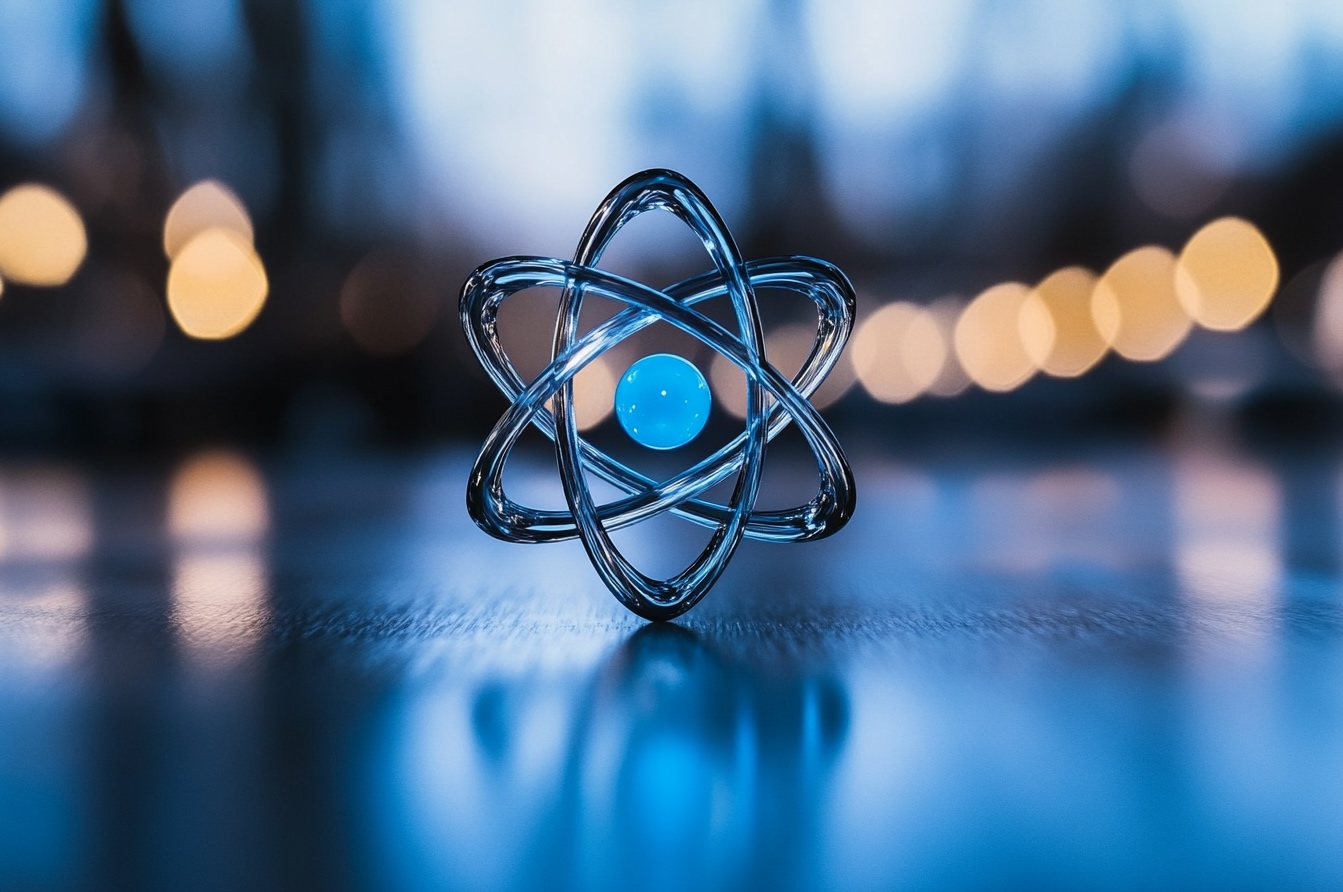
Mastering React's useCallback for Performance Optimization
Unlocking the secrets behind useCallback to reduce unnecessary renders and boost your application's performance.
Aug 09, 2024 - 13:04 • 5 min read
React is a powerful library for building user interfaces, and with it comes hooks that can simplify state management and lifecycle events. However, as React applications grow in complexity, developers are often faced with performance bottlenecks, particularly with unnecessary re-renders of components. One of the essential hooks designed to address this issue is useCallback
. In this post, we will explore the intricacies of useCallback
, how it operates under the hood, and best practices for leveraging this hook to optimize your React applications.
Understanding the Re-rendering Problem
Before we dive into useCallback
, it’s essential to grasp what causes components to re-render in React. A component will re-render if:
- Its state changes
- Its props change
- Force update methods are called (like calling
setState
)
When a component re-renders, React will also re-execute any functions provided as props or callbacks. This can lead to performance ramifications when you pass new function instances to child components on every render, prompting them to re-render even if their own props haven’t changed.
