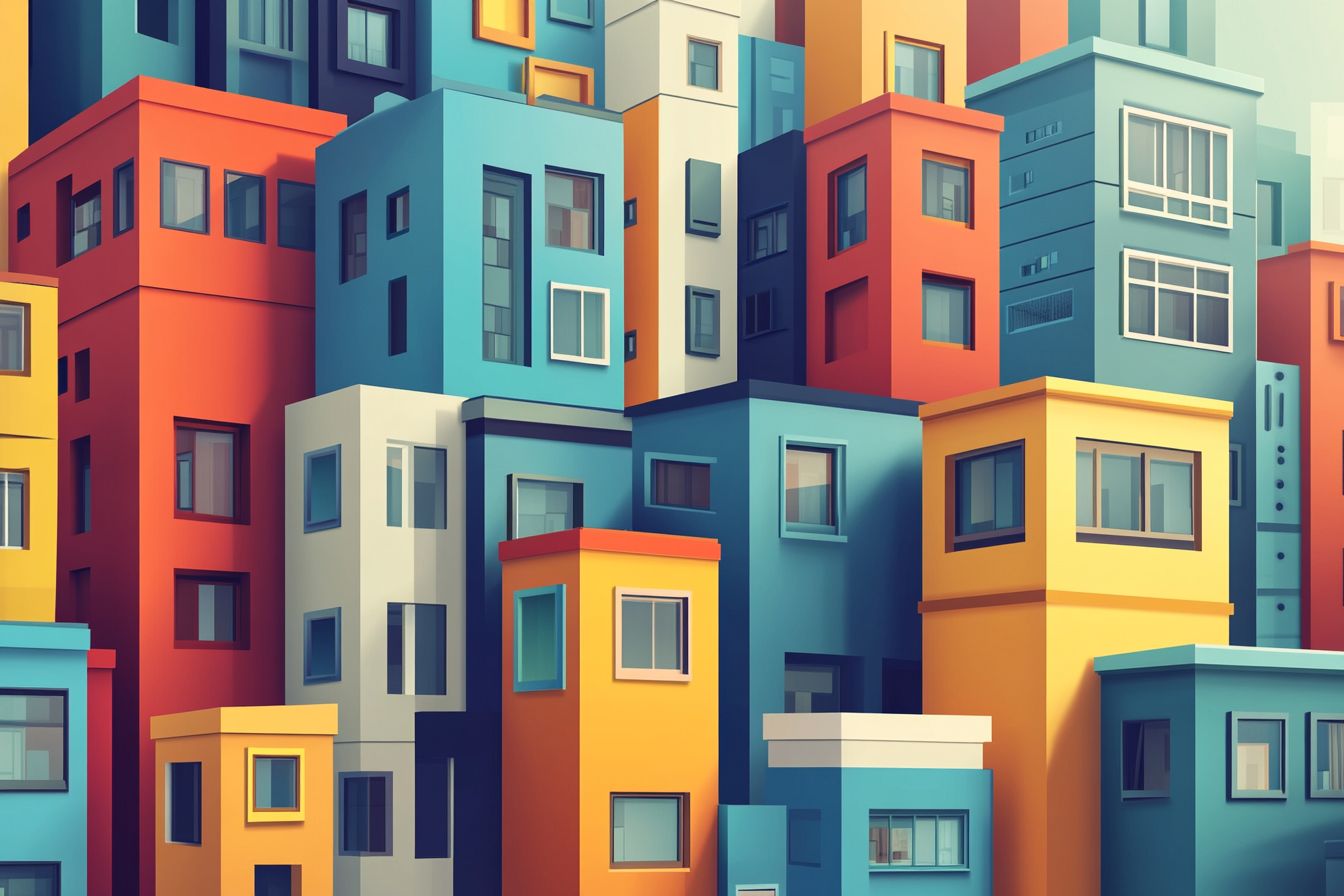
Dynamic Component Composition in React
Mastering Advanced Patterns to Build Flexible UI Systems
Aug 19, 2024 - 16:32 • 5 min read
With the rise of component-based design in React, developers are increasingly tasked with building dynamic interfaces that can adapt to user interactions and state changes. However, managing component composition effectively, especially in a complex application, can be a daunting challenge. In this post, we will explore advanced techniques for dynamic component composition in React. We will delve into strategies that can be employed to create flexible, reusable components and how to effectively manage their states and props.
