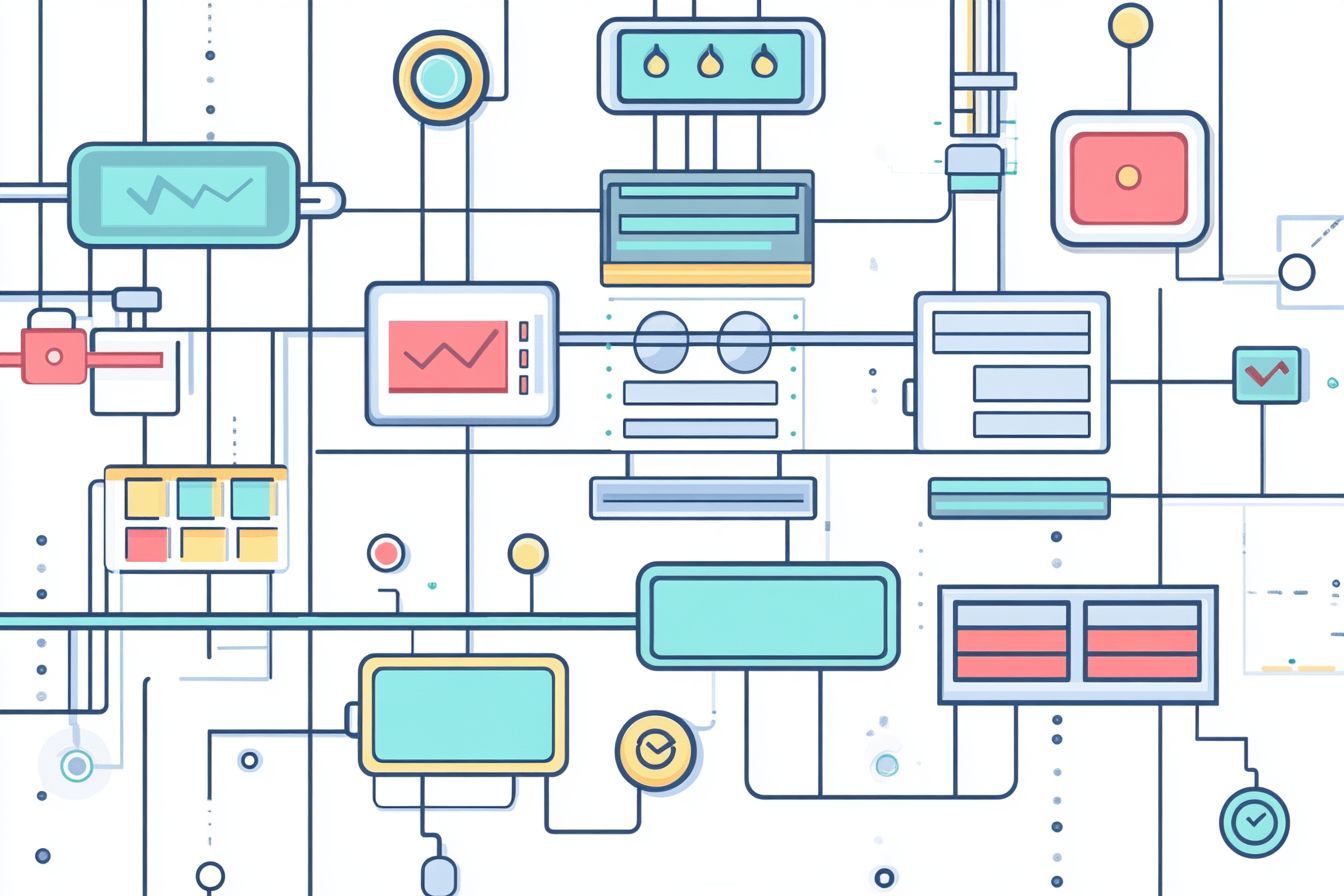
Creating Dynamic Component Systems with React and Custom Hooks
Utilize Custom Hooks for Enhanced React Component Reusability
Aug 02, 2024 - 12:46 • 5 min read
Creating Dynamic Component Systems with React and Custom Hooks
In the evolving world of modern web development, ensuring your components remain reusable, flexible, and maintainable is essential. As a developer, your goal often revolves around minimizing duplication and maximizing efficiency. When using React, one powerful way to achieve this is by leveraging custom hooks to create a dynamic component system, embracing both composition and encapsulation. This blog post dives deep into creating your own dynamic component systems using React and how custom hooks can help streamline this process.
