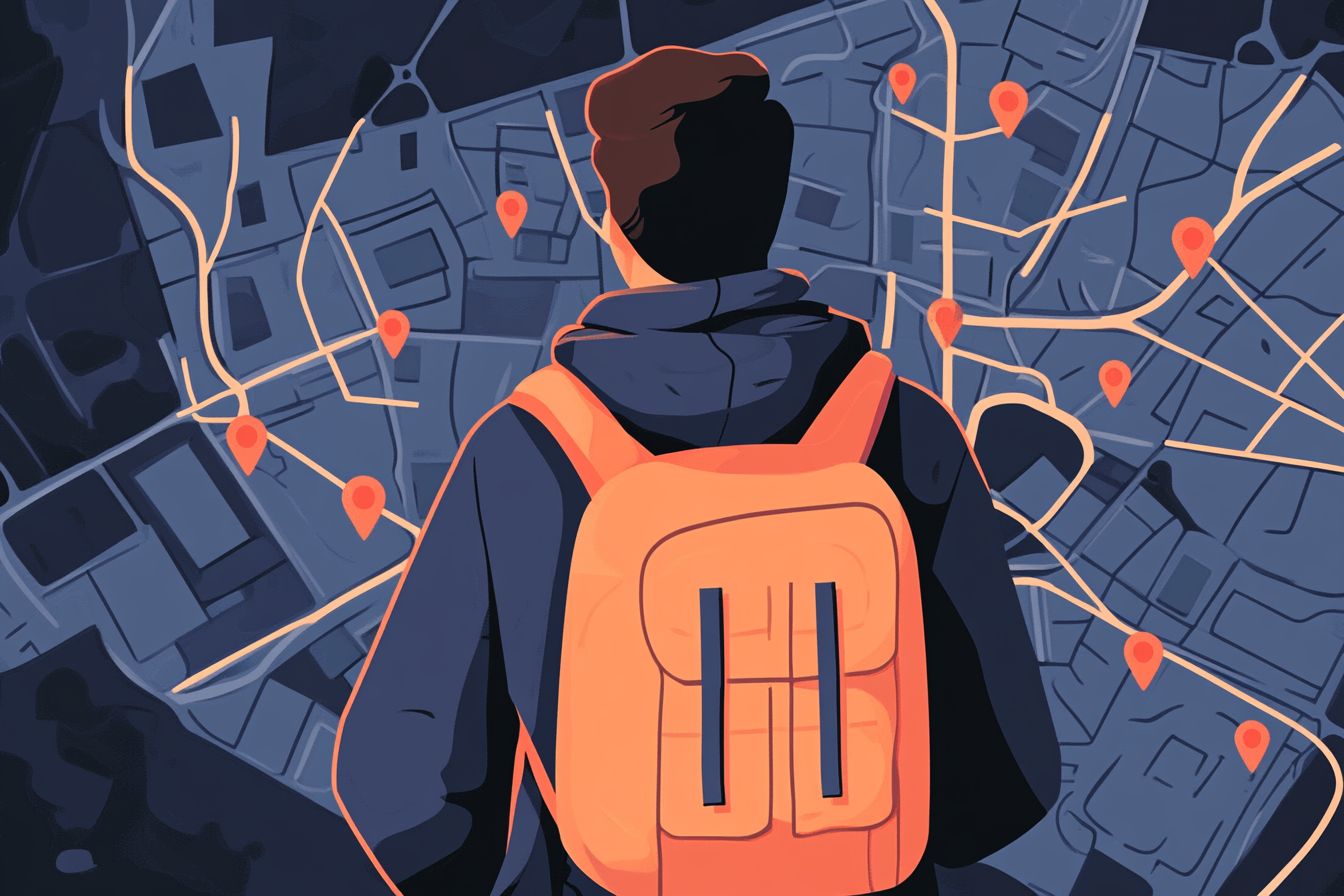
React Router v6: Advanced Routing Techniques
Mastering the intricacies of routing in React applications with version 6
Aug 20, 2024 - 12:56 • 5 min read
React Router has been a pivotal tool for building complex, client-side navigation in React applications. With the release of version 6, React Router introduced numerous enhancements and features designed to streamline routes and improve the developer experience.
