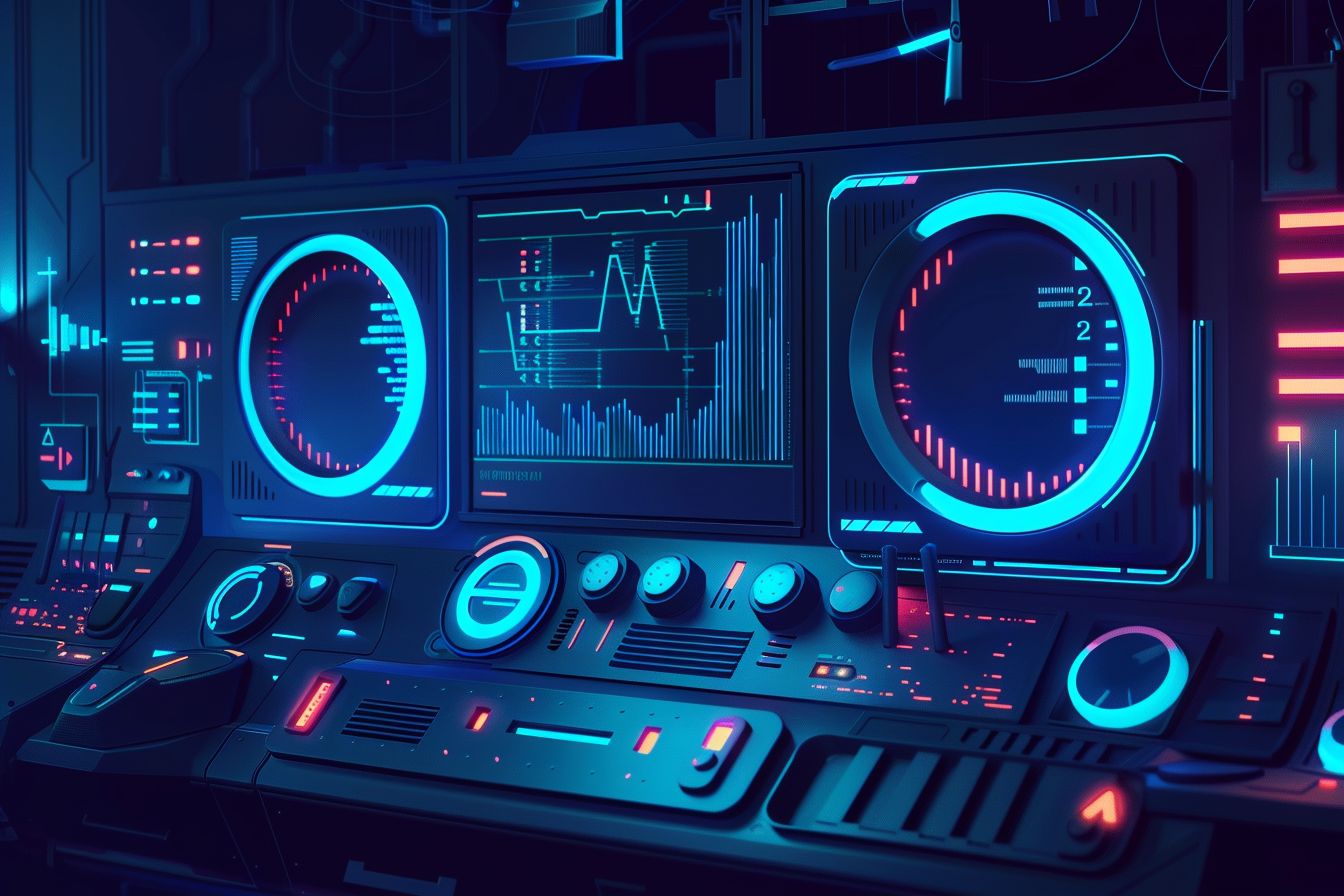
Optimizing React Applications with Memoization and UseMemo
Harnessing the Power of Memoization for Performance Gains in React Applications
Jun 27, 2024 - 11:06 • 3 min read
In the world of web development, performance optimization is a perpetual concern, especially as applications grow in complexity. One crucial tool in a React developer's arsenal is the concept of memoization. Memoization is a powerful technique that can significantly improve your application's performance by avoiding unnecessary calculations and rerenders.
Understanding Memoization
Memoization is an optimization technique used to improve the efficiency of functions by storing the results of expensive function calls and returning the cached result for the same inputs.
const memoize = (fn) => {
const cache = {};
return (...args) => {
const key = JSON.stringify(args);
if (cache[key]) {
return cache[key];
} else {
const result = fn(...args);
cache[key] = result;
return result;
}
};
};
In React, we leverage memoization through hooks like useMemo
, useCallback
, and the React.memo
higher-order component (HOC). These hooks and HOCs help us avoid unnecessary rerenders and recalculations, making our applications more efficient.
Using useMemo
The useMemo
hook allows you to memoize the result of a function. This means that the expensive computation only happens when its dependencies change.
Basic Usage
import React, { useMemo } from 'react';
const ExpensiveComponent = ({ num }) => {
const factorial = useMemo(() => {
const computeFactorial = (n) => (n <= 1 ? 1 : n * computeFactorial(n - 1));
return computeFactorial(num);
}, [num]);
return <div>Factorial of {num} is {factorial}</div>;
};
In this example, the factorial computation is only run when the num
prop changes.
Common Pitfalls
useMemo
should not be overused. Only use it for expensive computations. Memoization introduces its own performance costs, so it's crucial to measure and understand when it's beneficial.
Integrating Memoization with Hooks
useCallback
useCallback
is another hook that returns a memoized version of the callback function that only changes if one of the dependencies has changed.
import React, { useState, useCallback } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = useCallback(() => setCount(count + 1), [count]);
return <button onClick={increment}>Increment: {count}</button>;
};
React.memo
The React.memo
HOC memoizes functional components, avoiding unnecessary renders when props haven't changed.
const MyComponent = React.memo(({ value }) => {
console.log('Rendering MyComponent');
return <div>{value}</div>;
});
Real-world Example with Redux and React Query
Let's take a complex scenario combining Redux for state management and React Query for server state management.
Redux Connected Component
import React from 'react';
import { connect } from 'react-redux';
import { fetchData } from './actions';
const MyComponent = ({ data, fetchData }) => {
const handleFetch = useCallback(() => fetchData(), [fetchData]);
return <button onClick={handleFetch}>Fetch Data</button>;
};
const mapStateToProps = (state) => ({
data: state.data,
});
export default connect(mapStateToProps, { fetchData })(MyComponent);
React Query for Server State
import { useQuery } from 'react-query';
const fetchUser = async () => {
const response = await fetch('https://api.example.com/user');
return response.json();
};
const UserComponent = () => {
const { data, error, isLoading } = useQuery('user', fetchUser);
if (isLoading) return <div>Loading...</div>;
if (error) return <div>Error loading data</div>;
return <div>User: {data.name}</div>;
};
Conclusion
Memoization is a robust method for optimizing React applications, particularly when dealing with expensive computations and complex state management. However, it's essential to measure and understand when memoization is beneficial to avoid unnecessary overhead. By effectively integrating useMemo
, useCallback
, and React.memo
into your React applications, you can achieve significant performance improvements, creating a smoother and more efficient user experience.