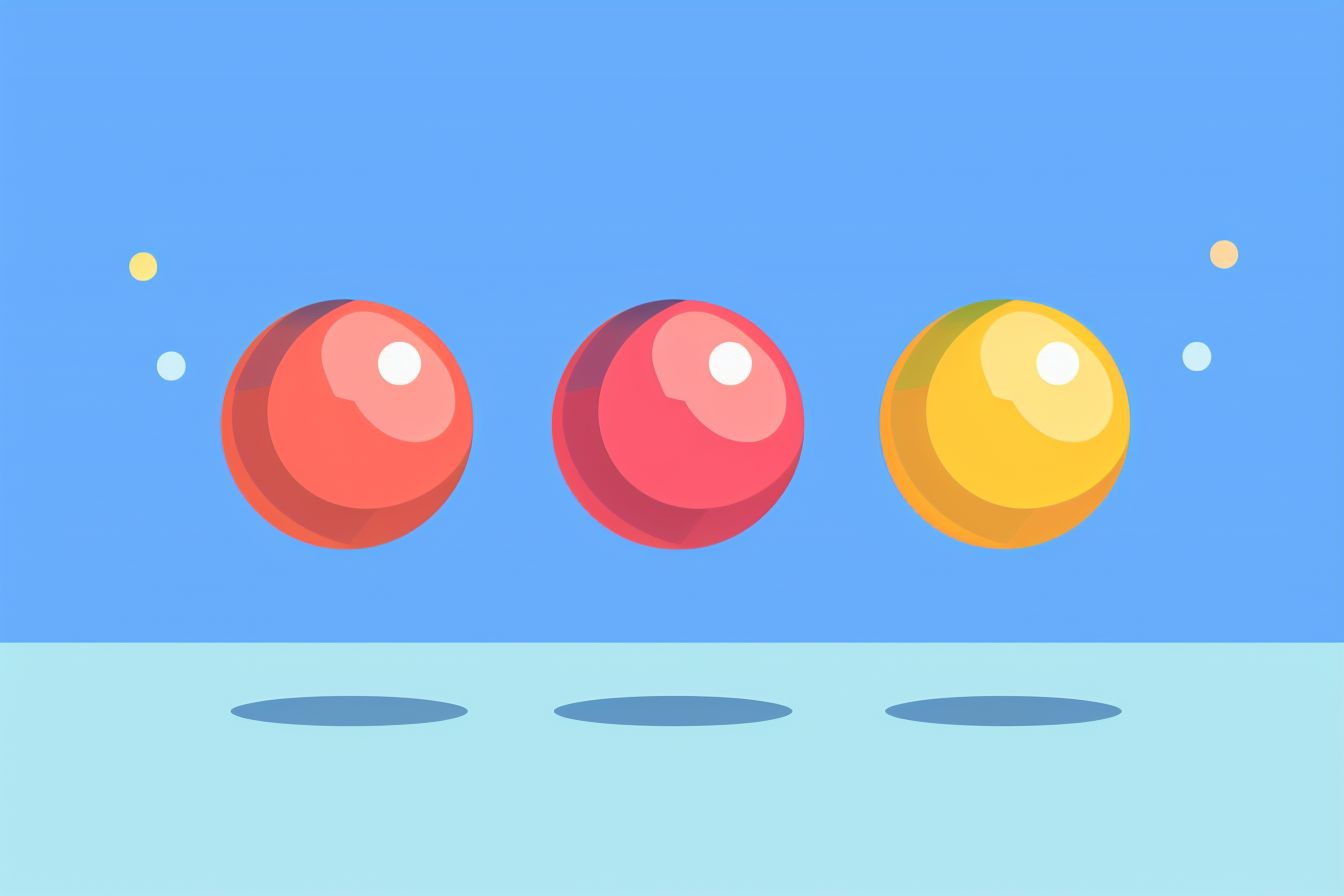
Mastering Asynchronous JavaScript with Promises, Async/Await, and Generators
Elevate Your JavaScript Skills by Delving into Advanced Asynchronous Patterns
Jul 24, 2024 - 13:11 • 4 min read
Introduction
In modern web development, dealing with asynchronous code is inevitable. Whether it’s API calls, timers, or event listeners, the ability to handle asynchronous operations is crucial. JavaScript offers several ways to manage this, each with its own set of advantages and complexities. This post aims to demystify three core asynchronous patterns in JavaScript: Promises, async/await, and generators.
