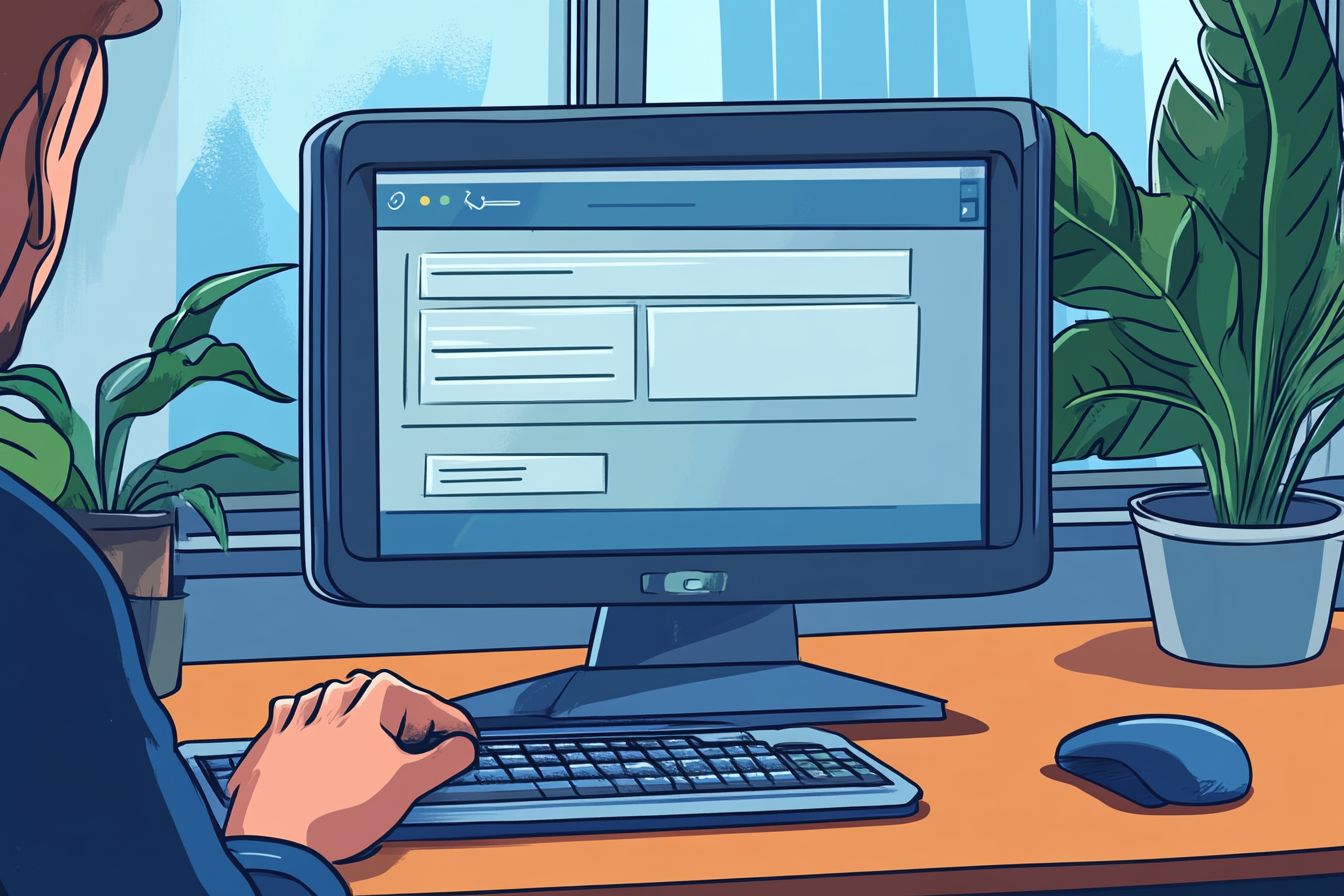
Customizing Forms with React and Custom Hooks
Harnessing the power of custom hooks for dynamic form management
Aug 09, 2024 - 15:53 • 6 min read
Forms are a fundamental part of web applications, serving as the primary interaction point between users and the application. With the introduction of React hooks, managing form state has become significantly more manageable and less error-prone. In this post, we will dive deep into the intricacies of creating dynamic forms using custom hooks, advanced validation techniques, and state management.
Understanding the Complexities of Form Management in React
Before we jump into code, let's understand why forms can be tricky. Traditional state management in forms, especially those with dynamic fields, can lead to a tangled mess of state and effect hooks. The challenges include maintaining input state, handling validation, and updating form data based on user interactions.
Building a Custom Hook for Form Management
Let’s create a custom hook called useForm
that encapsulates the logic for managing our form state. This hook will simplify form management by ensuring that we can focus on rendering our forms without tangled logic. Here’s a basic implementation:
import { useState } from 'react';
function useForm(initialValues) {
const [values, setValues] = useState(initialValues);
const handleChange = (e) => {
const { name, value } = e.target;
setValues({ ...values, [name]: value });
};
const resetForm = () => {
setValues(initialValues);
};
return [values, handleChange, resetForm];
}
In this hook, we utilize the useState
hook to hold our form values. The handleChange
function updates the state when an input changes, and resetForm
allows us to reset the form back to its initial state.
useForm
Hook in a Component
Utilizing the Now that we have our useForm
hook, let's see how we can integrate it into a component. Here’s a simple form component using our custom hook:
import React from 'react';
import useForm from './useForm';
function MyForm() {
const [formValues, handleChange, resetForm] = useForm({ name: '', email: '' });
const handleSubmit = (e) => {
e.preventDefault();
console.log('Submitted: ', formValues);
resetForm();
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Name:</label>
<input type="text" name="name" value={formValues.name} onChange={handleChange} />
</div>
<div>
<label>Email:</label>
<input type="email" name="email" value={formValues.email} onChange={handleChange} />
</div>
<button type="submit">Submit</button>
</form>
);
}
In this example, MyForm
component uses the useForm
hook to manage the state of the form inputs. Once the user submits the form, the handleSubmit
function is called, which logs the form data and resets the form.
