perform any expensive operations.
- Proper Fallbacks: Since the API may not work in some older browsers, ensure your application can still function without it—like providing graceful fallbacks or checks for feature support.
- Lazy Load Strategy: Decide on a lazy-loading threshold that best fits your design. For instance, you can pre-load images just before they enter the viewport for a better experience.
Enhancing the Lazy Loading Component
Building on our LazyLoadImage
component, we may want to extend its functionality to handle multiple images or even a grid layout. Let’s refactor it into a component that receives an array of images and displays them intelligently:
const LazyLoadImages = ({ images }) => {
return (
<div className="image-grid">
{images.map((image, index) => (
<LazyLoadImage key={index} src={image.src} alt={image.alt} />
))}
</div>
);
};
With this implementation, every LazyLoadImage
component operates independently and lazy loads its image when it enters the viewport.
Implementing Infinite Scrolling
In scenarios where you want to implement infinite scrolling, the Intersection Observer API shines through again. You could use it to load more content as the user approaches the bottom of a page. Here’s a simplified example:
const InfiniteScroll = () => {
const [items, setItems] = useState([...initialData]);
const [isLoading, setIsLoading] = useState(false);
const loadMoreRef = useRef();
useEffect(() => {
const observer = new IntersectionObserver((entries) => {
if (entries[0].isIntersecting && !isLoading) {
setIsLoading(true);
fetchMoreItems();
}
});
observer.observe(loadMoreRef.current);
return () => observer.unobserve(loadMoreRef.current);
}, [isLoading]);
const fetchMoreItems = async () => {
const newItems = await fetchData(); // Assume fetchData fetches more items
setItems((prevItems) => [...prevItems, ...newItems]);
setIsLoading(false);
};
return (
<div>
{items.map((item, index) => (<div key={index}>{item}</div>))}
{isLoading && <div>Loading more items...</div>}
<div ref={loadMoreRef} style={{ height: '20px' }}></div>
</div>
);
};
Conclusion
The Intersection Observer API is a powerful tool in a developer’s arsenal when it comes to optimizing web application performance. This approach to lazy loading images and implementing infinite scrolling enhances the overall user experience, making web pages feel faster and more responsive. By integrating this API into your React applications, you not only improve performance but also embrace a future-proof solution that scales effectively with your needs.
As we continue to strive for better applications in our ever-evolving digital landscape, harnessing the tools available, like the Intersection Observer API, will help us stay ahead of the curve. Start integrating lazy loading into your projects today for a more fluid and efficient user experience.