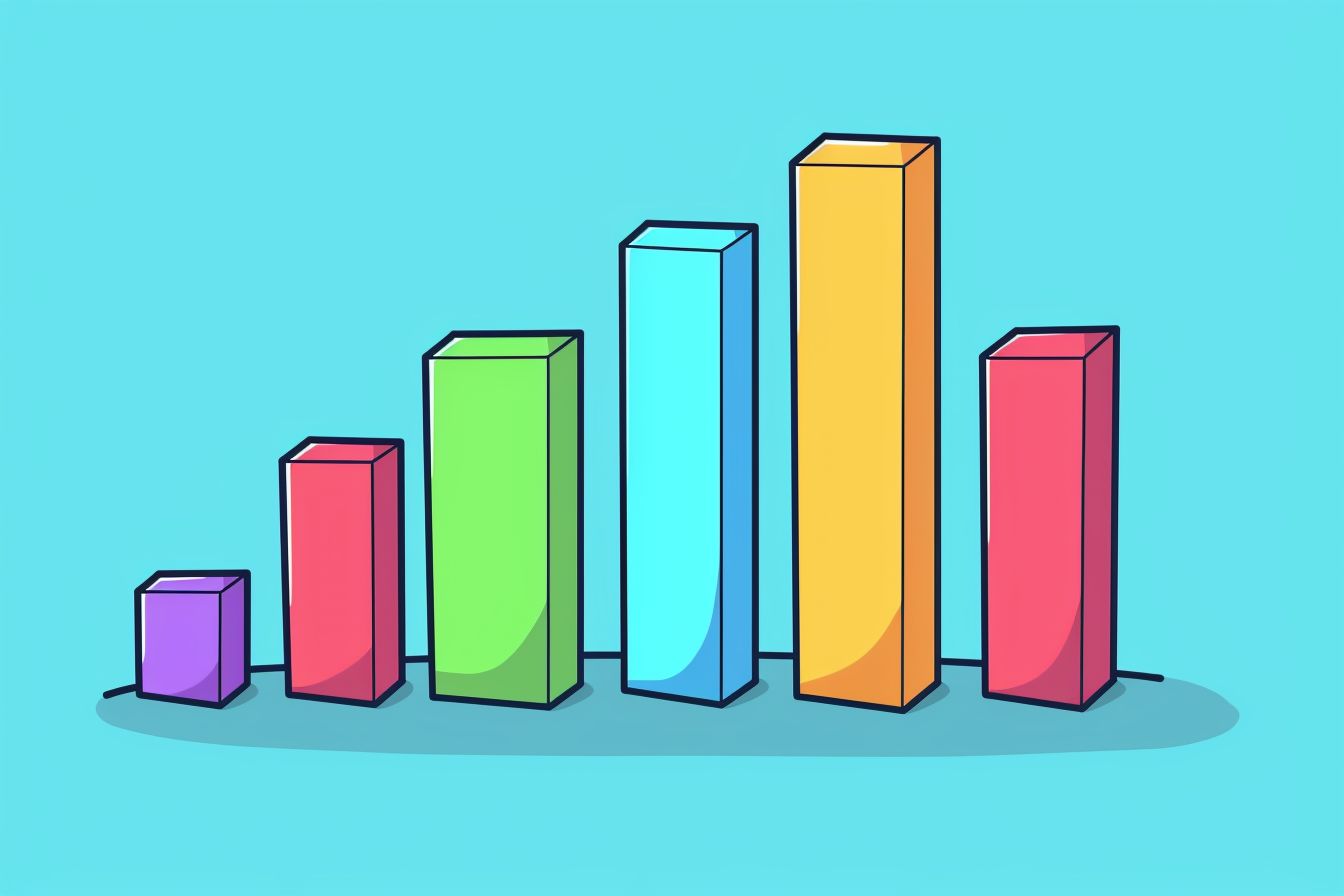
Customizing Data Presentation with React and D3.js
Unleashing the power of D3.js in React applications
Jul 29, 2024 - 16:09 • 4 min read
Data visualization is an essential aspect of modern web applications, and combining the strengths of React with D3.js can lead to stunning results. React handles the UI with its component-based architecture, while D3.js excels at manipulating documents based on data. The goal of this post is to show how to integrate D3.js into a React application effectively.
