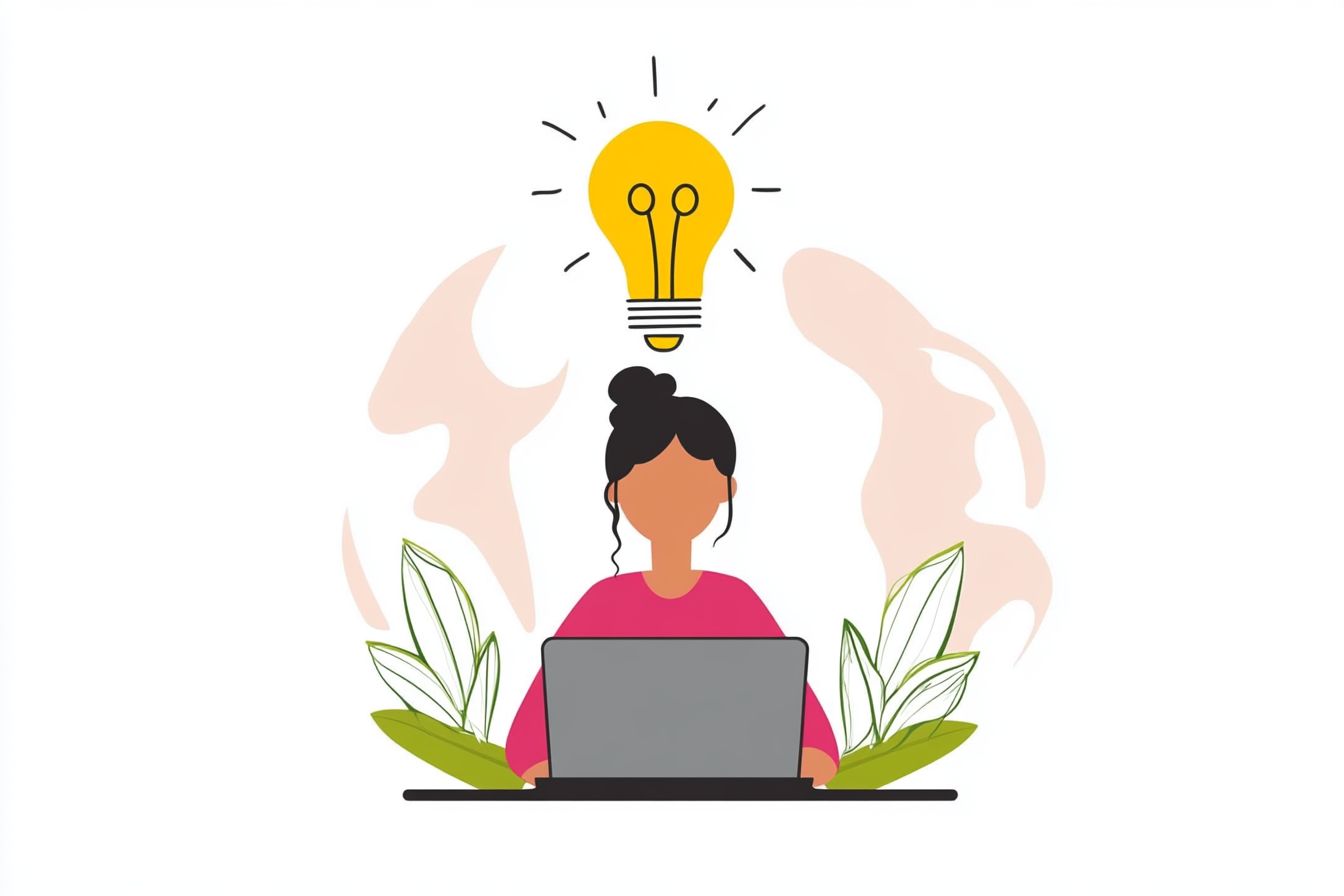
Understanding Render Prop Techniques in React
Elevate Your Component Architecture with Render Props
Aug 05, 2024 - 13:01 • 4 min read
Render Props is a powerful pattern for sharing code between React components using a prop whose value is a function. In this post, we will explore how to implement and utilize render props effectively, why they are beneficial for component composition, and some best practices to follow.
What Are Render Props?
At its core, the Render Prop pattern allows a component to act as a wrapper that controls its rendering using a function passed as a prop. This function returns JSX that is rendered by the child component, enabling different behaviors while keeping the logic encapsulated.
For example, let’s create a simple component using the Render Props pattern:
import React from 'react';
function DataFetcher({ render }) {
const [data, setData] = React.useState(null);
const [loading, setLoading] = React.useState(true);
React.useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
setData(data);
setLoading(false);
});
}, []);
return render({ data, loading });
}
In this example, DataFetcher
is a component that fetches data and provides it to its child through a render prop. The child component can then determine how to present the data:
function App() {
return (
<DataFetcher
render={({ data, loading }) => {
if (loading) return <div>Loading...</div>;
return <div>Fetched Data: {JSON.stringify(data)}</div>;
}}
/>
);
}
Here in App
, we pass a render function to DataFetcher
, specifying how we want to render the data depending on its loading state.
Why Use Render Props?
Code Reusability: Render props enable code reusability across components with different rendering logic. You can encapsulate shared logic within a render prop component and allow consumers to dictate their presentation.
Separation of Concerns: They allow separating the data-fetching logic from UI logic, improving maintainability.
Dynamic Rendering: As developers, we often need to modify how components render based on state or props, and render props provide an easy mechanism for this.
Steps to Implement Render Props
Identify the Common Logic: Determine which logic should be extracted to a shared component. For example, handling API calls or managing a component's visibility.
Create the Component: Define the component that will use a render prop. Make sure to expose necessary state and actions as part of the render prop's parameters.
Use the Render Prop: In the components that utilize the render prop, implement the desired rendering logic based on the props passed by the shared component.
Best Practices for Render Props
- Clear Naming: Use descriptive names for the render prop function to convey its purpose. For instance, instead of simply naming it
render
, consider something likechildren
orrenderItem
. - Documenting Props: Clearly document the props expected by the render function, including types and any dependencies.
- Use Memoization: If your render prop creates complex UI, consider using
React.memo
to prevent unnecessary re-renders. - Avoid Overusing: While render props are powerful, relying on them excessively can lead to complex usage patterns. Evaluate whether they are the most suitable pattern for your specific scenario or if alternatives like Higher-Order Components or custom hooks might be better suited.
An Example of Dynamic Behavior with Render Props
Let’s build on our previous example to create a more complex component that enables filtering of data based on user input:
function FilterableDataFetcher({ render }) {
const [filter, setFilter] = React.useState('');
const [data, setData] = React.useState([]);
React.useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
const filteredData = data.filter(item => item.includes(filter));
return (
<div>
<input
type="text"
value={filter}
onChange={e => setFilter(e.target.value)}
placeholder="Filter data..."
/>
{render(filteredData)}
</div>
);
}
In FilterableDataFetcher
, we added an input field that allows users to filter the data in real-time. The render
prop now receives the filtered data to display:
function App() {
return (
<FilterableDataFetcher
render={filteredData => (
<ul>
{filteredData.map(item => <li key={item}>{item}</li>)}
</ul>
)}
/>
);
}
Conclusion
The Render Props pattern is a powerful tool that enhances component composition in React. It allows developers to share logic without losing flexibility over component rendering. In this post, we explored the implementation of render props, their benefits, and best practices to keep in mind. By effectively leveraging this technique, you can elevate your component architecture and enhance code reusability within your React applications.