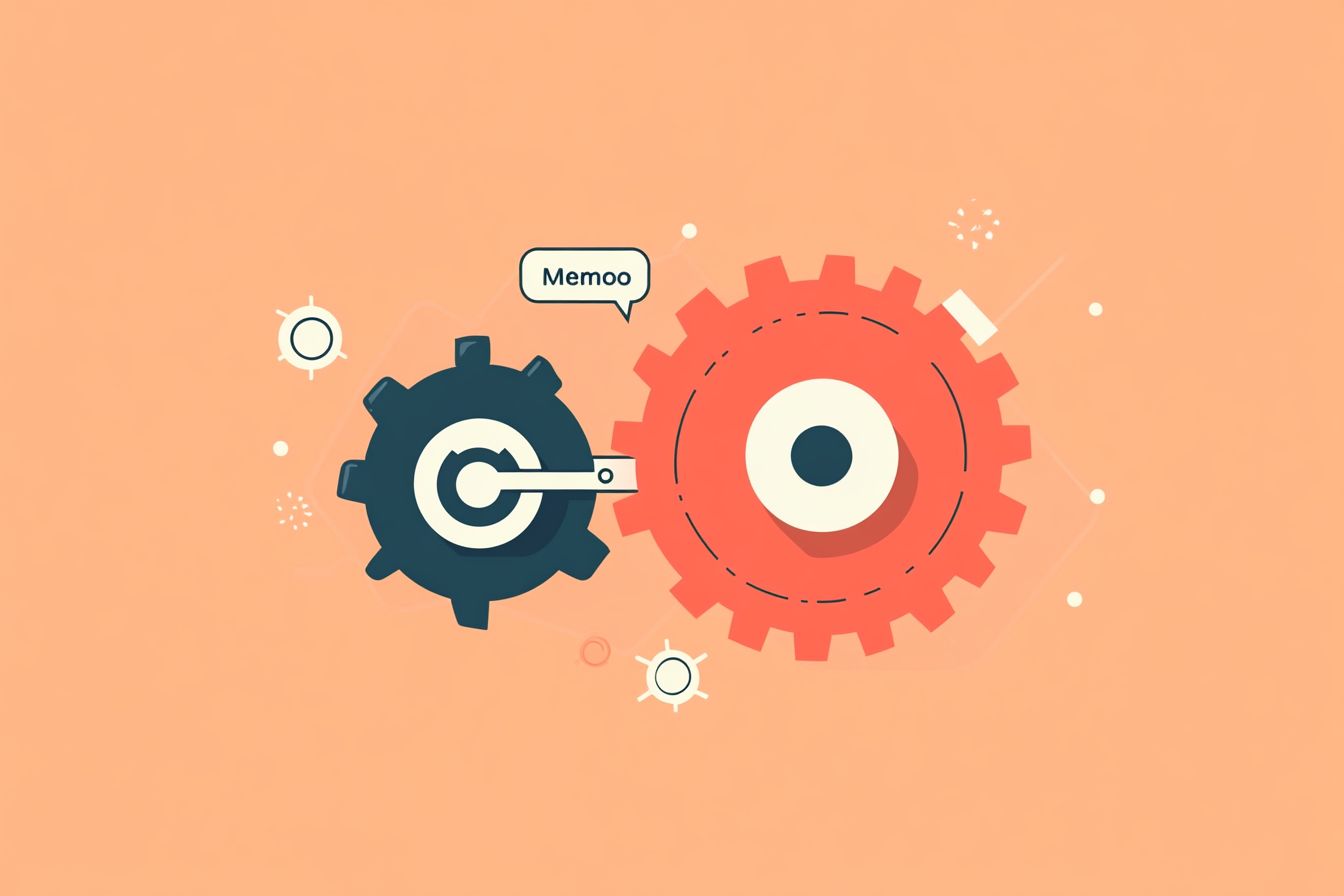
Optimizing React Re-renders with useMemo and useCallback
Master the subtleties of performance enhancements in React applications.
Aug 02, 2024 - 12:55 • 5 min read
In the world of React development, performance optimization is paramount. As applications grow in complexity, the methods we use to manage component re-renders become critical. The hooks useMemo
and useCallback
are handy tools that help us tackle the common pitfalls of unnecessary renders, but they are also often misunderstood or misused. This article aims to demystify these hooks, providing best practices and advanced techniques for harnessing their power, helping developers write efficient React apps.
Understanding the Basics: What are useMemo and useCallback?
