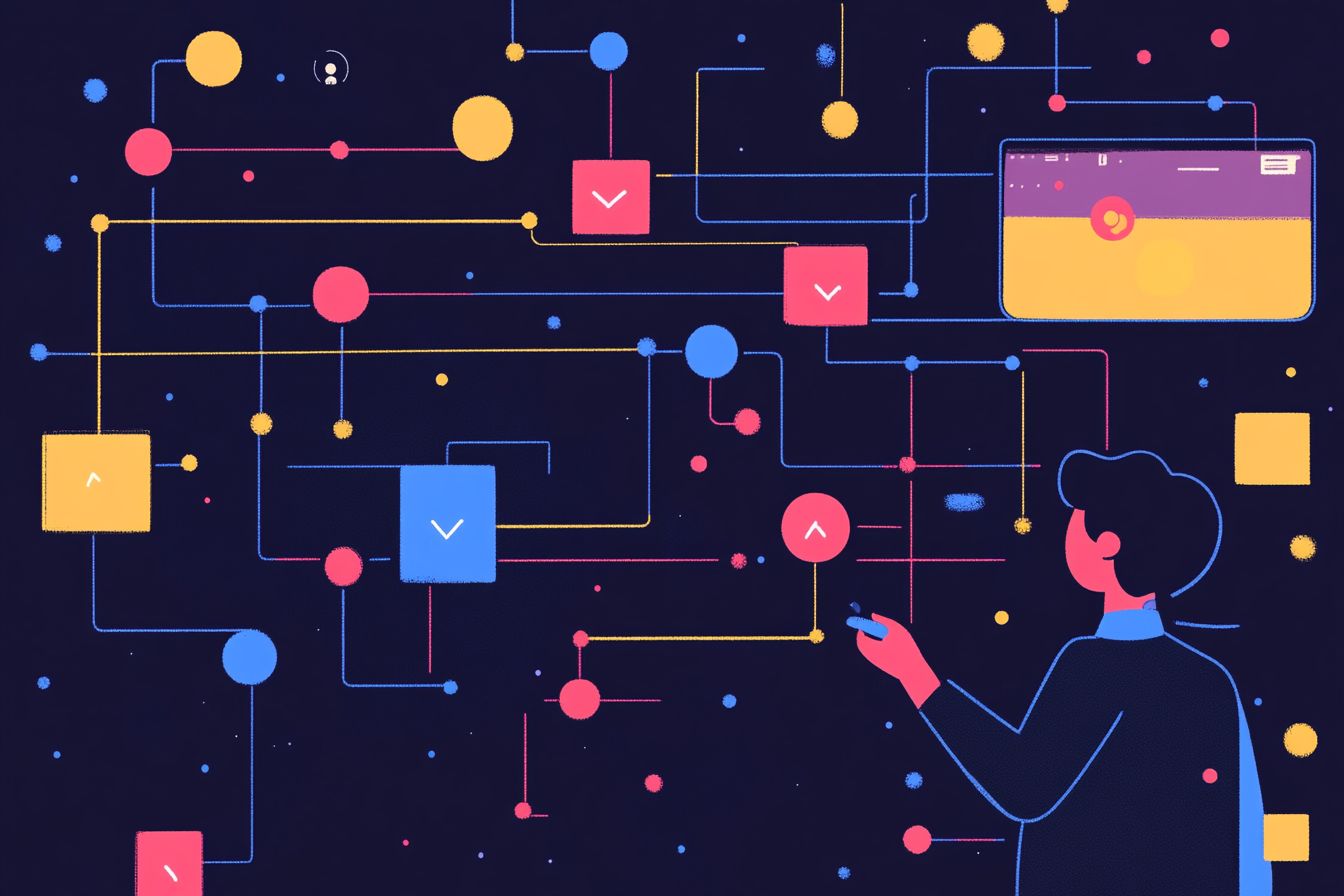
Managing State and Effects in React
Navigating the complexities of state management and side effects in React applications.
Aug 06, 2024 - 23:10 • 5 min read
The Complexities of Managing State and Effects in React
In the world of React, the separation of concerns can often become convoluted, especially when it comes to managing state and effects. Developers frequently find themselves grappling with the intricacies involved in synchronizing state variables with side effects. This post aims to delve into advanced patterns and techniques to streamline state management and effects handling.
