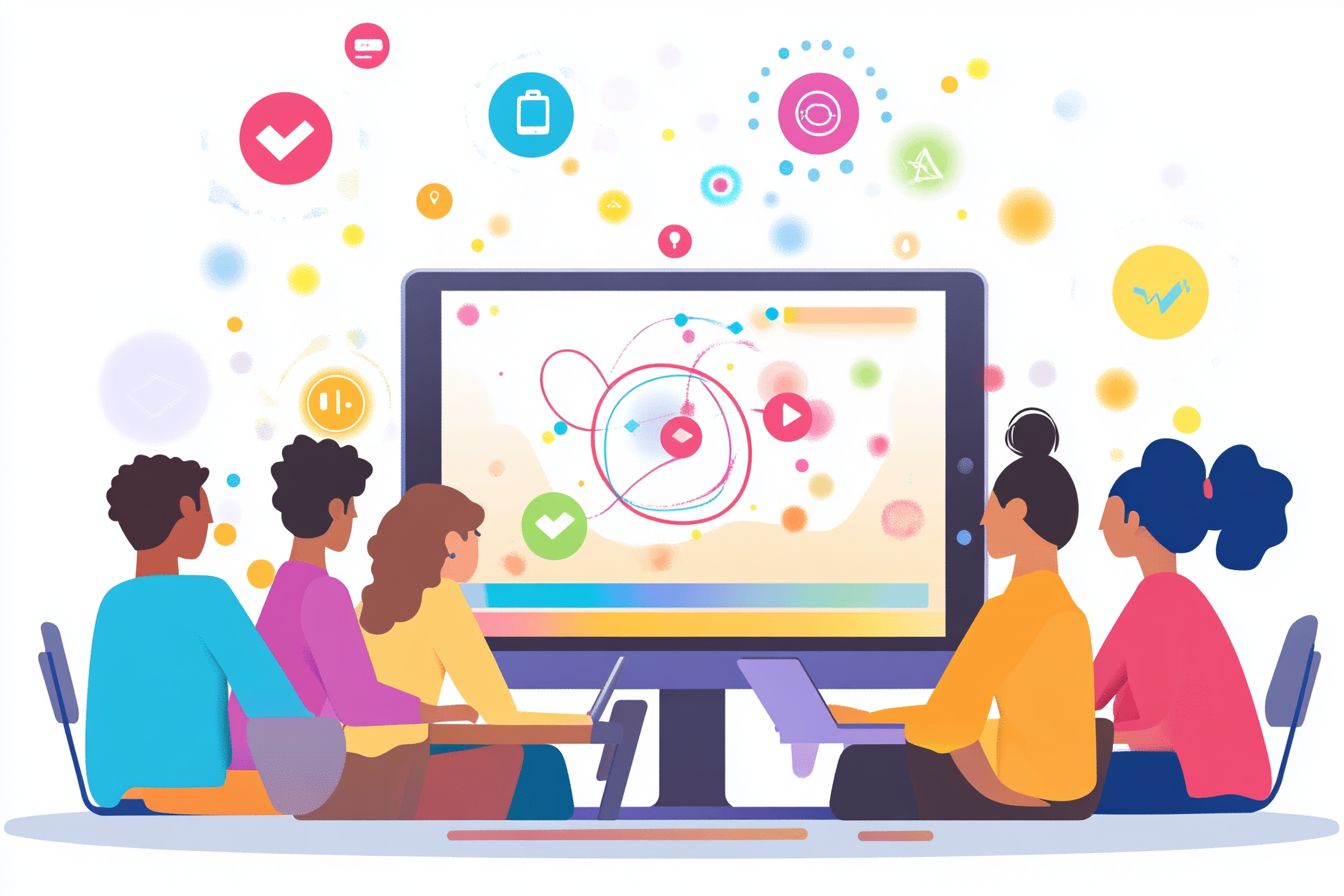
Understanding React Fragments for Cleaner Code
Master the use of React Fragments to optimize your component structure.
