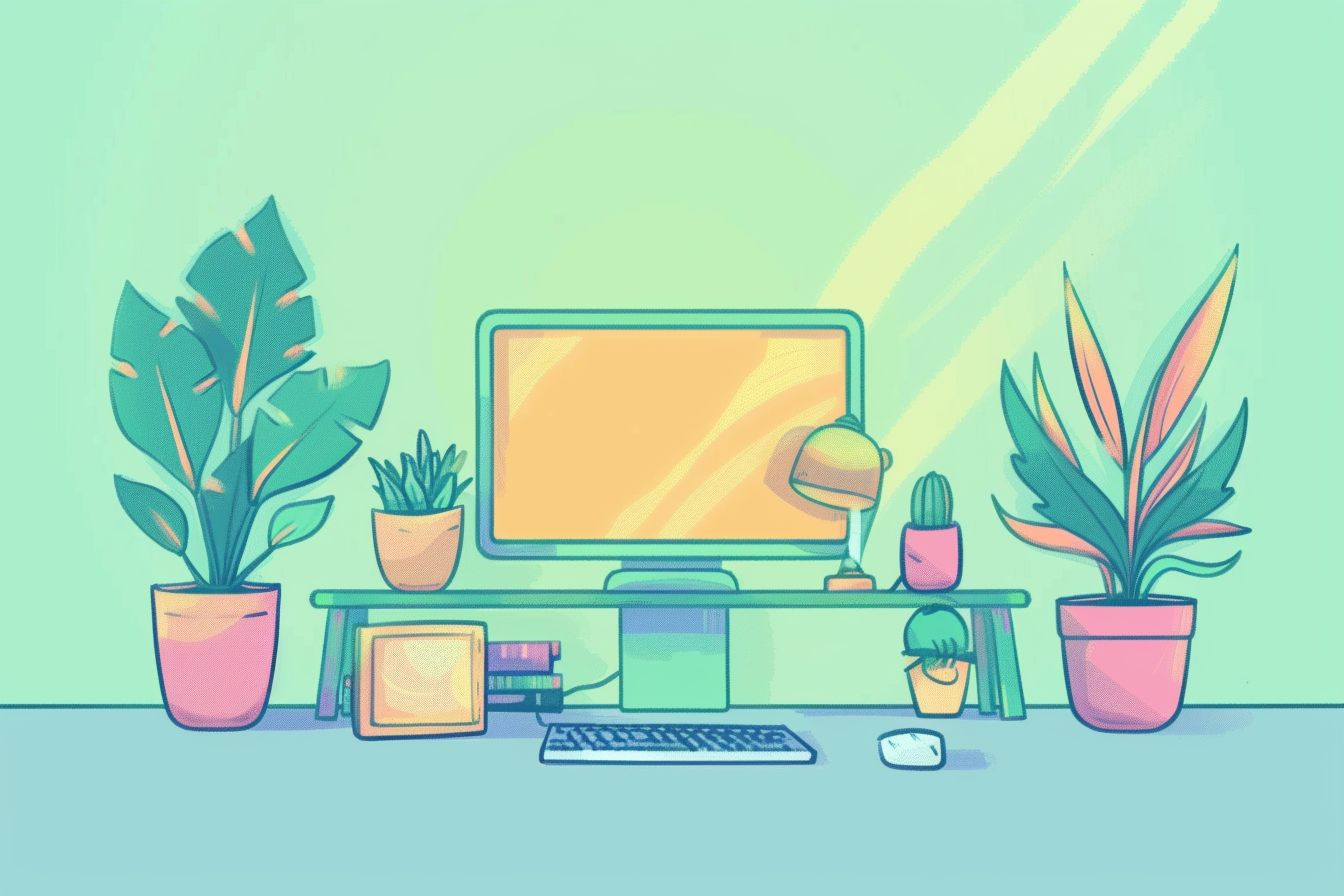
Enhancing React Apps with Advanced Animation Patterns
Unlock the secrets to crafting seamless animations using React and popular libraries
Jul 24, 2024 - 12:05 • 3 min read
Animations can significantly enhance user experience, making your React applications more engaging and intuitive. However, integrating smooth and efficient animations can be challenging. In this post, we will explore advanced animation patterns in React, leveraging libraries like Framer Motion, React Spring, and GSAP. We'll dive deep into their intricacies, showcase examples, and highlight best practices.
The Importance of Animations in Web Development
Animations are more than just eye candy; they provide visual feedback to users, guide their attention, and improve the overall flow of an application. With React, integrating complex animations becomes more manageable, thanks to a range of powerful libraries.
1. Framer Motion: A Deep Dive
Framer Motion is a robust library that offers declarative animations for React. Its API is intuitive, and it simplifies creating animations and gesture interactions.
import { motion } from 'framer-motion';
const Box = () => (
<motion.div
animate={{ scale: 1.5 }}
transition={{ duration: 0.5 }}
/>
);
Advanced Animations with Variants
Variants in Framer Motion allow us to define multiple animation states and switch between them easily.
const boxVariants = {
hidden: { opacity: 0 },
visible: { opacity: 1, transition: { duration: 2 } },
};
const Box = () => (
<motion.div
initial="hidden"
animate="visible"
variants={boxVariants}
/>
);
2. React Spring: Physics-based Animations
React Spring focuses on physics-based animations, providing a natural look and feel. It's based on the idea of dampened springs rather than linear interpolations.
import { useSpring, animated } from 'react-spring';
const Box = () => {
const styles = useSpring({ to: { opacity: 1 }, from: { opacity: 0 }, delay: 500 });
return <animated.div style={styles} />;
};
Using Trail Animations
Trail animations animate a list of elements with a delay between each.
import { useTrail, animated } from 'react-spring';
const items = ['item1', 'item2', 'item3'];
const trail = useTrail(items.length, {
opacity: 1,
from: { opacity: 0 },
});
return (
<>
{trail.map((style, index) => (
<animated.div key={index} style={style}>
{items[index]}
</animated.div>
))}
</>
);
3. GSAP: The GreenSock Animation Platform
GSAP is a powerful JavaScript library well-known for its performance and flexibility. GSAP's React integration allows seamless animations within React components.
import { useRef, useEffect } from 'react';
import { gsap } from 'gsap';
const Box = () => {
const boxRef = useRef();
useEffect(() => {
gsap.to(boxRef.current, { duration: 1, x: 100 });
}, []);
return <div ref={boxRef} />;
};
Using Timelines for Complex Animations
Timelines in GSAP allow you to chain multiple animation steps together in an orderly fashion.
const tl = gsap.timeline();
t
.to('.box1', { duration: 1, x: 100 })
.to('.box2', { duration: 1, y: 50 })
.to('.box3', { duration: 1, opacity: 0.5 });
Best Practices
- Optimize Performance: Use CSS transforms over properties that trigger layout changes (like width, height, margin, etc.).
- Avoid Overuse: Too many animations can overwhelm users. Use animations purposefully.
- Test Across Devices: Ensure your animations perform well on various devices, especially on lower-powered hardware.
- Maintainability: Abstract and encapsulate animation logic within reusable components or custom hooks.
Conclusion
Animations play a crucial role in creating engaging user interfaces. By leveraging libraries like Framer Motion, React Spring, and GSAP, you can easily integrate complex animations into your React applications. Remember to follow best practices to ensure your animations are both performant and purposeful.