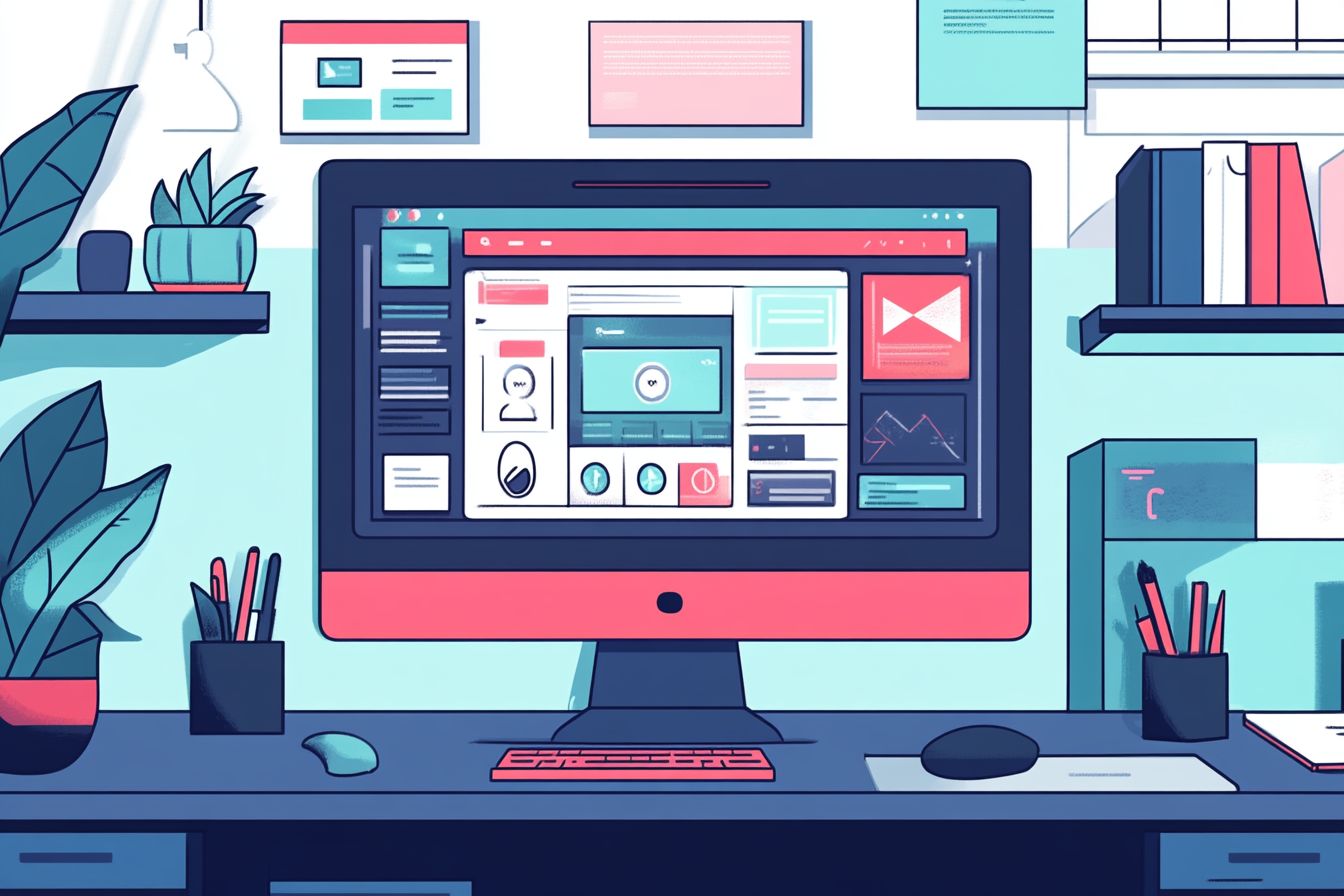
Optimizing React with Concurrent Features
Maximize Performance with Concurrent Mode in React
Aug 06, 2024 - 14:11 • 5 min read
In the evolving world of web development, React has continuously adapted to improve how developers can build high-performance applications. Among these enhancements, Concurrent Mode stands out as a way to optimize your React applications for responsiveness and interactivity, allowing you to create smoother user experiences. This blog post explores the intricacies of Concurrent Mode, its benefits, and how to effectively implement it in your React projects.
