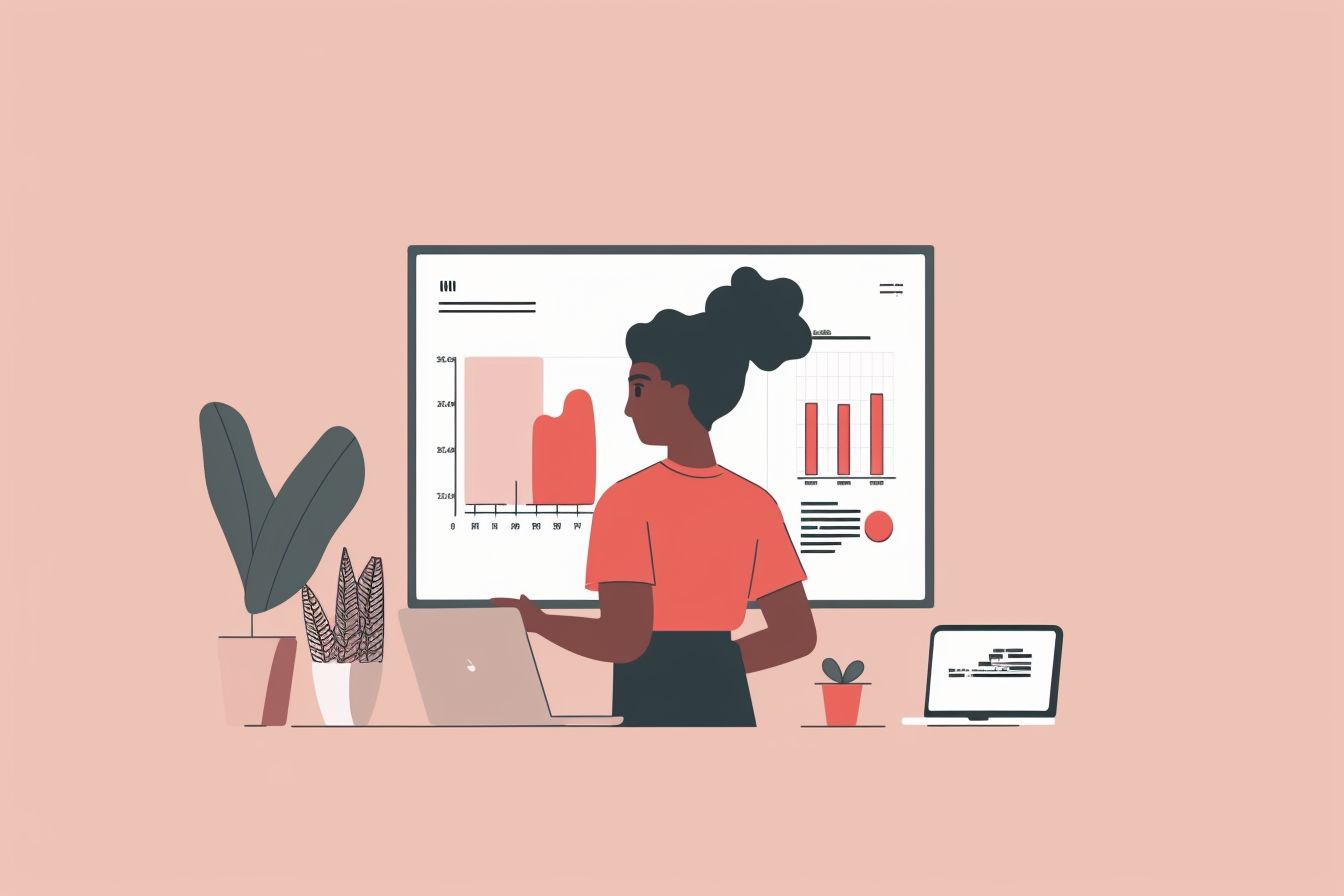
Building a Custom Animation Library with React Hooks
Elevate your UI with bespoke animations using Hooks for seamless transitions.
Jul 26, 2024 - 11:23 • 4 min read
In the world of web development, animations can significantly enhance user experience by making web applications more interactive and visually engaging.
