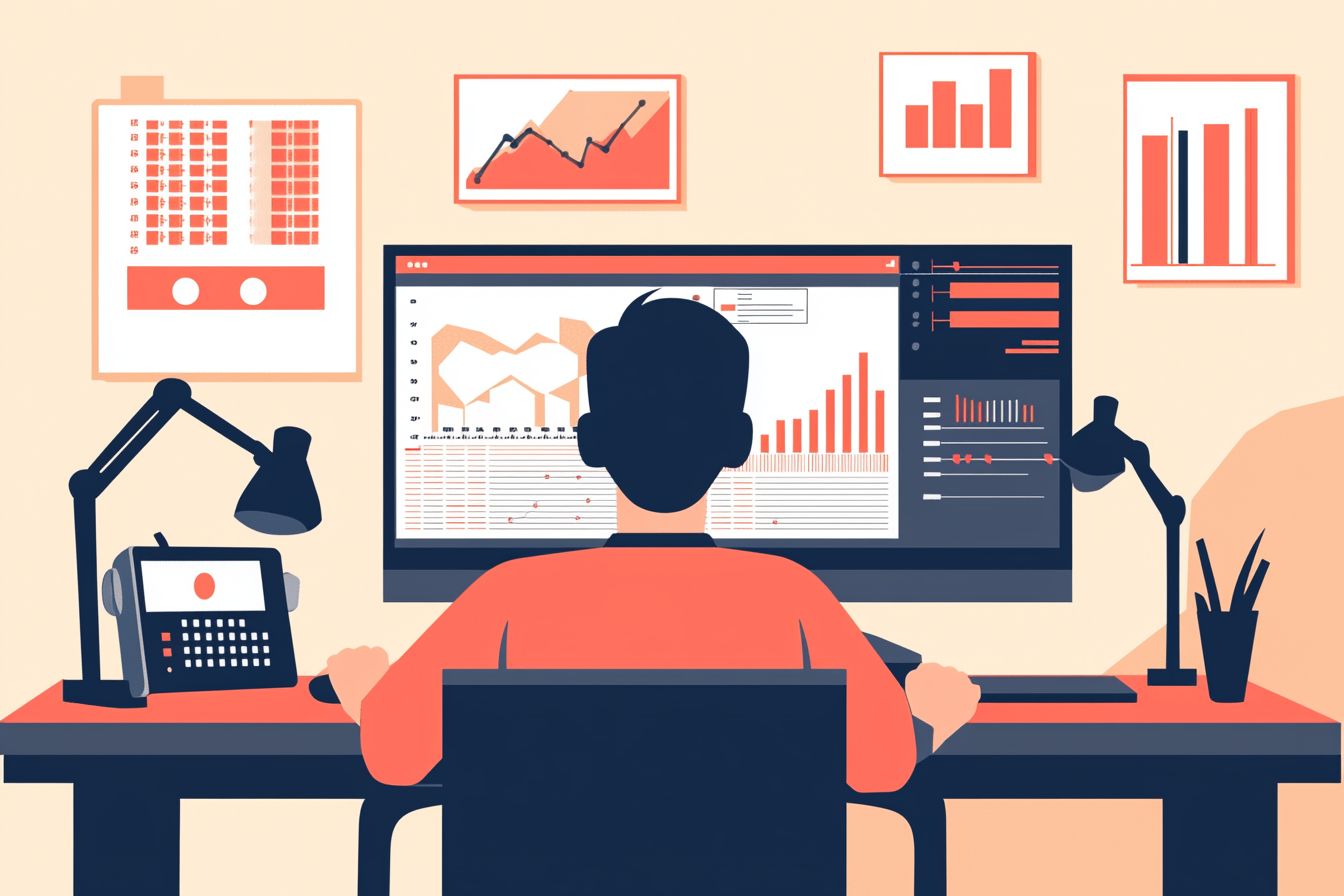
Deep Dive into React Query: Caching Strategies for Optimal Performance
Unlock the secrets of effective caching with React Query to supercharge your applications.
Aug 02, 2024 - 12:06 • 5 min read
Introduction
React Query has become a cornerstone for data fetching and state management in React applications. While its primary functionality revolves around simplifying data fetching, one of its most powerful features is its caching mechanism. In this post, we will explore advanced caching strategies and best practices with React Query to ensure optimal performance in your applications.
