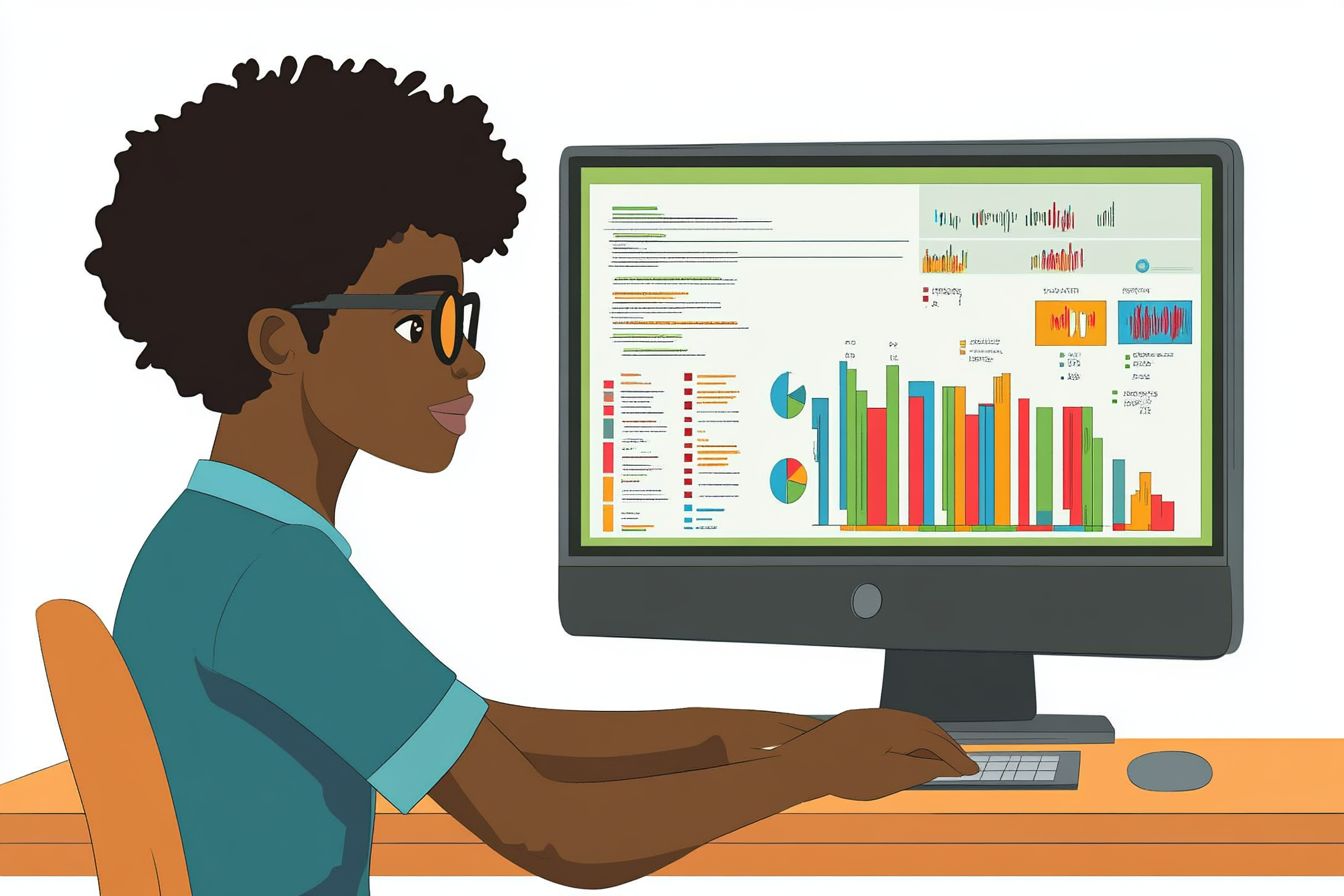
Understanding React's useEffect for Advanced Data Fetching
Harnessing Side Effects: Unlocking the Power of useEffect for Efficient Data Management
Jul 30, 2024 - 16:15 • 4 min read
Working with side effects in React is crucial for fetching data and managing asynchronous operations. One of the central hooks for handling side effects is useEffect
, yet its intricacies can often trip developers up, especially when dealing with async code, cleanup, and dependencies. In this post, we’ll explore how to harness useEffect
for efficient data fetching, optimizing its use with various implementation strategies, and avoiding common pitfalls.
Getting Started with useEffect
The useEffect
hook allows you to perform side effects in function components. It runs after every render by default, making it a powerful tool for fetching data from APIs or listening to events. The signature looks like this:
useEffect(() => {
// Your code here
}, [dependencies]);
Basic Data Fetching Example
Let’s start with a simple data fetching scenario. Consider the following function component which fetches user data from an API:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const UserProfile = () => {
const [user, setUser] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchUser = async () => {
try {
const response = await axios.get('https://api.example.com/user');
setUser(response.data);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchUser();
}, []); // Empty dependency array for one-time fetch
if (loading) return <div>Loading...</div>;
if (error) return <div>Error fetching user data</div>;
return <div>User: {user.name}</div>;
};
Here, useEffect
is set with an empty dependency array, meaning it will execute only once when the component is mounted. This is ideal for data fetching. However, be cautious of memory leaks if the component unmounts before the request completes. This can be addressed with cleanup functions.
Adding Cleanup Logic
If you want to manage subscriptions or requests that need to be canceled, useEffect
allows for cleanup. Here’s how you might implement it:
useEffect(() => {
const fetchUser = async () => {
try {
const response = await axios.get('https://api.example.com/user');
setUser(response.data);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchUser();
return () => {
// Any cleanup code can go here
};
}, []);
Using return
within useEffect
allows you to define a cleanup function that runs when the component is unmounted or if the effect is about to run again. This is critical for avoiding memory leaks, especially when working with asynchronous operations.
Handling Dependencies
The dependency array is one of the most critical elements of useEffect
. It tells React when to re-run the effect.
For example, to fetch user data dynamically based on some state:
const UserProfile = ({ userId }) => {
const [user, setUser] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchUser = async () => {
try {
const response = await axios.get(`https://api.example.com/user/${userId}`);
setUser(response.data);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchUser();
}, [userId]); // Runs when userId changes
// Rendering logic remains the same
};
Notably, if you forgot to include userId
in the dependency array, the effect wouldn’t respond to changes in userId
, potentially leading to stale data.
Common Pitfalls
Stale States: When using variables defined outside your effect, ensure they’re listed in the dependencies to avoid stale closures. Always use functional updates when setting states based on previous states:
setState(prev => prev + 1);
Infinite Loops: Carelessly setting states in
useEffect
without managing dependencies can lead to infinite re-renders. Always ensure that the state you’re setting doesn’t trigger another render unnecessarily.Unnecessary Fetching: If dependencies aren’t correctly managed, your request could be triggered far more often than expected, impacting performance.
Leveraging useCallback with useEffect
Using the useCallback
hook can enhance performance when passing functions as dependencies in useEffect
. Here’s how:
const fetchUser = useCallback(async () => {
// Your fetching logic
}, [userId]);
useEffect(() => {
fetchUser();
}, [fetchUser]);
This ensures that a fresh instance of fetchUser
doesn't get created on every render, thus optimizing the invocations of useEffect
.