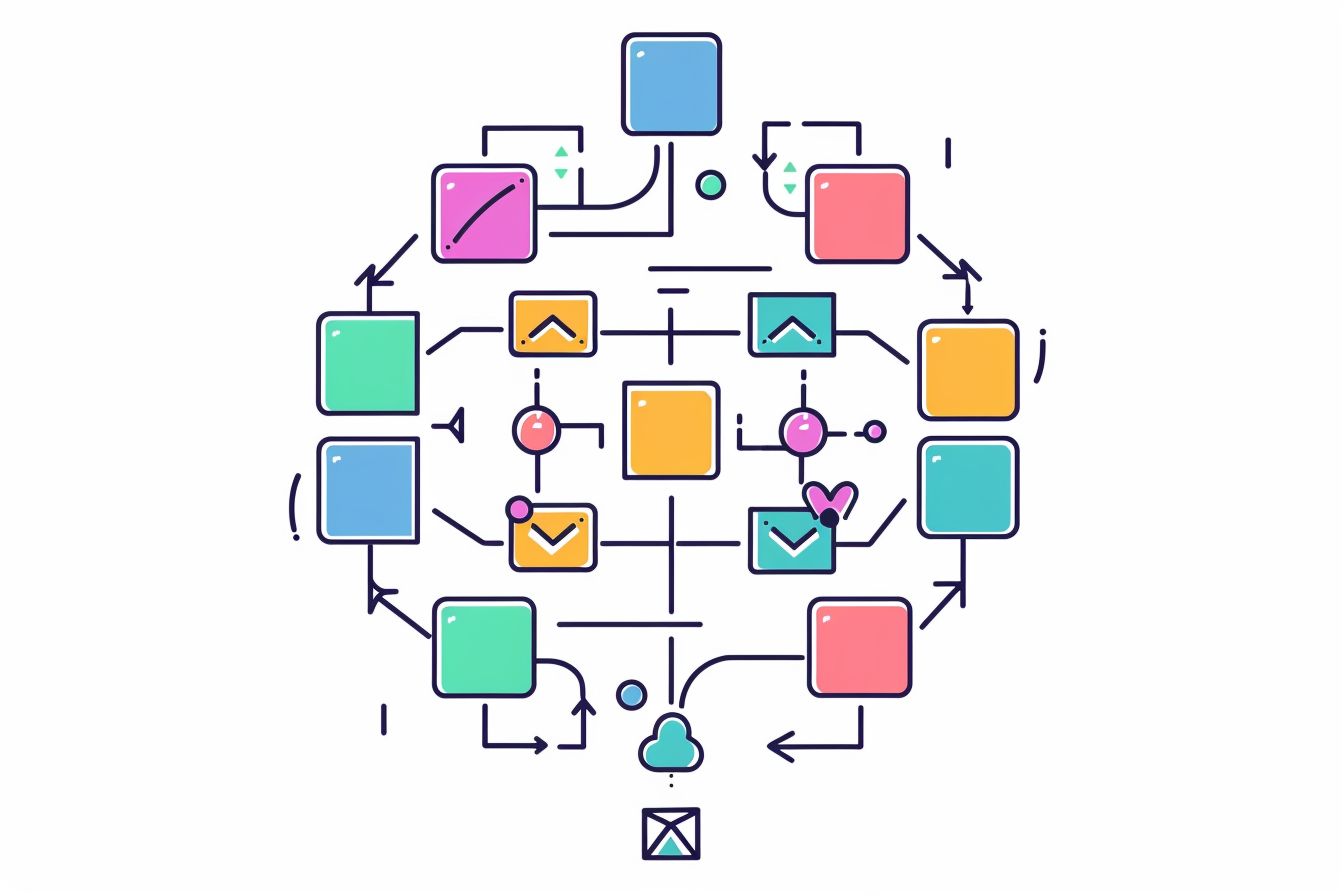
Managing Complex State Transitions in React with Finite State Machines
A detailed exploration of state management using finite state machines in React applications.
Jul 25, 2024 - 15:01 • 5 min read
In the fast-evolving world of front-end development, managing application state has come to represent both a challenge and an opportunity, particularly in complex applications. As the user experience demands grow more sophisticated, developers often find themselves wrestling with how to maintain clear, predictable state transitions within their applications.
This is where finite state machines (FSMs) emerge as a powerful tool. Finite state machines are a model of computation that can help manage states and transitions in a predictable and manageable way. They represent an abstract machine that can be in one of a finite number of states at any given time, transitioning from one state to another based on inputs.