Aug 09, 2024 - 10:53 • 4 min read
In the realm of React development, optimizing component structure contributes significantly to enhancing the performance and readability of applications. One approach that is often overlooked is the effective use of React Fragments. By employing Fragments, developers can minimize unnecessary markup in their components, making the code cleaner and more manageable.
What are React Fragments?
React Fragments allow you to group a list of children without adding extra nodes to the DOM. This can significantly improve the performance and organization of your React components, especially when rendering lists or aggregating JSX elements.
Basic Syntax
The syntax for using Fragments is straightforward. You can use either the Fragment
component or an empty tag <>
to denote a Fragment. Here are two equivalent ways to implement React Fragments:
Using the Fragment Component:
import React from 'react';
const MyComponent = () => {
return (
<React.Fragment>
<h1>Hello Fragment</h1>
<p>This will be grouped together without additional DOM nodes.</p>
</React.Fragment>
);
};
Using Short Syntax:
import React from 'react';
const MyComponent = () => {
return (
<>
<h1>Hello Fragment</h1>
<p>This will also be grouped together without additional DOM nodes.</p>
</>
);
};
Both snippets above will render the h1
and p
elements without wrapping them in an extra <div>
or similar element, maintaining a flat structure in the DOM.
Benefits of Using React Fragments
1. Avoiding Extra Nodes
One of the primary benefits of using React Fragments is that you can avoid extra nodes in the DOM. This is particularly crucial when rendering a large number of components where the resulting DOM structure can quickly become cluttered. This clutter can lead to performance issues since browsers have to manage and render more elements.
2. Enhanced Readability
By using Fragments, you can create a more readable and simpler JSX tree. This is particularly useful when your component returns multiple root elements, as it allows you to group them logically within a Fragment, improving maintainability.
3. Simplifying Styles and Scripts
When you use extra wrapper elements, you might have to adjust styles or write additional JavaScript to manage the added complexity. Fragments alleviate this problem by enabling you to avoid unnecessary styles that might apply to wrapper components, leading to a more straightforward styling approach.
Key Takeaways on When to Use Fragments
While Fragments are extremely beneficial, they should be used cautiously. Here are some scenarios where utilizing Fragments can greatly enhance your components:
Lists of Items
When rendering lists, it’s common to use extra <div>
tags to wrap items. Instead, consider using Fragments:
const listItems = items.map(item => {
return (
<React.Fragment key={item.id}>
<h2>{item.title}</h2>
<p>{item.description}</p>
</React.Fragment>
);
});
This method is efficient, keeps the structure clear, and reduces DOM overhead.
Grouping Multiple Elements
When you want to return multiple elements from a component, and they don't need to be wrapped in a specific HTML element, Fragments are an excellent choice. For instance:
const MultiElementComponent = () => {
return (
<>
<h1>This is the title</h1>
<p>This is a description.</p>
</>
);
};
Performance in Large Components
If a component involves multiple elements, using Fragments can improve performance as it eliminates unnecessary wrappers, thus optimizing the rendering speed.
Common Mistakes to Avoid
1. Not Providing Keys in Lists
When you create lists of Fragments, always remember to provide a unique key
prop to each Fragment for stability in rendering. For example:
const items = [1, 2, 3];
const itemComponents = items.map(item => (
<React.Fragment key={item}>
<p>Item {item}</p>
</React.Fragment>
));
This prevents React from re-rendering components unnecessarily, ensuring better performance.
2. Overusing Fragments
While Fragments are powerful, they should not be overused. Sometimes, it may make more sense to use a regular wrapper component to group items, especially when an element should be styled (e.g., using flexbox or grid).
Conclusion
React Fragments enhance the way components interact by allowing for cleaner, more efficient code without creating unnecessary DOM nodes. Understanding how to implement and when to use Fragments can dramatically simplify your React codebase, leading to improved performance and maintainability. As React continues to evolve, exploring features like Fragments and utilizing them effectively will be key in developing high-quality applications.
By harnessing React Fragments, you can successfully manage your component structures, especially as your project grows in size and complexity. Remember, less is often more in the world of web development, and Fragments embody that philosophy beautifully.