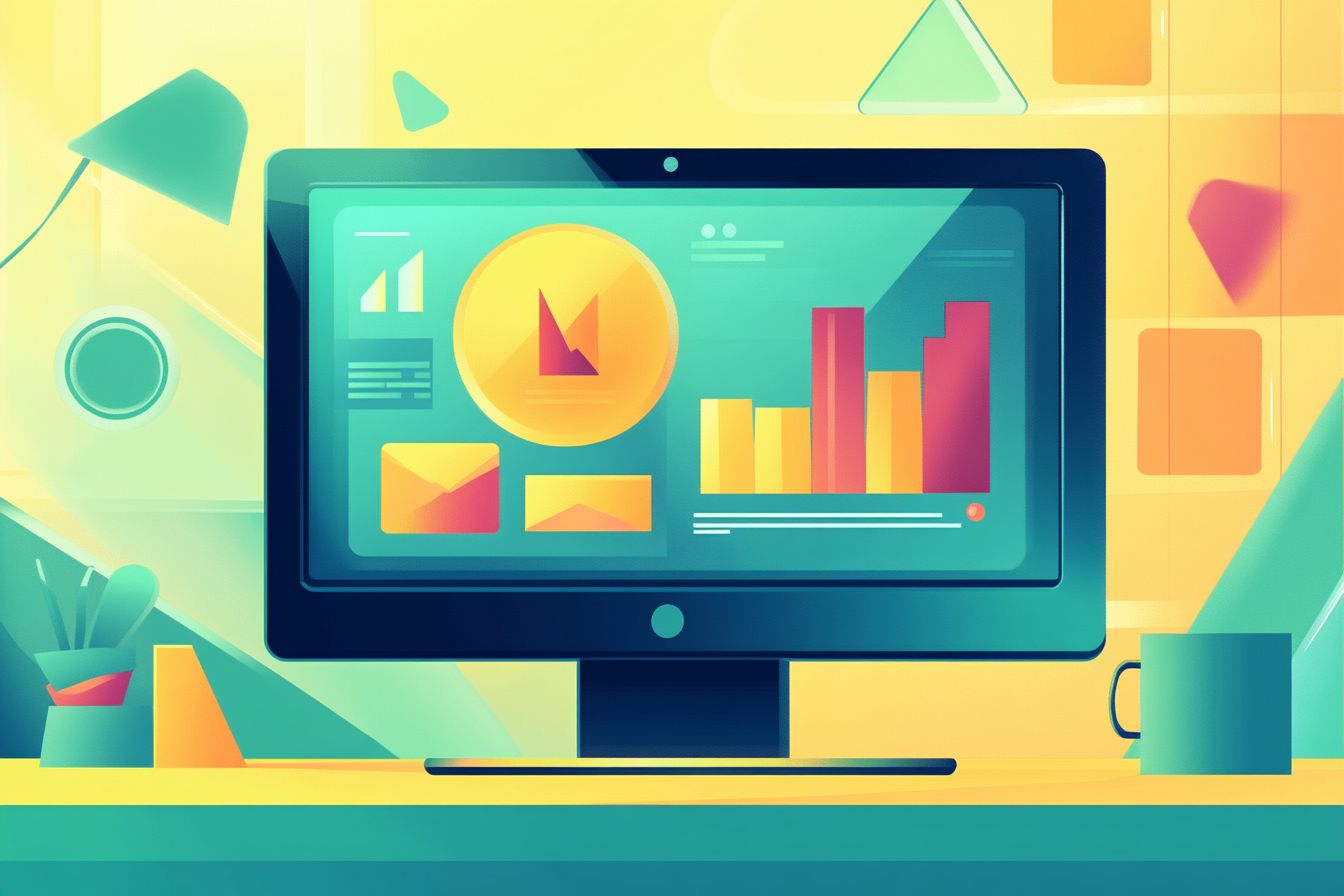
React Virtual DOM: Optimizing Updates for Enhanced Performance
Unlocking the Secrets of React's Rendering Mechanism
Aug 02, 2024 - 13:04 • 4 min read
As a web developer, understanding React's Virtual DOM and its optimization techniques is crucial to building high-performance applications. The Virtual DOM is a lightweight representation of the actual DOM, enabling React to minimize direct manipulations, which are notoriously slow. In this post, we will explore how the Virtual DOM works, examine common performance pitfalls, and uncover advanced optimization techniques.