Understanding the React Philosophy
At the core of React's design is a philosophy centered around declarative programming. The aim is to allow developers to describe what the UI should look like and let React manage the updates efficiently. However, as applications evolve, managing side effects can lead to state inconsistencies and performance issues.
In traditional class components, we relied heavily on lifecycle methods (componentDidMount
, componentDidUpdate
, componentWillUnmount
) to manage side effects. However, with the introduction of hooks, many of these effects and state management needs can be more elegantly handled. Still, there is a cost associated with adapting to this new paradigm, particularly in the area of effects.
function Example() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `Count: ${count}`;
}, [count]);
return <button onClick={() => setCount(count + 1)}>Increment</button>;
}
In the above example, the document title is updated every time the count changes. This approach is simple, but can become problematic when dealing with dependencies and conditions.
Common Pitfalls When Using useEffect
1. Outdated State References
An issue many encounter when using useEffect
is accessing stale closure state. Consider a scenario where we want to show a countdown timer:
function Countdown() {
const [count, setCount] = useState(10);
useEffect(() => {
const timer = setInterval(() => {
setCount(count - 1);
}, 1000);
return () => clearInterval(timer);
}, []);
return <h1>{count}</h1>;
}
Here, clicking "start" does nothing after the first update because the closure captures the initial state. To fix this, we can update the inner function to use the functional form of the setter:
setCount(c => c - 1);
2. Cleanup Issues
Another common issue is failing to clean up side effects properly when a component unmounts or updates.
For example, if we wish to fetch data when a component mounts, not cleaning up might lead to memory leaks or updates on unmounted components:
useEffect(() => {
let isMounted = true;
fetchData().then(data => {
if (isMounted) {
setData(data);
}
});
return () => { isMounted = false; };
}, []);
This cleanup method helps avoid inconsistent states that can occur if the component unmounts while fetching data.
Dynamic Effect Dependencies
One pattern that can beautifully streamline this process is to create custom hooks that abstract logic away from the component. Consider a custom hook for handling window size:
function useWindowSize() {
const [size, setSize] = useState({
width: window.innerWidth,
height: window.innerHeight,
});
useEffect(() => {
const handleResize = () => {
setSize({ width: window.innerWidth, height: window.innerHeight });
};
window.addEventListener('resize', handleResize);
return () => window.removeEventListener('resize', handleResize);
}, []);
return size;
}
Using this hook in a component becomes straightforward:
function App() {
const { width, height } = useWindowSize();
return <div>Width: {width}, Height: {height}</div>;
}
By decoupling logic into custom hooks, not only do we make our components cleaner and more manageable, but we also ensure better performance and state integrity.
Managing Multiple State Updates
When dealing with complex components with multiple states, managing when and how updates occur can quickly spiral into a headache. Effective patterns to consider include:
- Batching State Updates: Utilize functional updates to prevent unnecessary re-renders and handle state updates more fluidly.
- Reducer Pattern with useReducer Hook: Use the
useReducer
hook when multiple pieces of state are interdependent:
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, { count: 0 });
return (
<>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</>
);
}
This provides a centralized way of handling state transitions without cluttering components.
Advanced Side Effects Handling
When performing data fetching or other asynchronous actions within effects, it's vital to architect these properly. Using async
functions can lead to complications due to the asynchronous nature of JavaScript. Instead of defining the effect as async
, one can create an inner function:
useEffect(() => {
let isMounted = true;
const fetchData = async () => {
const result = await fetch('/data');
if (isMounted) {
setData(result);
}
};
fetchData();
return () => { isMounted = false; };
}, []);
This ensures the component handles async actions while remaining resilient against unmounted state updates. Consider ensuring cancellation mechanisms for fetch requests using AbortController.
Conclusion
Effectively managing state and effects in React is both an art and a science. Through patterns such as custom hooks, the reducer pattern, and dynamic effect dependencies, developers can create more maintainable and efficient components. As React continues to evolve, so too should our practices in handling state and side effects, adhering to the principle that code should remain declarative, clear, and robust.
This thorough understanding of state and effects not only boosts performance but also leads to a more seamless development experience. Remember, React is an evolving ecosystem, and staying updated with trends and techniques is key to leveraging its full potential.