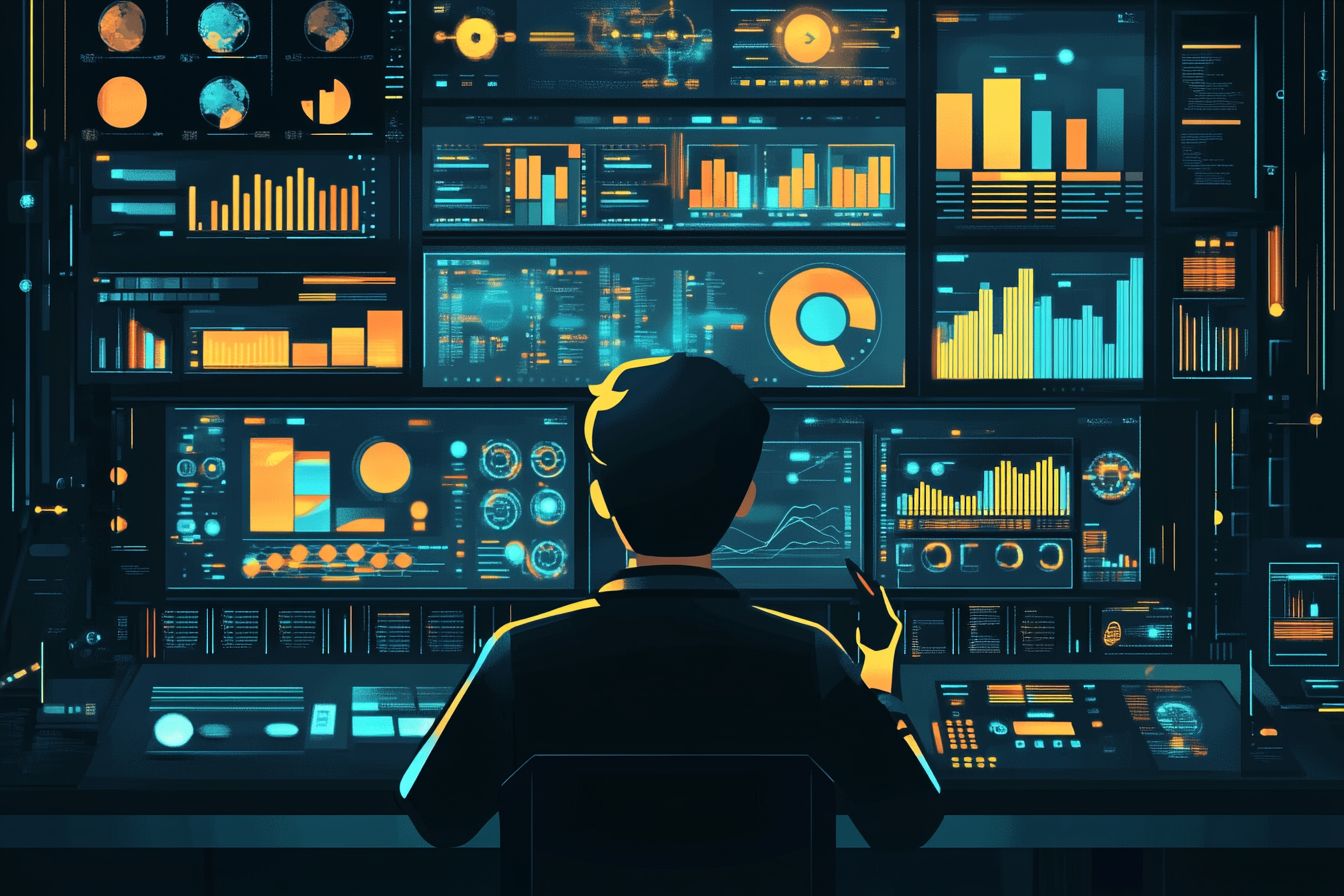
Understanding React Concurrent Features for Complex UIs
Dive deep into the power of React's concurrent features and how they can revolutionize performance in complex user interfaces.