Understanding the Virtual DOM
The Virtual DOM is a concept that allows React to optimize re-rendering processes. Rather than updating the actual browser DOM immediately when a component’s state changes, React first makes updates to a Virtual DOM. Here’s a brief overview of its workings:
- Rendering a Component: When a component's state or props change, React will render a new version of the component in the Virtual DOM.
- Diffing Algorithm: React employs a diffing algorithm that compares the new Virtual DOM with the previous Virtual DOM. It identifies what has changed between these two versions.
- Batching Updates: Instead of performing multiple updates to the browser’s DOM in sequence, React batches these updates, ensuring only necessary updates are applied.
- Updating the Real DOM: Finally, only the differences found in the diffing step are applied to the real DOM, leading to efficient updates.
Common Performance Pitfalls
While React does a fantastic job at optimizing updates, there are several common pitfalls that can hamper performance:
Excessive Component Renders: Components that render too often may negatively impact performance. If you’re using hooks like
useState
oruseEffect
, ensure they have the appropriate dependencies. Consistently usinguseEffect
without dependencies can lead to unwanted re-renders.useEffect(() => { // component logic }); // No dependencies will run on every render!
Large State Objects: When managing large state objects, be cautious. Updating any part of the state can lead to re-renders of all child components.
const [state, setState] = useState({ name: '', age: 0 }); setState({ ...state, age: state.age + 1 }); // Triggers a re-render for all children
Unoptimized Component Trees: Component trees with excessive depth or number can slow down rendering. Use React's
memo
orPureComponent
for functional components to prevent unnecessary re-renders.
Advanced Techniques for Performance Optimization
To enhance your application's performance with React, consider the following advanced techniques:
React.memo
and useMemo
1. Memoization with React.memo
: This higher-order component prevents functional components from re-rendering when their props have not changed.const MemoizedComponent = React.memo(({ data }) => { return <div>{data}</div>; });
useMemo
: Memoize expensive calculations inside functional components, so they only recompute when their dependencies change.const computedValue = useMemo(() => computeExpensiveValue(data), [data]);
2. Code Splitting
Utilize dynamic imports with React.lazy()
and Suspense
to split your code into smaller bundles. This allows React to load components only when they are needed, reducing initial load time.
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
3. Windowing and Virtualization
For applications that display large lists, like data tables or long lists, consider using libraries like react-window or react-virtualized. These libraries optimize rendering performance by rendering only the visible portion of the list.
import { FixedSizeList as List } from 'react-window';
<List
height={150}
itemCount={1000}
itemSize={35}
width={300}
>
{({ index, style }) => <div style={style}>Row {index}</div>}
</List>
useReducer
Hook
4. Leveraging the In complex applications, replace useState
with useReducer
to manage state logic more effectively. This will help in fine-tuning the updates that occur based on dispatched actions, keeping your components lean and minimizing unnecessary updates.
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
const [state, dispatch] = useReducer(reducer, initialState);
Conclusion
Understanding and optimizing the Virtual DOM is essential for building high-performance React applications. By grasping how the Virtual DOM operates, accounting for common performance pitfalls, and employing advanced techniques, you can significantly enhance your app's responsiveness and user experience. Always keep experimenting and profiling your application to find further improvements that suit your particular use case. React provides powerful tools to help us make the most of our applications, and optimizing how we handle updates can lead to smoother and faster UIs. Happy coding!