Advanced Validation Techniques
While our useForm
hook is sufficient for managing form input, we can extend it to include advanced validation checks. By adding a validation function as a parameter, we can validate input before updating the state. Here’s how we can adjust our useForm
hook:
import { useState } from 'react';
function useForm(initialValues, validate) {
const [values, setValues] = useState(initialValues);
const [errors, setErrors] = useState({});
const handleChange = (e) => {
const { name, value } = e.target;
setValues({ ...values, [name]: value });
setErrors(validate({ ...values, [name]: value }));
};
const resetForm = () => {
setValues(initialValues);
setErrors({});
};
return [values, errors, handleChange, resetForm];
}
In our updated useForm
hook, we now track errors
as well, which holds validation error messages. We validate the input on change, allowing us to give immediate feedback to users.
Example Validation Function
Now let’s create a simple validation function that we can pass into our hook:
function validateForm(values) {
let errors = {};
if (!values.name) {
errors.name = 'Name is required';
}
if (!values.email) {
errors.email = 'Email is required';
} else if (!/S+@S+.S+/.test(values.email)) {
errors.email = 'Email address is invalid';
}
return errors;
}
This function checks if the name and email fields are filled and validates the email format.
Integrating Validation with the Form
Now … let’s modify our MyForm
component to incorporate the new validation logic:
import React from 'react';
import useForm from './useForm';
import validateForm from './validateForm';
function MyForm() {
const [formValues, errors, handleChange, resetForm] = useForm({ name: '', email: '' }, validateForm);
const handleSubmit = (e) => {
e.preventDefault();
console.log('Submitted: ', formValues);
resetForm();
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Name:</label>
<input type="text" name="name" value={formValues.name} onChange={handleChange} />
{errors.name && <p>{errors.name}</p>}
</div>
<div>
<label>Email:</label>
<input type="email" name="email" value={formValues.email} onChange={handleChange} />
{errors.email && <p>{errors.email}</p>}
</div>
<button type="submit">Submit</button>
</form>
);
}
In this version of MyForm
, we display error messages underneath the relevant input fields, enhancing the user experience and providing real-time feedback based on input validation.
Dynamic Fields with Context
In many applications, forms need to handle dynamic fields. For example, an application may require collecting additional information based on user input. We can extend our form management to handle dynamic fields by leveraging React's context API. Here’s how to set up a simple context to manage an array of dynamic fields:
import React, { createContext, useContext, useState } from 'react';
const DynamicFormContext = createContext();
export const useDynamicForm = () => {
return useContext(DynamicFormContext);
};
export const DynamicFormProvider = ({ children }) => {
const [fields, setFields] = useState([{ name: '', email: '' }]);
const addField = () => {
setFields([...fields, { name: '', email: '' }]);
};
return (
<DynamicFormContext.Provider value={{ fields, setFields, addField }}>
{children}
</DynamicFormContext.Provider>
);
};
With this context, we can manage multiple form fields as an array of objects. The addField
function allows us to add new fields dynamically.
Leveraging the Dynamic Form Context in a Component
Now we can create a dynamic form component that makes use of our DynamicFormProvider
context to render multiple MyForm
instances:
import React from 'react';
import { useDynamicForm, DynamicFormProvider } from './DynamicFormContext';
import useForm from './useForm';
import validateForm from './validateForm';
function DynamicForm() {
const { fields, addField } = useDynamicForm();
return (
<div>
{fields.map((field, index) => (
<MyForm key={index} initialValues={field} />
))}
<button onClick={addField}>Add Field</button>
</div>
);
}
function MyForm({ initialValues }) {
const [formValues, errors, handleChange, resetForm] = useForm(initialValues, validateForm);
const handleSubmit = (e) => {
e.preventDefault();
console.log('Submitted: ', formValues);
resetForm();
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Name:</label>
<input type="text" name="name" value={formValues.name} onChange={handleChange} />
{errors.name && <p>{errors.name}</p>}
</div>
<div>
<label>Email:</label>
<input type="email" name="email" value={formValues.email} onChange={handleChange} />
{errors.email && <p>{errors.email}</p>}
</div>
<button type="submit">Submit</button>
</form>
);
}
export default function App() {
return (
<DynamicFormProvider>
<DynamicForm />
</DynamicFormProvider>
);
}
Conclusion
This post illustrates that while managing form state in React, custom hooks provide a clean and maintainable solution for handling forms. With useForm
, we can streamline the form state management process and enhance the user experience through validation and dynamic field handling.
In today's web applications, where forms are vital for user interaction, mastering these techniques enables versatile and user-friendly applications. By implementing those practices, you'll be well on your way to rich, dynamic forms that delight users. Remember that the key to effective form management lies in modular design, reusable components, and separation of concerns, making your codebase cleaner and easier to understand and maintain.