Consider the following scenario:
import React, { useState } from 'react';
function ChildComponent({ onButtonClick }) {
console.log('Child rendered');
return <button onClick={onButtonClick}>Click me!</button>;
}
function ParentComponent() {
const [count, setCount] = useState(0);
const handleClick = () => {
console.log('Button clicked');
};
return (
<div>
<ChildComponent onButtonClick={handleClick} />
<button onClick={() => setCount(count + 1)}>Increment Count</button>
<p>Count: {count}</p>
</div>
);
}
In this example, the ChildComponent
will re-render any time ParentComponent
is updated because handleClick
is recreated on every render. As your component hierarchy increases, this might lead to performance hits.
Enter useCallback
useCallback
is a hook that returns a memoized version of the callback function that only changes if one of the dependencies has changed. Here’s how we can apply it to our previous example:
import React, { useState, useCallback } from 'react';
function ChildComponent({ onButtonClick }) {
console.log('Child rendered');
return <button onClick={onButtonClick}>Click me!</button>;
}
function ParentComponent() {
const [count, setCount] = useState(0);
const handleClick = useCallback(() => {
console.log('Button clicked');
}, []);
return (
<div>
<ChildComponent onButtonClick={handleClick} />
<button onClick={() => setCount(count + 1)}>Increment Count</button>
<p>Count: {count}</p>
</div>
);
}
In this modification, handleClick
is wrapped in useCallback
. The function instance remains the same unless its dependencies change, which in this case, we specified an empty dependency array indicating that the function should not change.
Drilling Deeper: The Internal Mechanics of useCallback
Developers often overlook the internal mechanics of how useCallback
and useMemo
distinguish themselves in rendering cycles. The React hooks system keeps track of the dependencies and utilizes the last rendered component’s instance to dictate when to update the saved callback.
Here’s the basic architecture of how you'd think about a custom implementation of useCallback
:
import { useRef, useEffect } from 'react';
function useCustomCallback(fn, deps) {
const callbackRef = useRef();
useEffect(() => {
callbackRef.current = fn;
}, [fn]);
return (...args) => {
return callbackRef.current(...args);
};
}
The useRef
allows you to keep the most recent version of the function, allowing it to persist through renders without causing additional rerenders in components using it. Functions that depend on up-to-date state can also be correctly invoked each time.
Identifying Dependencies
One common pitfall while using useCallback
is neglecting to properly define dependencies. The dependencies array is crucial, as not specifying the right dependencies can lead to stale closures. For instance, if your callback accesses state or props directly, you need to include them in the dependencies.
const handleClick = useCallback(() => {
console.log(`Count is: ${count}`);
}, [count]);
This ensures that every time count
changes, a new instance of handleClick
is generated, thus eliminating stale closures yet still benefiting from the memoized optimization when count
remains the same.
Performance vs Readability Trade-off
To optimize performance, it's tempting to memoize everything using useCallback
, but this can lead to code that is harder to read and maintain. In practice, only memoize callbacks that are passed to optimized child components, as this prevents unnecessary renders and keeps your component performance intact.
For instance, using React.memo
on the child component ensures that it only renders when its props change:
const ChildComponent = React.memo(({ onButtonClick }) => {
console.log('Child rendered');
return <button onClick={onButtonClick}>Click me!</button>;
});
Combining useCallback with useMemo
Another advanced pattern is combining useCallback
with useMemo
. This approach is useful when you also want to memoize derived state based on props or state changes.
const computeHeavyResult = useMemo(() => computeExpensiveValue(count), [count]);
const handleClick = useCallback(() => {
console.log(computeHeavyResult);
}, [computeHeavyResult]);
In this example, computeHeavyResult
is recalculated only when count
changes, and handleClick
will have access to the latest result without additional re-renders.
Best Practices when using useCallback
- Use for Performance Gains: Only use
useCallback
when passing callbacks to memoized components to reduce renders. - Specify Dependencies Carefully: Missing dependencies can lead to stale closures, while over-specification may lead to unnecessary callback recreations.
- Combine with React.memo: Utilize
React.memo
in conjunction withuseCallback
to maximize performance benefits in child components. - Complex Scenarios: For complicated dependencies, consider extracting logic into separate hooks.
Conclusion
useCallback
offers a nuanced method of avoiding unnecessary renders in your React applications, significantly advancing performance in larger component trees. Understanding its behavior and proper applications is essential to sculpting efficient applications. Each application will come with its unique challenges, and leveraging these React features will provide the ability to craft seamless user experiences. With balanced use, useCallback
and its accompanying hooks can significantly enhance both user experience and application performance.