Understanding Component Composition
Component composition is a powerful concept in React, enabling developers to create modular and reusable components through the composition of smaller components. In essence, component composition is about combining several components to build a more complex user interface. The React philosophy encourages developers to think in components, meaning we should view our UI as a tree of components.
Why Component Composition?
There are several reasons why component composition is beneficial:
- Reusability: Components can be reused in multiple places.
- Separation of Concerns: Smaller components manage their logic, styles, and markup.
- Readability: A well-structured component tree increases readability.
Dynamic Composition with Props
Dynamic composition can be achieved by passing props to components. Props are used to make components configurable, allowing for different data or behavior based on the input.
Consider a Button
component that changes its label based on the type
prop:
function Button({ label, onClick }) {
return <button onClick={onClick}>{label}</button>;
}
You could then compose this button with different labels:
<Button label="Submit" onClick={handleSubmit} />
<Button label="Cancel" onClick={handleCancel} />
But what if we want to compose multiple different buttons dynamically?
Dynamic Button Group
We can create a component called ButtonGroup
that accepts an array of button configurations and renders them accordingly:
function ButtonGroup({ buttons }) {
return (
<div>
{buttons.map((button, index) => (
<Button key={index} label={button.label} onClick={button.onClick} />
))}
</div>
);
}
This allows the ButtonGroup
to render any number of Button
components based on the supplied buttons
array, showcasing the power of dynamic composition.
Using Children as a Function
Another powerful technique is the Render Prop pattern. This involves passing a function as a prop to a component, allowing it to control what should be rendered. It can be particularly useful in complex scenarios.
function ComplexComponent({ render }) {
return (
<div>
<h1>Dynamic Content</h1>
{render()}
</div>
);
}
Then, when using this component, you can effectively create dynamic content depending on the current state of your application:
<ComplexComponent render={() => <p>This is some dynamic content!</p>} />
Controlled vs Uncontrolled Components
In dynamic component composition, it’s crucial to distinguish between controlled and uncontrolled components.
Controlled Components: These are components whose state is managed by React. They do not maintain their internal state but rather rely on props passed from their parent.
function ControlledInput({ value, onChange }) {
return <input type="text" value={value} onChange={onChange} />;
}
Uncontrolled Components: These components manage their state internally. They often make use of refs for controlling their behavior.
function UncontrolledInput() {
const inputRef = useRef();
const handleSubmit = () => {
alert(`Input Value: ${inputRef.current.value}`);
};
return (
<div>
<input type="text" ref={inputRef} />
<button onClick={handleSubmit}>Submit</button>
</div>
);
}
Best Practices for Component Composition
- Prefer Composition Over Inheritance: React encourages a compositional model rather than an inheritance-based model. By composing components, you gain better flexibility and reusability.
- Break Down Components: Don’t create large monolithic components. Break them down into smaller, manageable pieces that handle specific functionality.
- Share State Wisely: Use context or state management libraries like Redux for state that needs to be shared between deeply nested components.
- Prop Drilling: Be aware of props drilling and use context or libraries such as
react-query
to manage data and state shared across your components.
Result-Driven Component Structures with Higher Order Components (HOCs)
Higher Order Components are another powerful pattern that can allow dynamic behavior to be added to components. A Higher Order Component is a function that takes a component and returns a new component with additional props or behavior.
For example, a HOC that adds loading functionality might look something like this:
function withLoading(Component) {
return function WithLoadingComponent({ isLoading, ...props }) {
return isLoading ? <span>Loading...</span> : <Component {...props} />;
};
}
This can be used to wrap any component you want, providing additional loading state management:
const EnhancedComponent = withLoading(MyComponent);
Useful Libraries and Tools
Several libraries can help with component composition in React:
- React Query: Great for data fetching and managing server state, which can be composed with components to manage their loading and error states.
- Styled Components: For styling your components dynamically based on props, facilitating a clean API for styling.
- Lodash: Provides utility functions that can simplify operations such as filtering, sorting, and transforming data before it’s passed as props to components.
Conclusion
Building dynamic component systems in React is a critical skill for modern web developers. By utilizing the techniques discussed — props for component configuration, Render Props, Controlled vs Uncontrolled patterns, HOCs, and maintaining clean compositional structures — we can create flexible, reusable components capable of adapting to various application states. The best part? With each technique, you not only improve the architecture of your code but also enhance the maintainability and scalability of your applications. Coupled with the right tools and libraries, your ability to dynamically compose components will elevate your React applications to the next level.