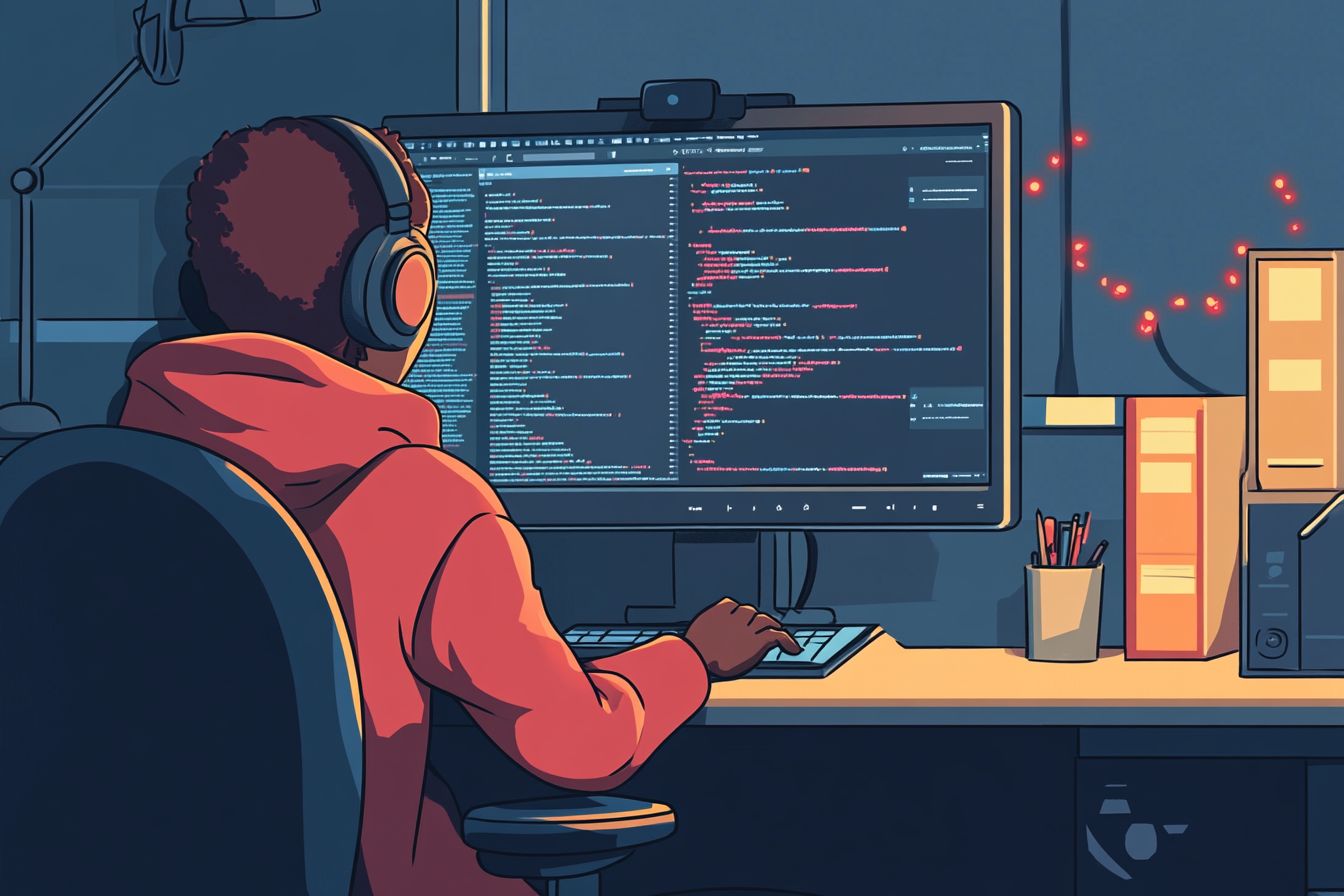
Optimizing API Calls in React with Custom Hooks
A deep dive into effective patterns for managing API interactions in React applications.
Aug 09, 2024 - 12:42 • 6 min read
Managing API calls in React applications can quickly become a complex endeavor, especially as the number and intricacy of requests grow. Developers often find themselves writing repetitive code, struggling with managing loading states, cache, and error handling, especially when using standard methods like fetch
or axios
. In this post, we'll explore advanced techniques for optimizing API requests in React using custom hooks, which can streamline code, promote reusability, and improve application performance.