Understanding React Query's Caching Mechanism
React Query’s caching system is nuanced and can lead to significant performance boosts. It allows components to retrieve previously fetched data without the need for new network requests, thus enhancing user experience and application speed.
The Cache
The cache in React Query is an in-memory structure where all the server state data is stored. When a query is made, React Query first looks for the data in the cache before making a network request. This behavior can dramatically reduce loading times and minimize unnecessary network calls.
Stale Data
Data that has been fetched is classified under various states:
- Stale: Data that has reached its designated time limit after being fetched.
- Fresh: Data that is still considered valid for consumption by the application.
React Query uses an internal algorithm to determine when to consider data stale, which can be customized by the developer.
Configuring Cache Time
const { data } = useQuery('todos', fetchTodos, {
staleTime: 1000 * 60 * 5 // 5 minutes
});
In this example, the data will remain fresh for five minutes after being fetched. After this period, the data becomes stale, prompting React Query to refetch it if it's needed again.
Cache-First Strategies
Using the cache-first strategy efficiently is key to enhancing application performance. Here are some approaches for implementing effective cache-first strategies:
1. Optimistic Updates
When you perform a mutation with React Query, using optimistic updates helps in making the UI more responsive.
const mutation = useMutation(addTodo, {
// Optimistically update to the new value
onMutate: async (newTodo) => {
await queryClient.cancelQueries('todos');
const previousTodos = queryClient.getQueryData('todos');
queryClient.setQueryData('todos', (old) => [...old, newTodo]);
return { previousTodos };
},
onError: (err, newTodo, context) => {
queryClient.setQueryData('todos', context.previousTodos);
},
onSettled: () => {
queryClient.invalidateQueries('todos');
},
});
The onMutate
hook allows us to optimistically update the cache, immediately reflecting the changes in the UI without waiting for the server response.
2. Prefetching Data
React Query provides an efficient way to prefetch data that might be required soon, keeping the cache warmed up.
useEffect(() => {
queryClient.prefetchQuery('todos', fetchTodos);
}, []);
Prefetching ensures data is ready when the user navigates to a different route.
Utilizing the Query Client
The Query Client plays an essential role in managing queries and mutations, providing methods to manipulate the cache directly. Here are some advanced techniques:
1. Invaldiating Queries
Invalidating queries gives us precise control over when to refetch data.
queryClient.invalidateQueries('todos');
This command ensures fresh data is fetched immediately if the todos
key is referenced again.
2. Batching Updates
Instead of invalidating queries separately, batching updates helps in maintaining efficiency:
await queryClient.invalidateQueries(['todos', 'user']);
Batching allows you to invalidate multiple queries in a single call, reducing the number of re-renders and improving performance.
Customizing the Cache
Advanced caching strategies also involve customizing default behaviors for cache keys, garbage collection, and stale time.
Unique Query Keys
A common pitfall when working with React Query is not using unique cache keys. This can lead to data being overwritten unintentionally.
const { data } = useQuery(['todos', userId], fetchTodosByUser);
Using an array as a query key allows better cache management, especially when dealing with nested data.
Garbage Collection
React Query automatically garbage collects unused cache entries after they go stale. This process can be configured:
const queryClient = new QueryClient({
defaultOptions: {
queries: {
cacheTime: 1000 * 60 * 10, // 10 minutes
staleTime: 1000 * 60 * 2, // 2 minutes
},
},
});
This configuration specifies how long to keep the data in the cache before garbage collecting it, which can help in optimizing memory usage in larger applications.
Performance Testing with React Query
To ensure your strategies are having the desired effect, employing effective performance testing is crucial. Use tools like React Query Devtools to monitor the behavior of your queries and their states.
Measuring Cache Efficiency
React Query Devtools can help you visualize the cache status:
- Number of fetched entries
- Current states
- Re-fetching triggers
These metrics are essential for diagnosing performance bottlenecks in your application.
Conclusion
Caching strategies are foundational in building high-performance applications using React Query. By understanding how to leverage the power of caching effectively, applying advanced techniques like optimistic updates, prefetching, and batch updates, developers can substantially improve user experience and application performance. Don’t overlook the cache; it is a formidable asset in your development arsenal.
Throughout this post, we’ve explored how to implement and optimize caching mechanisms with React Query. With these practices in hand, you can ensure your applications remain responsive and efficient, even under heavy load.