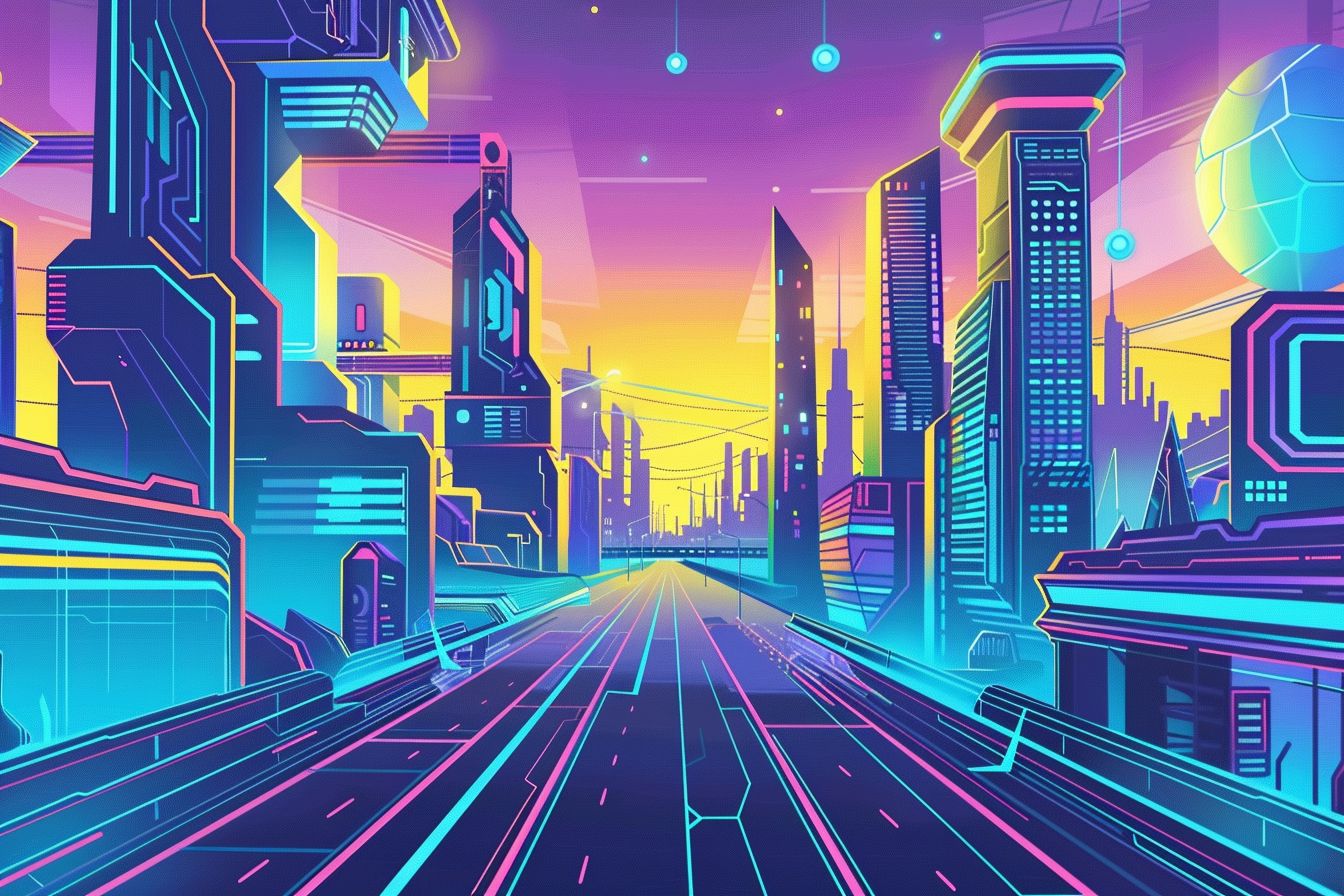
Mastering Advanced CSS Grid Techniques for Complex Layouts
Transform Your Designs with Next-Level CSS Grid Magic
Jun 28, 2024 - 00:08 • 3 min read
Introduction
CSS Grid has redefined how we approach web layouts, offering unprecedented control and flexibility. Yet, many developers only scratch the surface, missing out on advanced techniques that can make complex designs both simpler and more robust. This post dives deep into CSS Grid’s intricate elements, equipping you with the skills to create layouts that were once challenging to achieve.
The Power of Named Grid Lines
One often overlooked aspect of CSS Grid is its support for named grid lines. This feature allows you to create more readable and maintainable code.
Example
.grid-container {
display: grid;
grid-template-columns: [col-start] 1fr [col-middle] 2fr [col-end];
grid-template-rows: [row-start] auto [row-middle] auto [row-end];
}
With named grid lines, you can place items more explicitly:
.grid-item {
grid-column: col-start / col-middle;
grid-row: row-start / row-middle;
}
This clarity makes it easier to understand and update your layouts.
Implicit Grids
CSS Grid allows you to add items without explicitly defining all grid areas. This concept is known as implicit grids.
Example
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-auto-rows: minmax(100px, auto);
}
.grid-item-4 {
grid-column: 1 / span 2;
}
New items automatically create rows as needed:
<div class="grid-item">1</div>
<div class="grid-item">2</div>
<div class="grid-item">3</div>
<div class="grid-item grid-item-4">4</div>
<div class="grid-item">5</div>
This capability makes grids highly adaptable.
Grid Template Areas
Grid areas allow for a high-level representation of your layout. Instead of dealing with line numbers, you can name and refer to sections of the grid by name.
Example
.grid-container {
display: grid;
grid-template-areas:
"header header header"
"sidebar main main"
"footer footer footer";
}
.grid-header {
grid-area: header;
}
.grid-sidebar {
grid-area: sidebar;
}
.grid-main {
grid-area: main;
}
.grid-footer {
grid-area: footer;
}
This approach is remarkably intuitive, resembling wireframes.
Subgrid: The Game Changer
The subgrid
value for grid-template-columns
and grid-template-rows
properties enables a child grid to align with its parent grid's column or row structure. It’s indispensable for nested and more intricate designs.
Example
.parent-grid {
display: grid;
grid-template-columns: 1fr 2fr;
}
.child-grid {
display: grid;
grid-template-columns: subgrid;
}
Subgrid ensures the child elements align with the parent grid's columns perfectly, simplifying alignment.
Responsive Design with Grid
Responsive design is easily managed with CSS Grid. Combine grid-template areas and media queries to create adaptable layouts.
Example
@media (min-width: 800px) {
.grid-container {
grid-template-areas:
"header header header"
"sidebar main main"
"footer footer footer";
}
}
@media (max-width: 799px) {
.grid-container {
grid-template-areas:
"header header header"
"main main main"
"footer footer footer";
}
}
This simple adjustment makes your designs fluid across different screen sizes.
Conclusion
Mastering these advanced techniques allows you to unlock the full potential of CSS Grid, enabling you to create complex layouts with ease and precision. Whether it’s named grid lines, implicit grids, template areas, or leveraging subgrid, each tool offers unique benefits that can significantly enhance your web development workflow. Dive deeper into these features, and watch your designs transform!