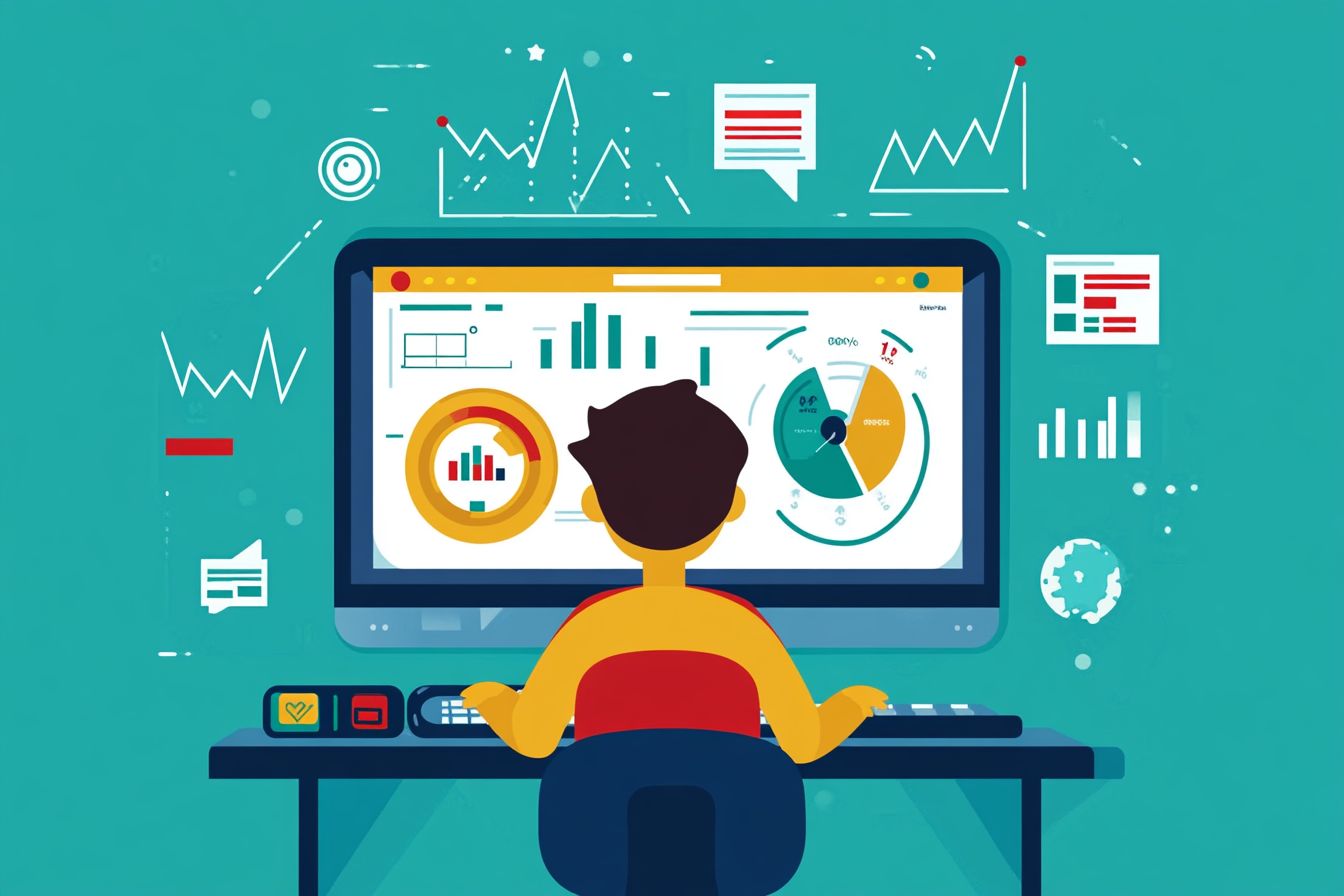
Efficient Memoization Patterns in React
Mastering the art of performance optimization with advanced memoization techniques.
Aug 21, 2024 - 22:49 • 5 min read
When working with React, performance is often a top priority, especially in complex applications. An important optimization technique is memoization, which allows components to avoid unnecessary re-renders. This post explores advanced memoization patterns and best practices in React, leveraging hooks and libraries that can make your applications faster and more efficient.
Understanding Memoization
Memoization is a technique that caches the results of function calls, avoiding the need to recompute results for previously encountered inputs. In the React context, this often means that we want to avoid unnecessary renders of components when props or state haven’t changed.
