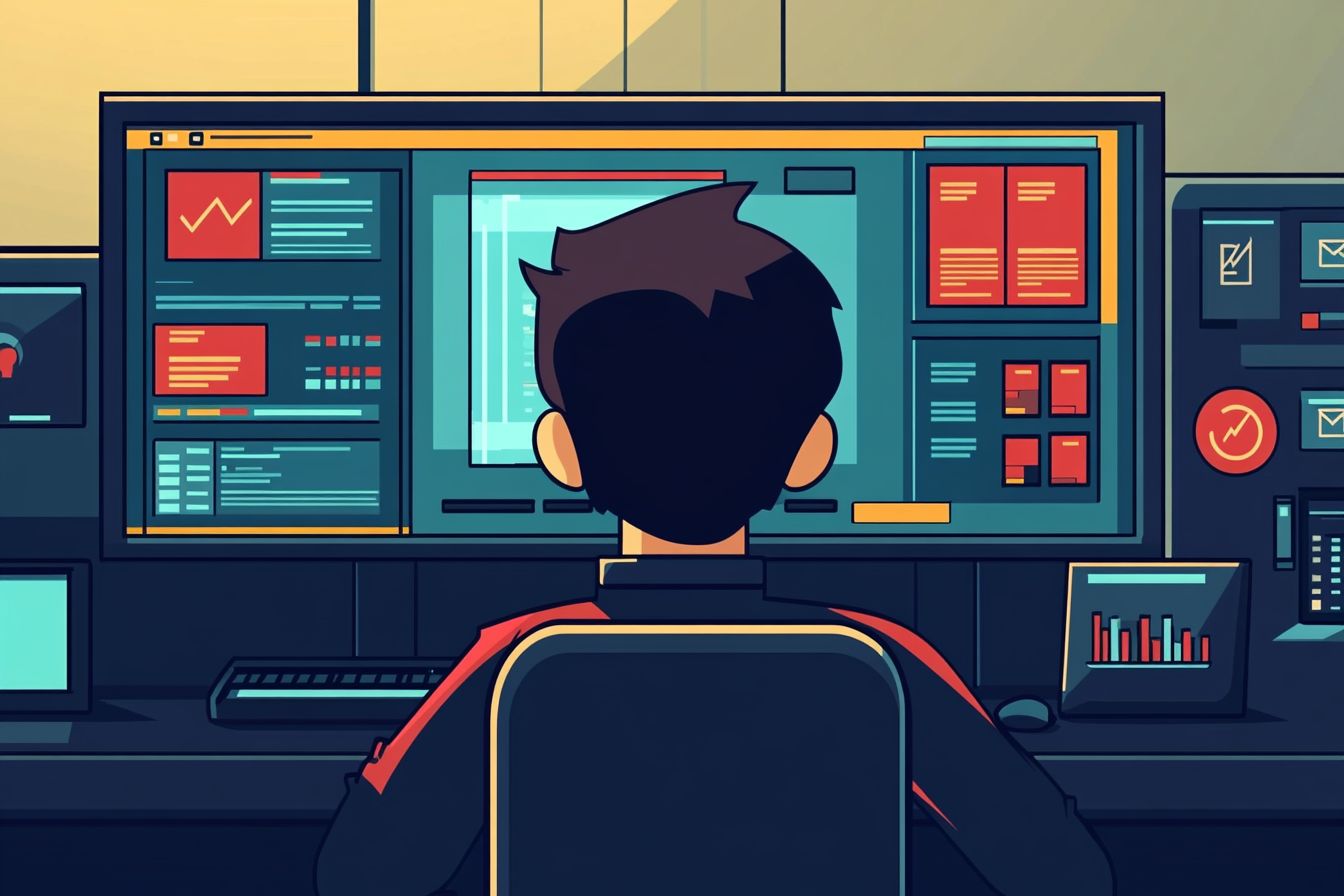
Understanding React's useLayoutEffect for Performance Optimization
Dive deep into the mechanics of useLayoutEffect and discover how it can boost your React apps' performance.
Aug 09, 2024 - 10:46 • 5 min read
The React Hooks API has revolutionized how we create components, providing a way for developers to encapsulate logic and state management in a more readable manner. Among the Hooks, useEffect
and useLayoutEffect
play critical roles in optimizing performance and rendering behavior in React applications. However, many developers may use useEffect
without fully understanding the intricacies of useLayoutEffect
and how it can significantly affect layout and rendering, particularly in complex UIs.
What is useLayoutEffect?