We'll explore these through detailed explanations, code samples, and best practices. By the end of this guide, you’ll have a more profound understanding of how to write and optimize asynchronous JavaScript.
Promises: The Foundation of Asynchronous JavaScript
What Are Promises?
A Promise is an object representing the eventual completion or failure of an asynchronous operation. It allows you to chain operations and handle errors more gracefully than traditional callback-based code.
Basic Promise Example
const fetchData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Data fetched!');
}, 1000);
});
};
fetchData().then(data => console.log(data)).catch(error => console.error(error));
Chaining Promises
One of the strengths of Promises is their ability to chain multiple asynchronous operations.
Chaining Example
fetchData()
.then(data => {
console.log(data);
return fetchData();
})
.then(newData => console.log(newData))
.catch(error => console.error(error));
However, as you chain more Promises, the code can become harder to read and maintain. This is where async/await
comes in.
Async/Await: Syntactic Sugar Over Promises
What is Async/Await?
async/await
allows you to write asynchronous code in a synchronous-looking manner. It makes your code cleaner and easier to read.
Basic Example of Async/Await
const fetchDataAsync = async () => {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error(error);
}
};
fetchDataAsync();
Error Handling with Async/Await
Using try/catch blocks with async/await
makes error handling straightforward.
Error Handling Example
const fetchDataWithErrorHandling = async () => {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
};
fetchDataWithErrorHandling();
Generators: The Power Users’ Tool for Async Operations
Understanding Generators
Generators are functions that can be paused and resumed, making them incredibly powerful for handling complex asynchronous workflows.
Basic Generator Example
function* generatorFunction() {
yield 'First yield';
yield 'Second yield';
return 'Finished';
}
const gen = generatorFunction();
console.log(gen.next().value); // 'First yield'
console.log(gen.next().value); // 'Second yield'
console.log(gen.next().value); // 'Finished'
Using Generators for Asynchronous Operations
Generators can be combined with Promises to handle asynchronous operations in a controlled manner.
Async Generator Example
function* asyncGenerator() {
const data1 = yield fetchData();
console.log(data1);
const data2 = yield fetchData();
console.log(data2);
}
function handleGenerator(gen) {
const iterator = gen();
function handle(result) {
if (result.done) return;
const value = result.value;
value.then(res => handle(iterator.next(res)));
}
handle(iterator.next());
}
handleGenerator(asyncGenerator);
Combining Generators with Async/Await
You can also use async/await in conjunction with Generators for more readable and manageable async code.
Combining Example
async function asyncFunction() {
return 'Async data';
}
function* generatorWithAsync() {
const data = yield asyncFunction();
console.log(data);
}
function handleAsyncGenerator(gen) {
const iterator = gen();
function handle(result) {
if (result.done) return;
const value = result.value;
if (value instanceof Promise) {
value.then(res => handle(iterator.next(res)));
} else {
handle(iterator.next(value));
}
}
handle(iterator.next());
}
handleAsyncGenerator(generatorWithAsync);
Best Practices for Asynchronous JavaScript
- Use Promises for Simpler Tasks: Promises are easier to read and manage for straightforward asynchronous operations.
- Adopt Async/Await for Better Readability: Write asynchronous code in a synchronous style to improve readability and maintainability.
- Harness the Power of Generators for Complex Workflows: Use Generators to manage complex async workflows where state and control flow need more granularity.
- Always Handle Errors: Whether using Promises, async/await, or Generators, ensure you handle errors appropriately.
- Leverage Libraries: Utilize libraries like
axios
for HTTP requests orbluebird
for advanced Promise utilities.
Conclusion
Mastering asynchronous JavaScript goes beyond knowing how to use Promises, async/await, and Generators. It involves understanding when and why each method is appropriate, and combining them effectively to create clean, efficient, and maintainable code. By adopting best practices and leveraging the strengths of each asynchronous pattern, you can elevate your JavaScript development skills to new heights.