Understanding the Need for Effective API Management
When building modern web applications, API calls are inherent to dynamic content delivery. For instance, imagine a dashboard that fetches user data, statistics, and live updates regularly. Without an efficient way to handle these API calls, our component can quickly turn into a waterfall of states and effects, which compromises both performance and maintainability.
The first key problem is redundancy. Without a dedicated workflow, developers may end up scattering the same API logic across multiple components, leading to difficulties in maintaining and updating the APIs in the future.
Additionally, there's the aspect of managing loading states and errors. Without clearly defined patterns, supporting user experiences during requests can often fall short, resulting in UI glitches or user frustration.
Introducing Custom Hooks for API Management
Custom hooks provide a powerful abstraction over traditional API calls, allowing developers to encapsulate logic into reusable functions that can easily manage fetching, caching, and error handling in one place. This allows components to focus solely on rendering and user interaction.
Let's take a look at a simple custom hook to fetch data from an API. Our goal is to create a reusable useFetch
hook:
import { useState, useEffect } from 'react';
function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
setLoading(true);
const response = await fetch(url);
if (!response.ok) throw new Error(`HTTP error! status: ${response.status}`);
const result = await response.json();
setData(result);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchData();
}, [url]);
return { data, loading, error };
}
In the useFetch
hook, we maintain states for data
, loading
, and error
. Then we use the useEffect
hook to manage the initial API call. This abstraction not only cleans up our component logic but also makes error handling and loading states much more manageable.
useFetch
Hook
Using the Here's how you would use the useFetch
hook in a component:
import React from 'react';
import useFetch from './useFetch';
function UserProfile() {
const { data, loading, error } = useFetch('https://api.example.com/user');
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return <div>{data.name}</div>;
}
By encapsulating functionality within a hook, we can beautifully keep our component minimal, focused, and easy to maintain.
Handling Caching and Performance
In addition to managing API calls, caching is crucial for optimizing performance levels. react-query
or swr
are popular libraries that can help manage caching and requests efficiently, but creating a custom caching system can also be advantageous in specific scenarios.
Imagine we want to cache responses in our hook. We can create a cache
object within our custom hook:
import { useState, useEffect } from 'react';
const cache = {};
function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
if (cache[url]) {
setData(cache[url]);
setLoading(false);
return;
}
const fetchData = async () => {
try {
setLoading(true);
const response = await fetch(url);
if (!response.ok) throw new Error(`HTTP error! status: ${response.status}`);
const result = await response.json();
cache[url] = result;
setData(result);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchData();
}, [url]);
return { data, loading, error };
}
In this setup, if a request has already been made for the given URL, the hook will return cached data instead of making a new request, thereby saving on unnecessary API calls and enhancing user experience.
Incorporating Querying and Pagination
Many applications require querying capabilities or pagination for their data. Extending useFetch
to handle parameters can make this straightforward. Here’s a slightly altered approach that incorporates parameters for fetching:
function useFetch(url, params = {}) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
setLoading(true);
const queryString = new URLSearchParams(params).toString();
const response = await fetch(`${url}?${queryString}`);
if (!response.ok) throw new Error(`HTTP error! status: ${response.status}`);
const result = await response.json();
setData(result);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchData();
}, [url, params]);
return { data, loading, error };
}
You can now pass search parameters or pagination details directly:
const { data, loading, error } = useFetch('https://api.example.com/users', { page: 2, limit: 20 });
This kind of flexibly will reinvent the way API data is handled inside your components.
Handling Concurrent Requests
In scenarios where multiple API calls may occur simultaneously, you can extend the logic further. Consider a case where a dashboard needs to fetch multiple sources of data, utilizing Promise.all
can simplify this:
async function fetchData(apis) {
const responses = await Promise.all(apis.map(api => fetch(api)));
const results = await Promise.all(responses.map(res => res.json()));
return results;
}
const useMultipleFetch = (urls) => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchAll = async () => {
try {
setLoading(true);
const results = await fetchData(urls);
setData(results);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchAll();
}, [urls]);
return { data, loading, error };
};
Conclusion
In wrapping up, designing custom hooks for managing API calls is not just a trend in React but is quickly establishing itself as a best practice. It not only results in cleaner, more maintainable code but also provides robust mechanisms for handling loading states, caching, and errors, thus improving user experience.
By progressively enhancing our hooks to include features like caching, querying, and concurrent requests, we're able to significantly amplify the efficiency of API management in our applications. Therefore, whether you’re building a simple application or a complex dashboard, investing in custom hooks for API management is worth every line of code. Go forth and create cleaner, more efficient, and intuitive React applications with API handling!