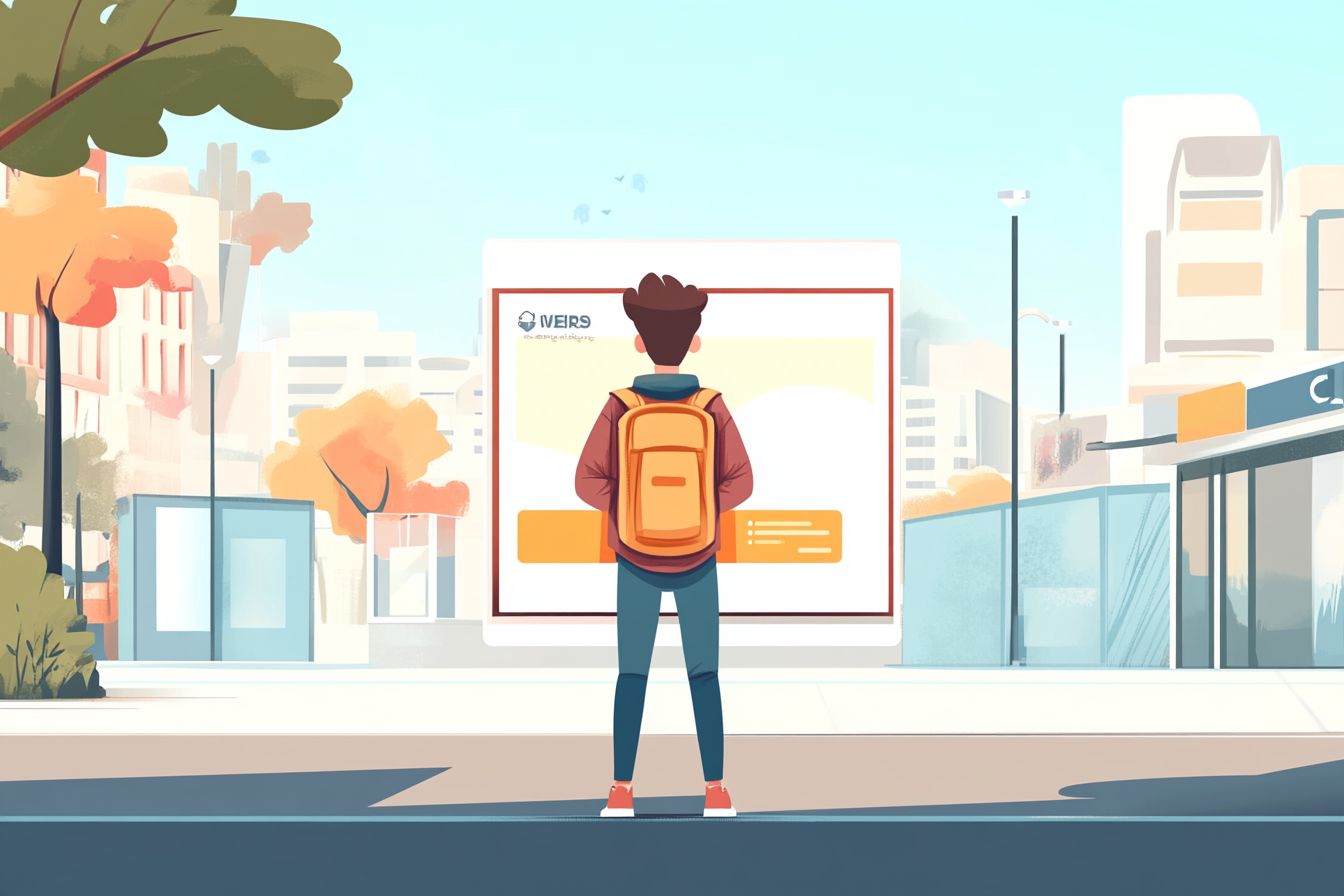
Advanced React Component Patterns with Render Props
Mastering the art of reusability in your React applications
Aug 09, 2024 - 13:06 • 5 min read
Over the last few years, as we’ve seen React gain traction within the web development ecosystem, we've also witnessed an evolution in how we architect our applications. While functional components and hooks have become the preferred way of writing modern React applications, the concept of render props has remained a powerful and flexible pattern that often goes underutilized.