Understanding Dynamic Components
Dynamic components are components that can change their appearance or behavior based on external conditions, such as state, props, context, etc. This allows developers to create highly reusable and adaptable UI elements without hardcoding multiple variations of the same logic. Dynamic components can reduce code duplication and improve the overall architecture of your application.
Why Use Custom Hooks?
Custom hooks provide a way to extract logic from components into reusable functions, promoting better organization and separation of concerns. They enable you to share stateful logic across components without changing the component hierarchy. For instance, you can build a custom hook that handles data fetching and manipulate UI based on the data's state.
Setting Up a Dynamic Component System
Let’s create a dynamic component system that renders different types of input fields based on user-selected criteria. This example demonstrates the power of custom hooks, explaining how we can structure our application.
Step 1: Base Logic with Custom Hook
We’ll start with a custom hook that determines the types of fields to render. This hook can contain the logic necessary to decide on the type and configuration of the input field.
import { useState } from 'react';
function useDynamicFields() {
const [fields, setFields] = useState([]);
const addField = (fieldType, fieldProps) => {
setFields(prevFields => [...prevFields, { type: fieldType, props: fieldProps }]);
};
return { fields, addField };
}
The useDynamicFields
hook initializes an empty fields array in its state and provides an addField
function that developers can use to add new fields dynamically. Every field can describe its type and any specific properties it may need.
Step 2: Creating Dynamic Field Components
Next, you need to implement the dynamic field renderer. Create individual components for the input types.
const TextField = ({ label, value, onChange }) => (
<div>
<label>{label}</label>
<input type="text" value={value} onChange={onChange} />
</div>
);
const NumberField = ({ label, value, onChange }) => (
<div>
<label>{label}</label>
<input type="number" value={value} onChange={onChange} />
</div>
);
const CheckboxField = ({ label, checked, onChange }) => (
<div>
<label>
<input type="checkbox" checked={checked} onChange={onChange} />
{label}
</label>
</div>
);
Step 3: Render Logic based on Type
Now, create an InputRenderer
component that utilizes the custom hook and renders appropriate field components based on the type defined in fields
.
import React from 'react';
import { useDynamicFields } from './useDynamicFields';
import TextField from './TextField';
import NumberField from './NumberField';
import CheckboxField from './CheckboxField';
const InputRenderer = () => {
const { fields } = useDynamicFields();
return (
<div>
{fields.map((field, index) => {
const { type, props } = field;
switch (type) {
case 'text':
return <TextField key={index} {...props} />;
case 'number':
return <NumberField key={index} {...props} />;
case 'checkbox':
return <CheckboxField key={index} {...props} />;
default:
return null;
}
})}
</div>
);
};
This renderer maps over each field and determines which component to render based on its type, applying the props defined by the custom hook.
Step 4: Integration: Adding Fields Dynamically
Let's connect our logic. You can create a Form
component that can add fields dynamically. Users can specify what type of field to add each time they submit.
const Form = () => {
const { addField } = useDynamicFields();
const handleAddField = () => {
const fieldType = document.querySelector('#fieldType').value;
const fieldLabel = document.querySelector('#fieldLabel').value;
addField(fieldType, { label: fieldLabel, value: '', onChange: (e) => { /* Handle change here */ }});
};
return (
<div>
<select id="fieldType">
<option value="text">Text</option>
<option value="number">Number</option>
<option value="checkbox">Checkbox</option>
</select>
<input id="fieldLabel" type="text" placeholder="Field Label" />
<button onClick={handleAddField}>Add Field</button>
<InputRenderer />
</div>
);
};
Best Practices for Dynamic Components
- Encapsulation: Create distinct components for different field types to manage their specific states and behaviors.
- Prop Customization: Utilize props effectively to allow parent components to dictate the rendering behavior and formatting.
- Optimizations: Consider using memoization for your dynamic fields if performance becomes an issue when dealing with many inputs.
- Typescript: If your app uses Typescript, consider typing your hooks and components to enhance code maintainability and reduce potential bugs.
Conclusion
Leveraging custom hooks in React development can transform how we approach dynamic components. By extracting complex logic into reusable hooks, we can keep our components cleaner, considerably easier to manage, and encourage proper separation of concerns. This pattern also not only enhances readability but contributes significantly to maintainability in larger applications.
Integrating dynamic capabilities into your components promotes a better user experience and aligns perfectly with modern development practices, following the trend towards functional programming paradigms. Keep exploring the vast capabilities of hooks to develop more organized and efficient React applications!