React, being a powerful library itself, has tools such as useState
and useEffect
that help us create dynamic animations. But when we want to build a custom animation library tailored to our specific needs, React Hooks provide a powerful way to achieve expressive, flexible, and reusable animations without the bloat of heavy libraries.
Why Custom Animations?
While libraries like Framer Motion and React Spring offer excellent pre-built functionalities for animations, sometimes, we need more control over our animations. Custom solutions allow developers to define the behavior of animations, improve performance by avoiding unnecessary re-renders, and reduce bundle size by eliminating unused code.
Let’s dive into creating our own custom animation library!
Setting Up the Animation Hook
We'll start by creating a hook called useAnimation
that will manage the state of our animations and trigger transitions. Using the power of React refs and state, we can dynamically control our animations.
Here’s the basic setup for our useAnimation
hook:
import { useState, useEffect, useRef } from 'react';
const useAnimation = (duration = 300) => {
const [isAnimating, setIsAnimating] = useState(false);
const elementRef = useRef(null);
const startAnimation = () => {
setIsAnimating(true);
setTimeout(() => {
setIsAnimating(false);
}, duration);
};
return { elementRef, isAnimating, startAnimation };
};
Adding Transition Styles
Next, we will define some basic CSS transition styles that will be applied to the element we are animating. Use inline styles or CSS classes depending on your preference. For this example, we'll use inline styles to apply during the animation:
const getTransitionStyles = (isAnimating) => ({
transition: `all ${isAnimating ? '0.3s' : '0s'} ease`,
opacity: isAnimating ? 1 : 0,
transform: isAnimating ? 'translateY(0)' : 'translateY(-20px)',
});
Using the Animation Hook in a Component
Now, we can integrate our useAnimation
hook into a functional component:
import React from 'react';
import useAnimation from './useAnimation';
const AnimatedComponent = () => {
const { elementRef, isAnimating, startAnimation } = useAnimation(300);
return (
<div>
<button onClick={startAnimation}>Animate</button>
<div ref={elementRef} style={getTransitionStyles(isAnimating)}>
I will animate!
</div>
</div>
);
};
Here, we've set up a button that triggers the animation, and our animated element changes its opacity and vertical position when the animation starts.
Advanced Features
Now that we have a basic custom animation setup, let's take it a step further by adding more capabilities.
- Animation Directions
We can enhance the hook to allow different animation directions and properties:
const useAnimation = (duration = 300, direction = 'up') => {
const [isAnimating, setIsAnimating] = useState(false);
const elementRef = useRef(null);
const startAnimation = () => {
setIsAnimating(true);
setTimeout(() => {
setIsAnimating(false);
}, duration);
};
const getTransitionStyles = () => {
const styles = {
transition: `all ${isAnimating ? '0.3s' : '0s'} ease`,
opacity: isAnimating ? 1 : 0,
};
styles.transform = isAnimating
? direction === 'up' ? 'translateY(0)' : 'translateY(20px)'
: direction === 'up' ? 'translateY(-20px)' : 'translateY(0)';
return styles;
};
return { elementRef, isAnimating, startAnimation, getTransitionStyles };
};
- Chaining Animations
By tweaking our approach, we could allow chaining of animations. For example, we can add a callback function to execute after the animation finishes:
const startAnimation = (callback) => {
setIsAnimating(true);
setTimeout(() => {
setIsAnimating(false);
if (callback) callback();
}, duration);
};
- Reusable Animations
For reusable animations, consider defining preset animations that allow users to specify the animation type:
const useAnimation = (animationType, duration = 300) => {
// Animation logic with cases for different types
switch (animationType) {
case 'fade':
// Fade logic here
break;
// Other cases...
}
};
Conclusion
Creating a custom animation library using React Hooks can offer lightweight, efficient, and reusable solutions to address the needs of your projects. By abstracting away animation logic into a hook, you increase the modularity and maintainability of your codebase. Plus, having the control to implement animations exactly how you envision can lead to a richer user experience that aligns perfectly with the look and feel of your application.
Through this post, you should now have a solid idea of how to create and extend a custom animation library in React. The possibilities are endless—from simple transitions to complex chained animations, the sky's the limit. So get creative, and start incorporating animations into your projects!