In the following sections, we’ll delve deep into advanced routing techniques with React Router v6, focusing on nested routes, data loading strategies, route protection, and dynamic routing. Along the way, we’ll explore best practices, common pitfalls, and the latest trends in routing within modern React applications.
Understanding Nested Routes
One of the standout features of React Router v6 is its intuitive handling of nested routes. Nested routes allow you to render child components within parent routes, enabling a more organized and manageable code structure. For instance, when building an application with a sidebar, you might want the sidebar content to remain persistent while replacing the main content when navigating between routes.
Here’s an example of how to create nested routes:
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
function App() {
return (
<Router>
<Routes>
<Route path="/" element={<Layout />}>
<Route index element={<Home />} />
<Route path="about" element={<About />} />
<Route path="dashboard" element={<Dashboard />}>
<Route path="settings" element={<Settings />} />
<Route path="profile" element={<Profile />} />
</Route>
</Route>
</Routes>
</Router>
);
}
In this example, the Dashboard
component will serve as a parent route, while Settings
and Profile
act as its nested child routes. This allows you to maintain the state and layout of the dashboard, with its sub-components rendered according to the active route.
Rendering Nested Routes
To render nested routes, use the Outlet
component provided by React Router. This component acts as a placeholder, indicating where the child routes should render within the parent component.
import { Outlet } from 'react-router-dom';
function Dashboard() {
return (
<div>
<h2>Dashboard</h2>
<Outlet />
</div>
);
}
Data Loading Strategies
One of the challenges when dealing with routing is fetching data before rendering the component. React Router v6 simplifies this with the new loader
function. By defining a loader at the route level, you ensure that data is fetched before the component mounts, enhancing performance and user experience.
Here's how you can define a loader function that fetches data:
import { createBrowserRouter, RouterProvider } from 'react-router-dom';
const router = createBrowserRouter([
{
path: '/users',
element: <Users />,
loader: async () => {
const response = await fetch('https://api.example.com/users');
return response.json();
}
},
]);
function App() {
return <RouterProvider router={router} />;
}
The loaded data can be consumed within your component using the useLoaderData
hook:
import { useLoaderData } from 'react-router-dom';
function Users() {
const users = useLoaderData();
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
This approach ensures that your components are provided with the necessary data before rendering, preventing any content flicker or loading state in the UI.
useRoutes
Route Protection with In a production application, protecting specific routes is often required. React Router v6 introduces useRoutes
, allowing you to create a layout for protected routes swiftly. You can implement authentication checks and render accordingly.
Here’s an example of how to protect your routes:
import { useRoutes, Navigate } from 'react-router-dom';
function ProtectedRoute({ children }) {
const isAuthenticated = useAuth(); // Custom hook to check auth status
return isAuthenticated ? children : <Navigate to="/login" />;
}
function AppRoutes() {
const routes = useRoutes([
{
path: '/dashboard',
element: (
<ProtectedRoute>
<Dashboard />
</ProtectedRoute>
),
},
{ path: '/login', element: <Login /> },
]);
return routes;
}
Using ProtectedRoute
, you can safeguard components and redirect unauthorized users back to the login page. This modular approach keeps the authentication logic centralized and promotes easier maintenance.
Dynamic Routing: Handling Parameters
Dynamic routing enables the app to respond based on URL parameters. React Router v6 makes it easy to capture and use these parameters in your components. For instance, if you have a page that displays user profiles based on an ID, you can set up a parameterized route:
<Route path="users/:userId" element={<UserProfile />} />
In your UserProfile
component, you can access this parameter using the useParams
hook:
import { useParams } from 'react-router-dom';
function UserProfile() {
const { userId } = useParams();
// fetch and display user data based on userId
}
This makes your routing flexible and your components capable of rendering various content based on dynamic input.
Conclusion
React Router v6 has revolutionized how routing is implemented in React applications. By employing nested routes, data loaders, route protection, and dynamic routing, developers can build sophisticated applications that are both responsive and user-friendly.
Don't shy away from exploring these advanced routing techniques, as they can significantly enhance the architecture of your React applications, making them more efficient and manageable. As the ecosystem grows, staying updated with the latest features will equip you to craft even better user experiences.