Aug 21, 2024 - 22:40 • 4 min read
In recent years, the adoption of React has exploded, not only as a library for building UIs but as a framework capable of crafting complex, high-performance applications. A central part of this evolution is React's concurrent features, which aim to enhance the user experience by allowing UI updates to happen more fluidly—without blocking the main thread.
To fully grasp the potential of these features, let’s take a deeper dive into what concurrency in React means, the mechanics behind it, and the best practices to harness its power effectively.
What is Concurrent React?
Concurrent React introduces the concept of scheduling tasks to keep the main thread unblocked. The key aspects include suspense, transitions, and deferred updates. With these tools, React can prioritize rendering based on the user interaction, leading to smoother and more responsive applications.
The Basics of Scheduling
Before diving into specifics, let’s understand the scheduling mechanism. React separates tasks into different priority levels. For example, tasks triggered by user interactions can be processed with higher priority while background tasks are throttled. This allows React to remain responsive.
Here’s a brief example of how you might implement a component that utilizes transitions:
import React, { useState, startTransition } from 'react';
function MyComponent() {
const [items, setItems] = useState([]);
const addItems = (newItems) => {
startTransition(() => {
setItems([...items, ...newItems]);
});
};
return (<button onClick={() => addItems(['item1', 'item2'])}>Add Items</button>);
}
In this example, when the button is clicked, the state is updated inside a transition. This means the UI can remain responsive while the item addition occurs without blocking the rendering of the rest of the component.
Leveraging Suspense
Suspense gives us a way to handle asynchronous code gracefully. When combined with concurrent features, it allows us to define loading boundaries in our application. You can use Suspense to wait for data before rendering components.
Consider fetching user data:
import React, { Suspense } from 'react';
const UserData = () => {
const user = fetchUserData(); // Assume this is a function that fetches data
return <div>{user.name}</div>;
};
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<UserData />
</Suspense>
);
}
In the above example, if the user data isn’t ready, the fallback loading indicator is displayed, keeping the UI fluid without a blocking experience.
Prioritizing Updates with Transitions
Another feature of concurrent rendering is the ability to prioritize updates. With the startTransition
API, you can distinguish between urgent and non-urgent updates, allowing for better performance and user experience.
Example: Prioritized Update
function SearchInput() {
const [query, setQuery] = useState('');
const handleChange = (e) => {
const value = e.target.value;
startTransition(() => {
setQuery(value);
});
};
return (<input type="text" value={query} onChange={handleChange} />);
}
In this search input example, typing in the input does not freeze the UI, as it allows for the urgent task of handling the input change while simultaneously rendering the results based on the search query.
Optimizing Performance with Concurrent Features
Utilizing React’s concurrent features effectively can lead to significant performance improvements, especially in large applications. Here are some best practices:
- Use Suspense for Data Fetching: Every time you load data, wrap it in a Suspense component to handle loading states gracefully.
- Prefer Transitions Over Immediate Updates: Utilize transitions for updates that are not time-sensitive. This keeps the important user interactions responsive.
- Use
React.lazy()
with Dynamic Imports: For larger applications, lazy-load components to improve performance, especially on initial load.
Here’s an example of dynamic imports with Suspense:
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading component...</div>}>
<LazyComponent />
</Suspense>
);
}
Conclusion
Understanding and applying React's concurrent features can give developers the tools they need to create responsive, high-performance UIs. As applications grow in complexity, utilizing these principles will be critical in maintaining user experience while handling large amounts of data and complex state management.
By leveraging Suspense, transitions, and effective scheduling, you can ensure that your applications are not only performant but also enjoyable to interact with. Employ these features wisely and embrace the future of React development.
Additional Resources
To deepen your understanding of concurrent rendering and its benefits:
By mastering these tools, you’ll be well-prepared to tackle the challenges of building high-performance applications in React. Remember, the key is to prioritize user experience and keep interactions smooth and responsive.