Here’s the basic idea: when a component re-renders, React will call the render function again. But if the inputs haven’t changed, we can return the previous output immediately. This reduces the amount of work React has to do, leading to performance gains.
React.memo
Basic Usage of Let’s start with the most straightforward form of memoization in React: React.memo()
. It is a higher-order component that memoizes rendering for functional components.
import React from 'react';
const MyComponent = React.memo(({ data }) => {
console.log('Rendering MyComponent');
return <div>{data}</div>;
});
In this example, MyComponent
will only re-render if the data
prop changes. You can provide a custom comparison function as the second argument to React.memo()
if you need more control over the comparison process.
Custom Comparison Function
A custom comparison function allows you to specify exactly how props should be compared. For instance, if your component receives an object as a prop, sometimes it is more beneficial to compare specific properties rather than the entire object.
const areEqual = (prevProps, nextProps) => {
return prevProps.data.value === nextProps.data.value;
};
const MyComponent = React.memo(({ data }) => {
console.log('Rendering MyComponent');
return <div>{data.value}</div>;
}, areEqual);
Here, MyComponent
will only re-render if the value
property within the data
object has changed, which is useful in preventing unnecessary updates when only other properties change.
Using useMemo and useCallback
While React.memo
is great for component memoization, we also have hooks like useMemo
and useCallback
to optimize the values/functions that a component uses.
useMemo
useMemo
is a hook that memoizes a calculated value to prevent recomputation on every render. It takes a function that calculates the value and an array of dependencies. If the dependencies haven’t changed since the last render, React will return the cached value.
const expensiveCalculation = (num) => {
// Complex calculation
return num * 2;
};
const MyComponent = ({ num }) => {
const memoizedValue = useMemo(() => expensiveCalculation(num), [num]);
return <div>{memoizedValue}</div>;
};
In this example, expensiveCalculation
will only rerun if num
changes, which can significantly improve performance when dealing with computationally intensive calculations.
useCallback
Similarly, useCallback
is used to memoize functions to avoid recreating them on every render, which can be particularly useful when passing callbacks to child components.
const MyComponent = ({ onChange }) => {
const handleClick = useCallback(() => {
onChange();
}, [onChange]);
return <button onClick={handleClick}>Click me</button>;
};
In this case, handleClick
will only be recreated when onChange
changes, allowing it to be passed without concern for unnecessary updates.
When to Use Memoization
Using memoization wisely is essential. Overusing it can lead to increased memory consumption and added complexity in debugging. Here are some guidelines:
- Component complexity: Only use memoization for components that are complex and likely to benefit from optimizations. Simple components may not need it.
- Performance testing: Measure performance before and after implementing memoization to ensure it provides a benefit.
- Avoid premature optimizations: Don’t introduce memoization too early in development; it complicates the code without a clear performance need.
Popular Libraries
For more complex scenarios, consider using libraries that facilitate memoization and caching. Some of the most popular include:
- Recoil: A state management library for React that supports derived state and inter-component dependencies, making memoization easier and more powerful.
- React Query: Handles caching and optimizing server-state management, which simplifies and speeds up data fetching in React applications.
- Lodash: Contains
memoize
and other utility functions that can be used for memoization tasks beyond React.
Examples of Memoization in Real Applications
Let’s discuss a case study where memoization can make a significant impact. Consider a data visualization dashboard where complex charts are updated in response to user input. If a chart component receives extensive data and recalculates the visualization on every render, the app may experience lag.
By memoizing the chart with React.memo
and using useMemo
to cache data transformations, we can ensure that the chart only updates when necessary. Here’s an example:
const Chart = React.memo(({ data }) => {
console.log('Rendering Chart');
// Render chart logic here
});
const Dashboard = ({ data }) => {
const processedData = useMemo(() => processData(data), [data]);
return <Chart data={processedData} />;
};
In this case, Chart
will only render when processedData
changes, significantly improving the performance of the dashboard.
Common Pitfalls
- Forgetting dependencies: When using
useMemo
anduseCallback
, ensure to include every external variable in the dependencies array to avoid stale data issues. - Unnecessary memoization: Applying memoization to trivial components may actually degrade performance instead of improving it. Always assess the trade-off.
Conclusion
Mastering memoization in React is an invaluable skill for developing high-performance applications. Using React.memo
, useMemo
, and useCallback
effectively can offload unnecessary computations and re-renders, making your user experience smoother. Explore the available libraries that complement React, harness the full potential of memoization, and always remember to test and measure the performance before and after implementing these optimizations. Your applications will not only run faster but will also be more responsive to user interactions.