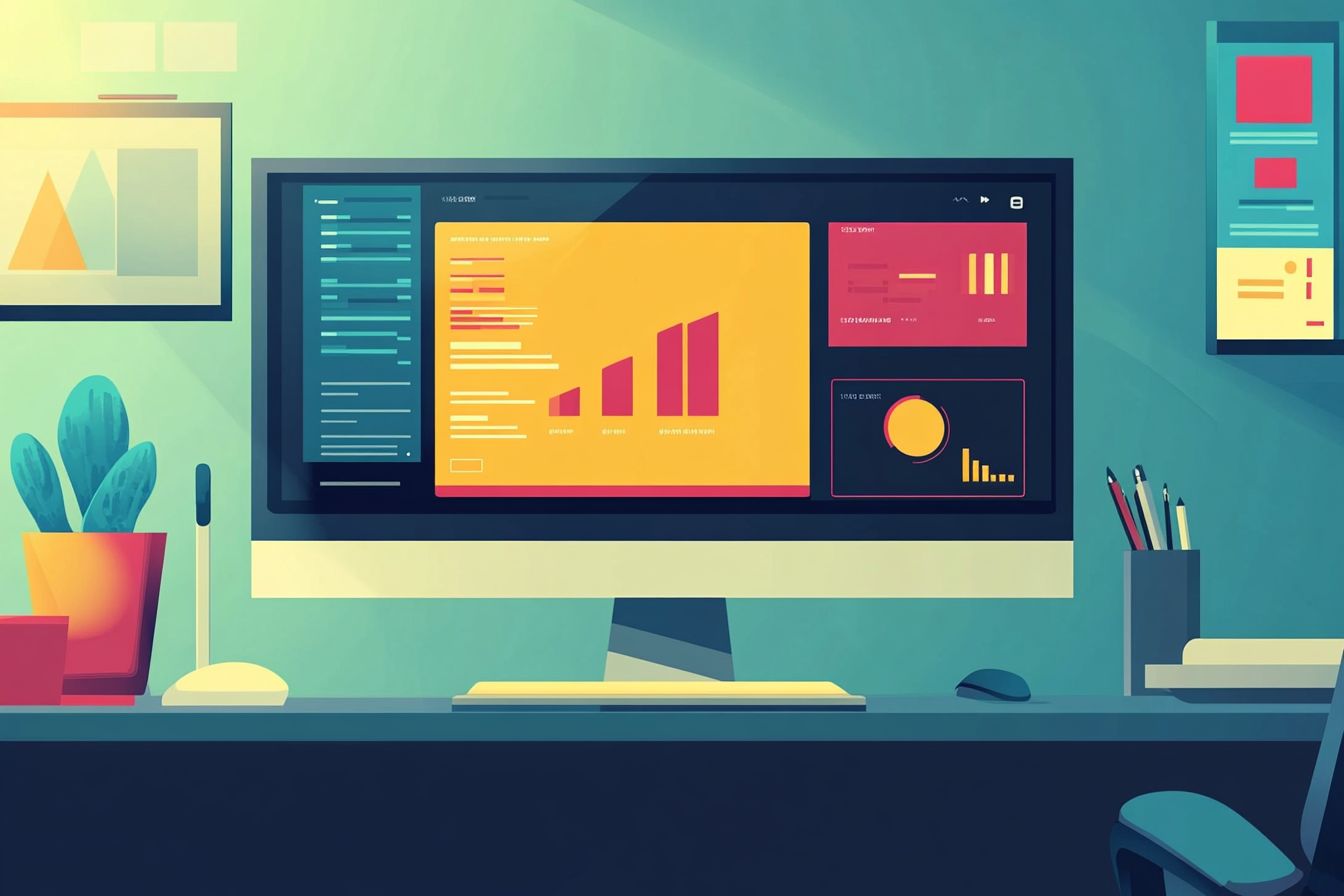
Getting Started with useTransition in React
Enhancing performance and user experience with async transitions.
Aug 12, 2024 - 12:02 • 4 min read
Understanding React's useTransition Hook
React 18 introduced several new features to improve the performance and user experience of applications, one of which is the useTransition
Hook. Understanding how to effectively implement this Hook can significantly enhance your application's responsiveness, especially when dealing with slow or potentially blocking updates.
useTransition
?
What is useTransition
is a Hook that allows developers to mark certain updates as "transitions". It allows you to defer a less critical state update while keeping the UI responsive for user interactions. In simpler terms, it helps to avoid blocking updates, ensuring that users can still interact with the app while some tasks are still processing.
When using this Hook, updates that are marked as transitions might take longer, but they don’t block the main thread from rendering critical updates. This means your app remains snappy even as it processes large datasets or makes complex changes.
Getting Started
Firstly, let's install React 18 if you haven't done so yet:
npm install react@18 react-dom@18
Next, we can implement the useTransition
Hook in our React component. Here’s a simple example of how to use it:
import React, { useState, useTransition } from 'react';
function App() {
const [isPending, startTransition] = useTransition();
const [count, setCount] = useState(0);
const handleClick = () => {
startTransition(() => {
setCount((prevCount) => prevCount + 1);
});
};
return (
<div>
<button onClick={handleClick}>Increment</button>
<h1>{isPending ? 'Updating...' : `Count: ${count}`}</h1>
</div>
);
}
How It Works
In the above example, we create a counter that increments when the button is clicked. The useTransition
Hook returns an isPending
boolean that indicates whether the transition is in progress. The startTransition
function is used to wrap any updates we want to mark as transitions.
When startTransition
is called, it allows React to delay rendering this update until the browser has completed the more urgent updates. In our case, while counting incrementally, if the button is pressed rapidly, the component won’t feel sluggish but will still update correctly when processing completes.
useTransition
Key Advantages of - User Experience: The most significant advantage is the improved UX. Users can see immediate response for interactions while the app processes potentially hefty computations.
- Combine with
Suspense
: It pairs beautifully with React Suspense. You can use Suspense to show a loading state while some data is being fetched in the background. - Improved Performance: For complex applications, having the ability to prioritize updates makes a big difference in application performance. The app feels much faster and more responsive.
Transition States
You can also manage more sophisticated states with useTransition
. For example, we can manage multiple transition states and show different loading indicators based on the context:
function ComplexTransition() {
const [isPending, startTransition] = useTransition();
const [count, setCount] = useState(0);
const [items, setItems] = useState([]);
const handleAddItem = () => {
startTransition(() => {
setItems((prev) => [...prev, `Item ${count}`]);
setCount((prevCount) => prevCount + 1);
});
};
return (
<div>
<button onClick={handleAddItem}>Add Item</button>
<h1>{isPending ? 'Adding item...' : `Item Count: ${items.length}`}</h1>
<ul>
{items.map((item) => <li key={item}>{item}</li>)}
</ul>
</div>
);
}
Here we manage two pieces of state while also marking the operation of adding items as a transition. This way, the user sees a loading message until the items are fully added.
Best Practices
When using useTransition
, keep the following best practices in mind:
- Use
startTransition
for non-urgent state updates that can be delayed. - Ensure to handle the
isPending
state to improve user feedback. - Test with different component structures to see how it enriches user experiences based on your app’s unique data needs.
Conclusion
The useTransition
Hook is a powerful tool for any developer looking to optimize the user experience in React applications, especially those that require robust rendering of data-heavy interfaces. By understanding and implementing this Hook, you can significantly reduce any noticeable lag that comes from intensive computational tasks in your application.
As always, with new features, it's crucial to test and ensure that they fit well within your performance goals and user experience design. Happy coding!