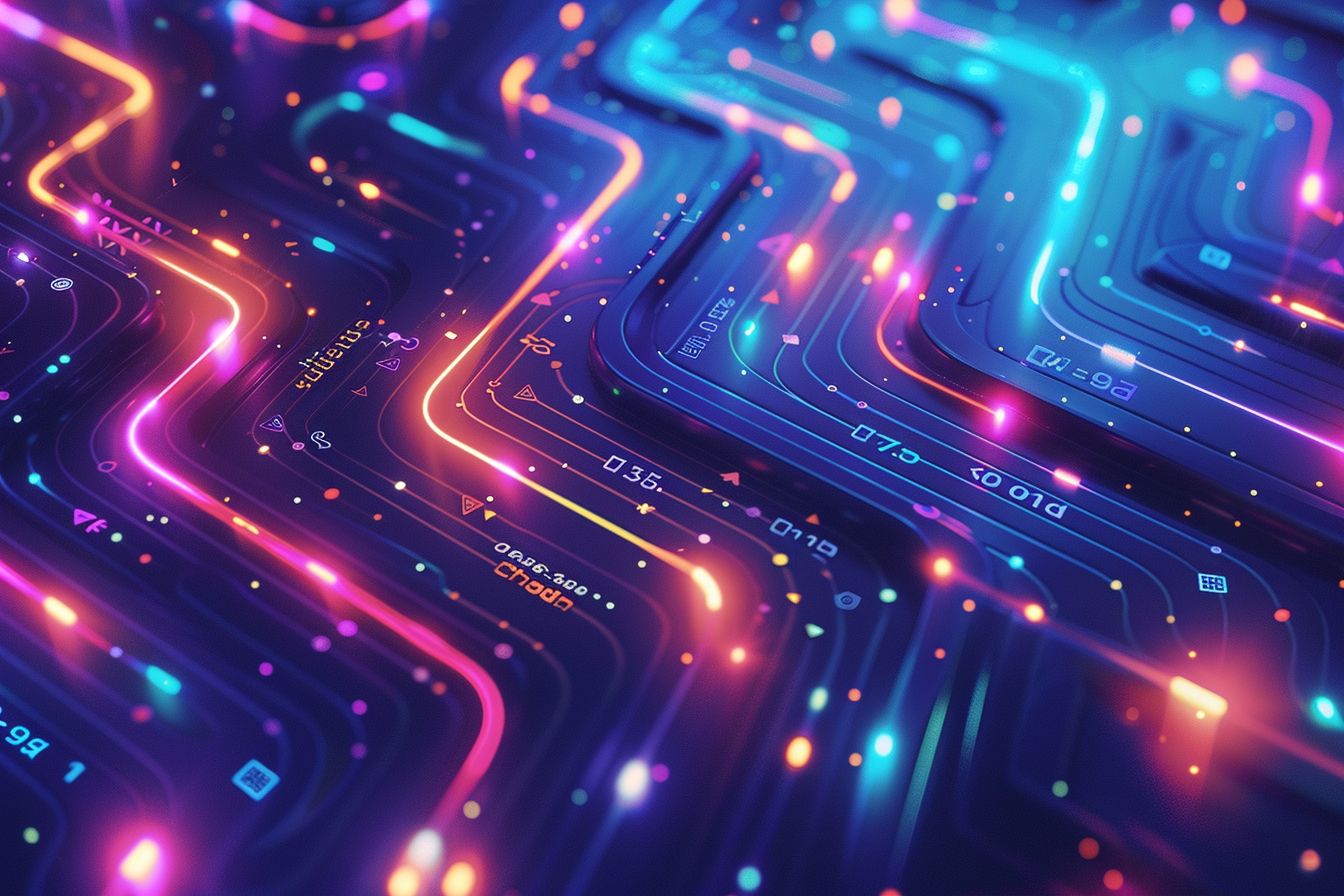
Deep Dive into React Suspense and Concurrent Features
Unleashing the Full Power of Asynchronous Rendering in React
Jun 26, 2024 - 23:23 • 6 min read
Deep Dive into React Suspense and Concurrent Features
React's asynchronous rendering capabilities have opened up new paradigms for building scalable, high-performance web applications. Among the most groundbreaking features are Suspense and Concurrent Mode. In this post, we will take a deep dive into how to effectively harness these features, ensuring that your applications remain snappy and user-friendly even under heavy load.
Why Suspense?
Suspense is designed to make handling asynchronous operations, like data fetching, easier and more declarative. Traditional asynchronous operations often involve setting state and handling various lifecycle methods, leading to complex and often convoluted code.
Consider this typical approach to fetching data in a React component:
import React, { useState, useEffect } from 'react';
function DataLoader() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
fetch('/api/data')
.then(response => response.json())
.then(setData)
.catch(setError)
.finally(() => setLoading(false));
}, []);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error!</p>;
return <pre>{JSON.stringify(data, null, 2)}</pre>;
}
This approach is effective, but it introduces a lot of state management and boilerplate code. Suspense aims to simplify this by consolidating the loading and error states into the component tree.
Introducing Suspense
Suspense allows you to declaratively specify a loading state for your components. Here is a simple example of how it works:
import React, { Suspense } from 'react';
const DataComponent = React.lazy(() => import('./DataComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<DataComponent />
</Suspense>
);
}
In this code, React.lazy()
is used to lazily load the DataComponent
when it is needed. The Suspense
component wraps the lazy-loaded component and specifies a fallback UI to be shown while it is being loaded.