Setting Up the Project
To get started, we’ll create a React application using Create React App and install D3.js:
npx create-react-app react-d3-integration
cd react-d3-integration
npm install d3
Creating a Basic Chart Component
Let’s create a simple bar chart using D3.js. First, we need to import D3.js into our Chart component:
import React, { useEffect, useRef } from 'react';
import * as d3 from 'd3';
const BarChart = ({ data }) => {
const svgRef = useRef();
useEffect(() => {
const svg = d3.select(svgRef.current);
const width = 500;
const height = 500;
svg.attr('width', width).attr('height', height);
// Create scales
const xScale = d3.scaleBand()
.domain(data.map((d) => d.name))
.range([0, width])
.padding(0.1);
const yScale = d3.scaleLinear()
.domain([0, d3.max(data, (d) => d.value)])
.range([height, 0]);
// Create bars
svg.selectAll('rect')
.data(data)
.enter()
.append('rect')
.attr('x', (d) => xScale(d.name))
.attr('y', (d) => yScale(d.value))
.attr('width', xScale.bandwidth())
.attr('height', (d) => height - yScale(d.value))
.attr('fill', 'teal');
}, [data]);
return <svg ref={svgRef}></svg>;
};
Passing Data to the Chart Component
Now, let’s define some sample data and render the BarChart
component in our App:
const App = () => {
const data = [
{ name: 'A', value: 30 },
{ name: 'B', value: 80 },
{ name: 'C', value: 45 },
{ name: 'D', value: 60 },
{ name: 'E', value: 20 },
{ name: 'F', value: 90 },
{ name: 'G', value: 55 }
];
return (
<div>
<h1>Simple Bar Chart with D3.js</h1>
<BarChart data={data} />
</div>
);
};
export default App;
Now run your application using npm start
. You should see your first D3.js powered bar chart.
Handling Updates and Transitions
D3.js shines when it comes to dynamic updates and animations. Let’s integrate updates into the BarChart
functionality. To do that, we will modify the useEffect
hook to manage data changes:
Adding Click Interactions to Update Data
const App = () => {
const initialData = [
{ name: 'A', value: 30 },
{ name: 'B', value: 80 },
{ name: 'C', value: 45 },
{ name: 'D', value: 60 },
{ name: 'E', value: 20 }
];
const [data, setData] = useState(initialData);
const changeData = () => {
const randomData = initialData.map(d => ({...d, value: Math.floor(Math.random() * 100)}));
setData(randomData);
};
return (
<div>
<h1>Dynamic Bar Chart with D3.js</h1>
<BarChart data={data} />
<button onClick={changeData}>Change Data</button>
</div>
);
};
Applying D3.js Transitions
To make the chart visually appealing, we will add smooth animations. Modify the BarChart
component as follows:
useEffect(() => {
const svg = d3.select(svgRef.current);
const width = 500;
const height = 500;
svg.attr('width', width).attr('height', height);
// Create scales
const xScale = d3.scaleBand()
.domain(data.map((d) => d.name))
.range([0, width])
.padding(0.1);
const yScale = d3.scaleLinear()
.domain([0, d3.max(data, (d) => d.value)])
.range([height, 0]);
// Bind data
const bars = svg.selectAll('rect').data(data);
// Create new bars
bars.enter()
.append('rect')
.attr('x', (d) => xScale(d.name))
.attr('y', height)
.attr('width', xScale.bandwidth())
.attr('height', 0)
.attr('fill', 'teal')
.merge(bars)
.transition()
.duration(800)
.attr('y', (d) => yScale(d.value))
.attr('height', (d) => height - yScale(d.value));
// Remove old bars
bars.exit().remove();
}, [data]);
In this snippet, we use D3 transitions to modify the bars based on new data. Applying a transition makes the rendering smoother and visually consistent.
Conclusion
Combining React and D3.js allows developers to create dynamic, interactive data visualizations effortlessly. React takes care of the component structure, while D3.js handles the complex data manipulation and rendering efficiently.
In this post, we have explored the basic integration of D3.js with React, handled user interactions, and applied D3 transitions, giving us a solid foundation for developing advanced data visualization components.
Stay curious and keep experimenting with these tools to unlock new potentials in your web applications!