In this post, you'll learn about the integration of FSMs into React applications, leveraging libraries such as XState to create more reliable and maintainable state management solutions. We'll cover the motivations behind using FSMs, how they enhance the predictability of state within applications, and provide real-world examples showcasing their implementation.
Understanding Finite State Machines
Before diving into the implementation details, let’s clarify the concept of a finite state machine. In its basic form, an FSM is represented by:
- States: Represents different conditions or statuses of the application.
- Transitions: The movement from one state to another based on specific events or actions called 'inputs'.
- Events: Triggers that cause transitions to occur.
- Initial State: The starting point of the FSM.
The beauty of FSMs lies in their clarity and rigor. You know exactly what states exist and under what circumstances your application will transition between these states. This level of predictability becomes increasingly important as application complexity grows.
Key Benefits of Using FSMs in React
- Predictability: Understanding how states change allows for easier debugging and maintenance.
- Separation of Concerns: State management logic is decoupled from UI logic, enhancing modularity.
- Visual Representation: FSMs are inherently visual. This makes it easier for team members to grasp the flow of state changes.
- Better Testing: Clearly defined states and transitions lead to more robust unit tests.
Installing XState
For our implementation, we will make use of XState, a popular JavaScript library for creating, interpreting, and executing finite state machines and statecharts.
To install XState, use:
npm install xstate
Or if you're using Yarn:
yarn add xstate
Building a Simple FSM with XState
Let's create a simple counter application with three states: idle
, incrementing
, and decrementing
. This application will allow us to increment or decrement a value based on user input.
import React from "react";
import { createMachine } from "xstate";
import { useMachine } from "@xstate/react";
// Define the state machine
const counterMachine = createMachine({
id: "counter",
initial: "idle",
states: {
idle: {
on: {
INCREMENT: "incrementing",
DECREMENT: "decrementing",
},
},
incrementing: {
after: {
1000: "idle",
},
},
decrementing: {
after: {
1000: "idle",
},
},
},
});
function Counter() {
const [state, send] = useMachine(counterMachine);
const count =
state.value === "incrementing" ? (prev) => prev + 1 : (prev) => prev - 1;
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => send("INCREMENT")}>Increment</button>
<button onClick={() => send("DECREMENT")}>Decrement</button>
</div>
);
}
export default Counter;
Explanation of the Code
- Creating the Machine: The
createMachine
function defines the states and transitions of our finite state machine. Here, it specifies that the machine is in anidle
state initially and can transition toincrementing
ordecrementing
based on user actions. - State Transitions: The state machine defines transitions that will occur after 1000ms in both
incrementing
anddecrementing
states, returning to theidle
state. - Sending Events: The
send
function dispatches events ('INCREMENT' or 'DECREMENT') to transition between states, driving the state of the counter.
Enhancing the Application with Context
As the application complexity grows, it may be beneficial to introduce the concept of Context to share state across components without prop drilling. Here's how to implement a CounterContext.
Using React Context with XState
import React, { createContext } from "react";
import { createMachine } from "xstate";
import { useMachine } from "@xstate/react";
const CounterContext = createContext();
const counterMachine = createMachine({
// same as before
});
export function CounterProvider({ children }) {
const [state, send] = useMachine(counterMachine);
return (
<CounterContext.Provider value={{ state, send }}>
{children}
</CounterContext.Provider>
);
}
export function useCounter() {
return React.useContext(CounterContext);
}
Accessing State in Child Components
Now we can use our useCounter
hook to access state and send actions in any child component.
function CounterDisplay() {
const { state } = useCounter();
return <h1>Count: {state.context.count}</h1>;
}
function CounterControls() {
const { send } = useCounter();
return (
<div>
<button onClick={() => send("INCREMENT")}>Increment</button>
<button onClick={() => send("DECREMENT")}>Decrement</button>
</div>
);
}
Putting it All Together
Wrap your components in the CounterProvider
to give them access to the state machine:
function App() {
return (
<CounterProvider>
<CounterDisplay />
<CounterControls />
</CounterProvider>
);
}
Conclusion
Using finite state machines in React can drastically enhance the reliability and maintainability of your applications as they grow in complexity. By leveraging tools like XState, developers can create clear, predictable state transitions that are easier to understand, test, and visualize.
This approach not only reduces bugs and unexpected behaviors but also contributes to better overall application architecture. Take the time to explore FSMs and consider incorporating them into your next React project to see the difference in state management clarity firsthand.