The useLayoutEffect
Hook is similar to useEffect
, but it fires synchronously after all DOM mutations. This means that it blocks the browser's painting process until the effect has finished execution. It is ideal for any operations that need to read layout from the DOM and re-render it. To clarify, this is especially useful if the effect updates the DOM in a way that requires an immediate visual update before the browser performs the paint.
Here is the basic syntax:
import { useLayoutEffect } from 'react';
function Example() {
useLayoutEffect(() => {
// Your code here
});
}
When to Use useLayoutEffect
Measuring Layout: If your effect is dependent on the layout of the page (e.g., reading dimensions of an element), you should use
useLayoutEffect
to make sure you read the dimensions before the browser has a chance to paint.For instance, if you have a modal that you want to center based on its dimensions, you could do the following:
import React, { useState, useLayoutEffect, useRef } from 'react'; function CenteredModal() { const modalRef = useRef(); const [modalStyle, setModalStyle] = useState({}); useLayoutEffect(() => { const modalDimensions = modalRef.current.getBoundingClientRect(); setModalStyle({ top: `${window.innerHeight / 2 - modalDimensions.height / 2}px`, left: `${window.innerWidth / 2 - modalDimensions.width / 2}px` }); }, []); return ( <div ref={modalRef} style={modalStyle} className="modal"> {/* Modal content */} </div> ); }
Avoiding Flash of Unstyled Content (FOUC): When you need to update the DOM based on some state or prop changes that might affect layout, it’s better to use
useLayoutEffect
to minimize the impact of paint.For instance, if you are implementing a responsive layout, using
useLayoutEffect
will ensure the layout calculations are finished before the browser renders the updates:import React, { useLayoutEffect, useState } from 'react'; function ResponsiveComponent() { const [width, setWidth] = useState(window.innerWidth); useLayoutEffect(() => { const handleResize = () => setWidth(window.innerWidth); window.addEventListener('resize', handleResize); return () => window.removeEventListener('resize', handleResize); }, []); return <div>{width > 600 ? 'Wide' : 'Narrow'}</div>; }
Differences between useLayoutEffect and useEffect
- Execution Timing:
useLayoutEffect
runs synchronously after all DOM mutations, whileuseEffect
runs asynchronously after rendering is completed and before the paint. - Use Cases: Use
useLayoutEffect
for operations that require reading DOM measurements and manipulating it immediately, while useuseEffect
for side-effects that can be executed after rendering, such as data fetching or subscriptions.
Performance Implications
Using useLayoutEffect
unnecessarily can lead to performance issues since it blocks painting. It’s crucial to only use it in situations that require immediate DOM measurements or updates. In cases where DOM read operations do not directly influence the rendering or layout, use useEffect
instead for better performance.
Here’s an example showing how both can coexist in a component:
import React, { useEffect, useLayoutEffect, useState } from 'react';
function Example() {
const [data, setData] = useState(null);
useLayoutEffect(() => {
console.log('This runs before the paint.');
}, []);
useEffect(() => {
fetch('/api/data')
.then(response => response.json())
.then(setData);
}, []);
return <div>{data ? data.content : 'Loading...'}</div>;
}
In this code, useLayoutEffect
runs first, ensuring that any DOM measurements needed for rendering occur before the paint, while useEffect
handles data fetching post-render, creating a more efficient utilization of resources.
Best Practices
- Minimize Usage: Only use
useLayoutEffect
when absolutely necessary. For most scenarios,useEffect
is sufficient and does not block paint. - Clean Up Effects: Always return a cleanup function from your effects to prevent memory leaks and ensure optimal performance.
- Testing with React DevTools: Utilize React’s built-in profiling tools to observe the performance implications of using
useLayoutEffect
versususeEffect
. - Avoid Complex Dependencies: Use simple dependencies in
useLayoutEffect
to prevent unnecessary re-renders and re-calculations, confirming constant layout models.
Conclusion
The meticulous distinction of when to use useLayoutEffect
versus useEffect
can significantly enhance the user experience through smoother and faster UI performance. Leveraging useLayoutEffect
gives developers the tools to create responsive applications that react fluidly to user interactions.
By deep understanding and judicious application of useLayoutEffect
, we as developers can continue crafting performant, user-friendly React applications that center around layout dynamics. As always, careful performance testing should guide our decisions before opting for useLayoutEffect
in development practices.
Additional Notes
This discussion opens the door to further inquiries regarding the React lifecycle methods and how they interplay with Hooks. Consider expanding your knowledge by diving into other advanced React topics such as context optimizations, memoization patterns, and concurrent rendering techniques to enhance even further your development skill set.