Advanced Suspense Strategies
While the above examples are straightforward, real-world applications require more advanced strategies. For instance, managing multiple independent resources or coordinating asynchronous data fetching across various components.
Integration with React Query
React Query is a powerful library that makes fetching, caching, synchronizing, and updating server state in your React applications a breeze. It also plays very well with Suspense.
Let's integrate React Query with Suspense to manage data fetching more elegantly:
import React from 'react';
import { QueryClient, QueryClientProvider, useQuery } from 'react-query';
import { ReactQueryCacheProvider, ReactQuerySuspense } from '@tanstack/react-query';
const queryClient = new QueryClient();
function DataLoader() {
const { data } = useQuery('fetchData', () =>
fetch('/api/data').then(res => res.json())
);
return <pre>{JSON.stringify(data, null, 2)}</pre>;
}
function App() {
return (
<QueryClientProvider client={queryClient}>
<ReactQuerySuspense fallback={<div>Loading...</div>}>
<DataLoader />
</ReactQuerySuspense>
</QueryClientProvider>
);
}
In the above example, the ReactQuerySuspense
component handles the loading state for the DataLoader
component, encapsulating the logic within React Query’s ecosystem.
Concurrent Mode
Concurrent Mode is a set of new features that help React apps stay responsive and gracefully adjust to the user’s device capabilities and network speed. It makes React applications more fluid by introducing features like interruptible rendering and better scheduling.
Benefits of Concurrent Mode
- Automatic Batching: Updates inside concurrent mode are automatically batched, improving performance.
- Interruptible Rendering: React can interrupt rendering to stay responsive to user input.
- Deferred Value: Allows you to defer updates, making user interactions smoother.
Enabling Concurrent Mode
To start using Concurrent Mode, you need to use the concurrent root API introduced in React 18:
import { createRoot } from 'react-dom/client';
import App from './App';
const root = createRoot(document.getElementById('root'));
root.render(<App />);
This API changes how the root component is rendered, enabling Concurrent Mode features.
Practical Example: Data Fetching with Suspense and Concurrent Mode
Let's put it all together with a practical example.
Data Fetching with Suspense
import React, { Suspense, useState } from 'react';
import { createRoot } from 'react-dom/client';
import { QueryClient, QueryClientProvider, useQuery } from 'react-query';
import { ReactQuerySuspense } from '@tanstack/react-query';
const queryClient = new QueryClient();
function fetchData() {
return fetch('/api/data').then(res => res.json());
}
function DataLoader() {
const { data } = useQuery('data', fetchData);
return <pre>{JSON.stringify(data, null, 2)}</pre>;
}
function App() {
return (
<QueryClientProvider client={queryClient}>
<ReactQuerySuspense fallback={<div>Loading...</div>}>
<DataLoader />
</ReactQuerySuspense>
</QueryClientProvider>
);
}
const root = createRoot(document.getElementById('root'));
root.render(<App />);
In this example, ReactQuerySuspense
is used to encapsulate the loading state for the DataLoader
. This approach ensures that the UI remains responsive, leveraging both Suspense and Concurrent Mode.
SuspenseList for Coordinating Suspense Components
SuspenseList
allows you to coordinate the loading sequence of multiple components. It ensures a more cohesive user experience by orchestrating the display of several Suspense boundaries.
Example with SuspenseList
import React, { Suspense } from 'react';
function ProfileDetails() {
return <div>Loading Profile...</div>;
}
function CommentsSection() {
return <div>Loading Comments...</div>;
}
function App() {
return (
<SuspenseList revealOrder="together">
<Suspense fallback={<div>Loading Profile...</div>}>
<ProfileDetails />
</Suspense>
<Suspense fallback={<div>Loading Comments...</div>}>
<CommentsSection />
</Suspense>
</SuspenseList>
);
}
In this case, SuspenseList
ensures that both ProfileDetails
and CommentsSection
components are shown together once they are ready, enhancing the perceived performance of the application.
Error Handling in Suspense
Handling errors is crucial, especially in large applications. Suspense can be combined with error boundaries to handle errors gracefully.
Example with Error Boundaries
import React, { Suspense } from 'react';
import { QueryErrorResetBoundary, useQueryErrorResetBoundary } from 'react-query';
function ErrorFallback({ error, resetErrorBoundary }) {
return (
<div>
<p>Something went wrong: {error.message}</p>
<button onClick={resetErrorBoundary}>Try again</button>
</div>
);
}
function DataLoader() {
const { data } = useQuery('data', fetchData);
return <pre>{JSON.stringify(data, null, 2)}</pre>;
}
function App() {
const { reset } = useQueryErrorResetBoundary();
return (
<QueryErrorResetBoundary>
{({ reset }) => (
<ErrorBoundary
FallbackComponent={ErrorFallback}
onReset={reset}>
<Suspense fallback={<div>Loading...</div>}>
<DataLoader />
</Suspense>
</ErrorBoundary>
)}
</QueryErrorResetBoundary>
);
}
In this setup, the ErrorBoundary
component captures any errors that occur within the DataLoader
component, displaying a user-friendly error message and providing an option to retry.
Conclusion
React Suspense and Concurrent features represent a significant shift in how we think about and handle asynchronous operations and rendering in web applications. These tools enable more elegant and efficient handling of data fetching, loading states, error handling, and user interactions, resulting in a smoother and more responsive user experience.
The key is to start experimenting with these features in your projects. While the initial learning curve might seem steep, the long-term benefits in terms of code readability, maintainability, and performance are immense.
Happy coding!