In this post, we'll dive deep into render props, unravel the intricacies of how they can enhance reusability within your components, and explore best practices Tailored for both new and seasoned developers.
What Are Render Props?
The render prop pattern is a technique for sharing code between React components using a prop that is a function. This prop allows one component to control the rendering of another component, enabling a more flexible way to compose components without tightly coupling them together.
Here's a simple example to illustrate:
import React from 'react';
const DataProvider = ({ render }) => {
const data = [1, 2, 3]; // Imagine this data is fetched from an API
return <div>{render(data)}</div>;
};
const App = () => {
return (
<DataProvider render={(data) => (
<ul>{data.map(item => <li key={item}>{item}</li>)}</ul>
)}/>
);
};
export default App;
In this example, DataProvider
takes a render
prop and invokes it to render the data. This pattern makes the DataProvider
component reusable because it does not dictate how the data should be rendered; it hands control over to the render function provided by the parent component.
Building a Reusable Modal with Render Props
Let's take this understanding and build a more complex example. Suppose we want to create a modal component that can be reused in various parts of our application but with different content and behavior each time it is opened.
Firstly, we create a Modal
component:
import React, { useState } from 'react';
const Modal = ({ render }) => {
const [isOpen, setIsOpen] = useState(false);
const toggleModal = () => setIsOpen(!isOpen);
return (
<div>
<button onClick={toggleModal}>{isOpen ? 'Close' : 'Open'} Modal</button>
{isOpen && (
<div className="modal">
<div className="modal-content">
<button onClick={toggleModal}>Close</button>
{render()}
</div>
</div>
)}
</div>
);
};
export default Modal;
In this example, the Modal
component uses a render prop to allow consumers to dictate what should happen within the modal's body. Now, when utilizing this modal component in our app, it can accept any content:
const App = () => {
return (
<Modal render={() => <h1>Hello, World!</h1>} />
);
};
Avoiding Prop Drilling
One of the most compelling reasons to leverage render props is to avoid prop drilling—passing data through many layers of components. When you've got a component nested deep within your tree, it can become cumbersome to pass props down through every intermediary. This is especially true in larger applications.
Consider this structure:
const GrandParent = () => (<Parent><Child /></Parent>);
const Parent = ({children}) => (<div>{children}</div>);
const Child = () => <span>Child Component</span>;
Using render props, we can easily make data access more streamlined:
const GrandParent = () => (
<DataProvider render={data => (
<Parent>
<Child data={data} />
</Parent>
)} />
);
This approach results in cleaner components and decreases complexity.
Use Cases and Scenarios
Render props shine in various scenarios. Here are some common use cases:
- Dynamic Forms: Create a
Form
component that accepts a render prop to generate dynamic form fields based on data passed by the client. - Authentication: Develop a
RequireAuth
component that wraps around protected routes, rendering children only if a user is authenticated. - Data Fetching: Use a
FetchData
component to fetch data from an API, with the ability to customize how that data is displayed through render props.
Best Practices for Using Render Props
While render props can be powerful, there are some best practices to keep in mind:
- Avoid Heavy Logic in Render Props: Avoid placing complex logic inside the render prop itself. Try to keep it simple and focus on making your components clean and easy to read.
- Memoization: Use
React.memo()
oruseMemo()
to prevent unnecessary re-renders when render props receive the same values. - Consistent API: Ensure that your render props maintain a consistent API when interacting with child components so that developers using your library don’t get confused.
- Combine with Context API: For shared states across many components, consider combining with React's Context API to enhance state management across render props.
Conclusion
Render props are a powerful pattern that fosters both reusability and flexibility within your React components. As your applications grow in complexity, leveraging this pattern can help you manage state and behaviors in a way that makes components easier to read and maintain.
By adopting the render props pattern in your React applications, you create a cleaner separation of concerns, allowing each component to focus on its specific responsibility while still collaborating effectively.
Explore this design pattern, and integrate it where appropriate to enhance modularity within your codebase. As you continue improving your React skills, you'll find that render props are just one of the many tools at your disposal for building sophisticated user interfaces with ease.