What is Concurrent Mode?
Concurrent Mode is a set of new features in React that enable React applications to prepare multiple tasks at once, instead of blocking the main thread for rendering. It allows React to interrupt rendering work, give updates quickly, and keep your application responsive to user interactions.
Here are some key features of Concurrent Mode:
- Interruptible Rendering: React can pause rendering to work on more important updates, like user interactions, and then return to the previous work.
- Prioritized Updates: Important updates can be prioritized using a concept called "Concurrency".
- Deferred Rendering: Components that are not immediately required for rendering can be deferred, leading to faster initial loads.
By utilizing these features, you can boost the performance of your application significantly, especially for applications with complex UIs or those that rely on asynchronous data fetching.
Enabling Concurrent Mode
To enable Concurrent Mode, you need to switch to the new root API in React 18. Here is how to set it up:
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
Once you switch to the createRoot
API, you’re able to use features like Concurrent Rendering
by simply wrapping your components with the new API.
Using Transitions in React
One of the most powerful features in Concurrent Mode is the ability to mark updates as transitions. This allows React to prioritize updates differently, providing a way to inform React which updates are more important and should be processed first.
Here’s an example that shows how to use the startTransition
API:
import { useState, startTransition } from 'react';
function App() {
const [inputValue, setInputValue] = useState('');
const [items, setItems] = useState([]);
const updateItems = (newValue) => {
// Marking the update as a transition
startTransition(() => {
setItems(newValue);
});
};
return (
<div>
<input
value={inputValue}
onChange={(e) => setInputValue(e.target.value)}
/>
<button onClick={() => updateItems([inputValue])}>Add Item</button>
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
</div>
);
}
In this example, when you call updateItems
, React treats that update as a transition, allowing the input to remain responsive while the items list updates in the background.
Suspense for Data Fetching
Concurrent Mode also enhances the way you can handle asynchronous data fetching in your application. With Suspense, you can tell React to wait for some condition (like data being fetched) before rendering a component. This works seamlessly with React.lazy()
for code-splitting as well.
Here’s how to achieve this:
import React, { Suspense } from 'react';
const Resource = (props) => {
const data = props.data; // assume data fetching logic here
return <div>{data}</div>;
};
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<Resource data={fetchData()} />
</Suspense>
);
}
The fallback prop allows you to manage loading states effortlessly while keeping your UI responsive.
Best Practices for Concurrent Mode
Leveraging Concurrent Mode effectively involves understanding the flow of tasks in your React application. Here are some best practices to consider:
- Prioritize Important Updates: Use
startTransition
for non-urgent updates to keep the UI responsive. - Utilize Suspense: Incorporate Suspense for data fetching to manage loading states intuitively.
- Break Down Large Components: Decompose complex UI hierarchies into smaller components for more efficient rendering.
- Test Performance: Regularly profile your application with React’s Profiler to identify any performance bottlenecks.
Common Pitfalls and How to Avoid Them
While adopting Concurrent Mode, developers often face similar challenges. Here are a few common pitfalls:
- Blocking Main Thread: Ensure that your updates don’t block the main rendering thread, causing jank. Utilize transitions effectively.
- Improper Handling of State Updates: Keep React’s concurrent behavior in mind when managing state mutations, especially with class components.
- Inefficient Data Fetching Logic: Ensure your data fetching is optimized to prevent unnecessary renders. Check your dependencies and data states thoroughly.
Conclusion
Concurrent Mode in React is a game-changer for developing high-performance web applications. By enabling seamless interactions, optimizing rendering paths, and decoupling UI from the underlying data synchronization logic, you can create applications that feel snappy and responsive.
As you incorporate these features, keep experimenting and profiling your application to extract the best performance from the Concurrent Mode. By understanding and leveraging the powerful tools React provides through Concurrent Mode, you can create a better experience for your users, ensuring your application meets their needs efficiently.
Stay tuned for further advancements in React as we continue to explore the exciting new features and best practices to optimize web applications!