Before diving into optimizations, let’s clarify the roles of useMemo
and useCallback
. Both hooks are designed to memoize values and functions, preventing unnecessary recalculations.
useMemo
: This hook returns a memoized value. It should be used when you want to optimize performance by avoiding expensive calculations on every render.useCallback
: This hook returns a memoized version of a callback function. It prevents the creation of a new function instance on every render, which can be particularly useful when passing callbacks to optimized child components.
Here's a simple example to illustrate how these hooks are used:
import React, { useState, useMemo, useCallback } from 'react';
function ExpensiveComponent({ compute }) {
return <div>{compute()}</div>;
}
function Parent() {
const [count, setCount] = useState(0);
const compute = useMemo(() => {
// Simulate an expensive calculation
let total = 0;
for (let i = 0; i < 10000000; i++) {
total += i;
}
return () => total;
}, []);
return (
<div>
<h1>{count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
<ExpensiveComponent compute={compute} />
</div>
);
}
In this example, useMemo
ensures that the computation is performed only once when the component mounts, avoiding recalculating the expensive operation on every render.
The Problem with Overusing useMemo and useCallback
While useMemo
and useCallback
can significantly improve performance, it’s crucial to avoid overusing them. Memorization itself has a performance cost; therefore, if used inappropriately, it can lead to worse performance than not using them at all.
For example, this code:
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
will only be beneficial if computeExpensiveValue
is genuinely expensive. Otherwise, the overhead of maintaining the memoized value can counteract its benefits.
Thus, best practice leans towards:
- Use
useMemo
for costly computations that are dependent on specific inputs. - Avoid applying
useMemo
to values that do not change frequently or might be cheaper to recalculate.
Optimizing Props with useCallback
Passing callbacks to child components can also lead to unnecessary re-renders. Using useCallback
can help if those child components are optimally designed to avoid re-renders (e.g., using React.memo
). Consider this example:
const handleClick = useCallback(() => {
setCount(prevCount => prevCount + 1);
}, []);
Best Practices for Using useMemo and useCallback
Be Specific with Dependencies: Always specify dependencies accurately. Ignoring dependencies may introduce bugs, whereas overly long dependency arrays can cause unnecessary re-renders.
const value = useMemo(() => expensiveFunction(a, b), [a, b]);
Empirical Testing: Performance optimizations should be empirically tested. Use React's built-in Profiler to analyze the performance impact of your implementations.
Use in Conjunction with Memoization: Combine
useMemo
anduseCallback
effectively, especially in larger applications with a prop drilling situation. Memoizing both the values and the functions can lead to reducing unnecessary renders and making components more efficient.Beware of Object and Array Dependencies: When using objects or arrays as dependencies, ensure to keep their references consistent, or use
useMemo
to create stable objects or arrays.Don’t Optimize Prematurely: Avoid the temptation to optimize every possible component right away. Focus on profiling your application and optimizing only what matters.
Advanced Techniques:
Dynamic Dependencies: For scenarios where dependencies can change, use a
ref
to keep track of the previous value and update accordingly:const previousValue = useRef(); const memoizedValue = useMemo(() => { previousValue.current = value; return computeNewValue(value, previousValue.current); }, [value]);
Custom Hooks for Reusable Logic: Build custom hooks encapsulating memoization logic across different components for improved code organization and DRY principles. This can also simplify the dependency management.
Here’s a generic example:
function useCachedFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const fetchData = useCallback(async () => {
const response = await fetch(url);
const result = await response.json();
setData(result);
setLoading(false);
}, [url]);
useEffect(() => {
fetchData();
}, [fetchData]);
return { data, loading };
}
Measuring Performance Improvements To measure the performance improvements from using useMemo
and useCallback
, you can use the React DevTools Profiler.
- Start profiling, perform actions to trigger rerenders, and then stop profiling.
- Analyze which components rendered, their durations, and how often they updated.
- Look for unnecessary renders caused by props changing between renders, and check if the memoization techniques helped reduce those renders.
Conclusion To summarize, useMemo
and useCallback
are powerful hooks that, if used wisely, can significantly enhance React application performance. Understanding when to utilize them, alongside their limitations, is crucial for creating applications that are not only functional but also performant. By adhering to best practices, you pave the way for future scalability and smoother user experiences. As you continue your journey in React, remember that performance optimizations should stem from real-world observations and measurable outcomes. Stay curious, keep profiling, and optimize intelligently!