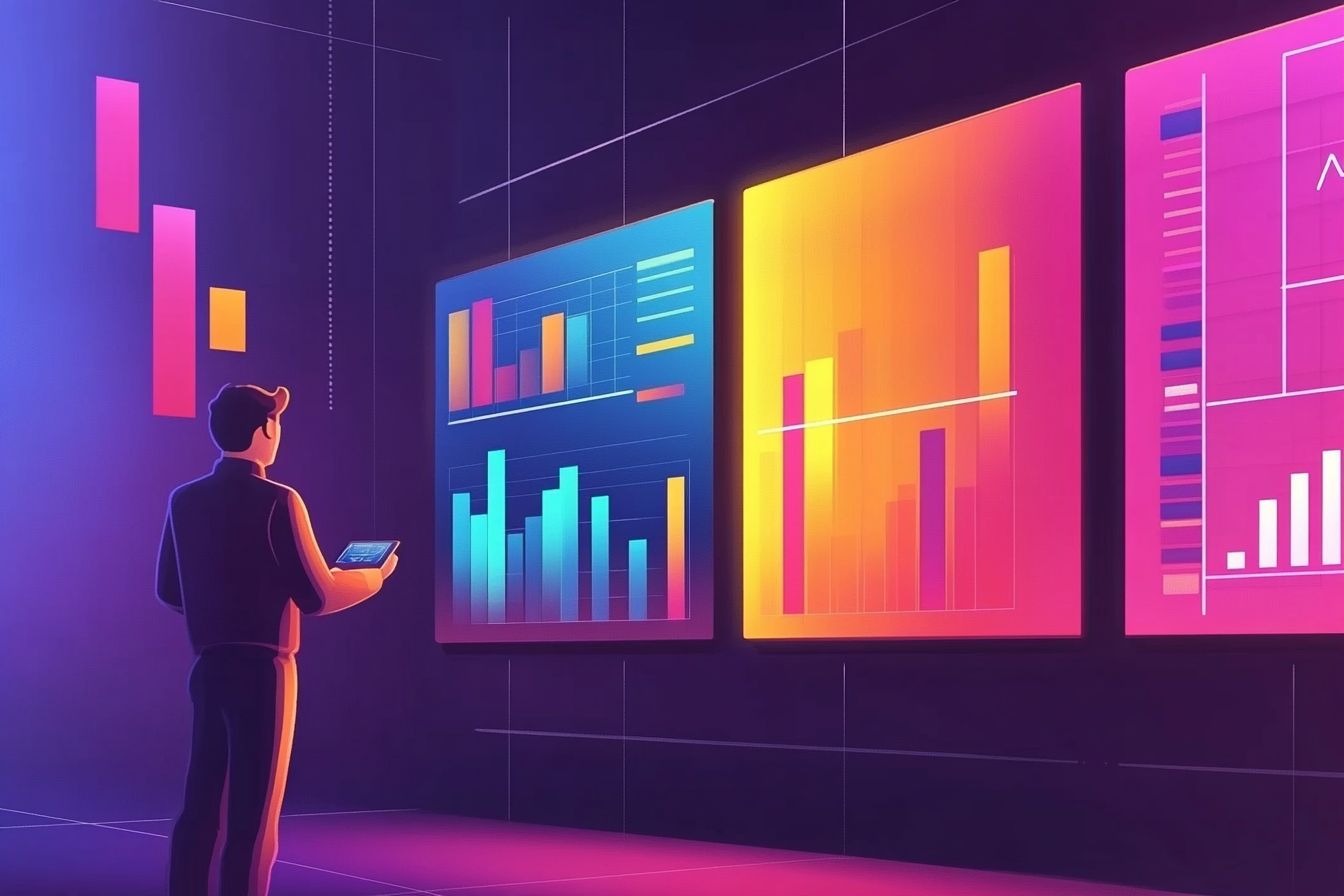
Building Efficient Data Visualization with React and D3.js
Master the integration of React with D3.js for stunning visualizations.
Aug 12, 2024 - 13:07 • 4 min read
Data visualization can be a complex task, especially when combining powerful libraries like D3.js with a component-based architecture like React. Each library has its strengths — D3.js excels in data manipulation and dynamic visualizations, while React provides a robust structure for building user interfaces. In this post, we will explore how to efficiently merge these two frameworks to create a seamless data visualization experience.
The Challenge of Integrating React and D3
When working with D3.js in a React app, the challenge lies in managing the DOM manipulations that D3 performs, which can conflict with React’s virtual DOM updates. React's goal is to minimize direct manipulation of the DOM for performance, while D3 is traditionally used for that purpose. Thus, we need to find a balance.
Setting Up the Environment
Before diving into code, let's set up a simple React application with D3.js installed. You can use create-react-app
to bootstrap your project:
npx create-react-app d3-react-integration
cd d3-react-integration
npm install d3
This will install React and D3.js, preparing the ground for our visualizations.
Creating a Simple Bar Chart
Now, let’s create a simple bar chart component using D3.js within a React functional component. We’ll start with a static dataset:
Sample Data
const data = [10, 20, 30, 40, 50];
The BarChart Component
In your src
folder, create a new file named BarChart.js
:
import React, { useEffect, useRef } from 'react';
import * as d3 from 'd3';
const BarChart = ({ data }) => {
const svgRef = useRef();
useEffect(() => {
const svg = d3.select(svgRef.current);
svg.selectAll('*').remove(); // Clear the previous content
const width = 500;
const height = 300;
const xScale = d3.scaleBand()
.domain(data.map((_, i) => i))
.range([0, width])
.padding(0.1);
const yScale = d3.scaleLinear()
.domain([0, d3.max(data)])
.range([height, 0]);
svg.attr('width', width)
.attr('height', height)
.selectAll('rect')
.data(data)
.enter()
.append('rect')
.attr('x', (d, i) => xScale(i))
.attr('y', d => yScale(d))
.attr('width', xScale.bandwidth())
.attr('height', d => height - yScale(d))
.attr('fill', 'teal');
}, [data]);
return <svg ref={svgRef} />;
};
export default BarChart;
Integrating BarChart in the App
Now, let’s use our BarChart
component in App.js
:
import React from 'react';
import BarChart from './BarChart';
const App = () => {
const data = [10, 20, 30, 40, 50];
return (
<div>
<h1>Simple Bar Chart</h1>
<BarChart data={data} />
</div>
);
};
export default App;
Understanding the Code
In our BarChart
component:
- We create a
ref
for the SVG element to manipulate it with D3. - In
useEffect
, we apply D3 operations directly on the DOM element referenced bysvgRef
. - The
data
prop triggers re-renders, allowing for dynamic updates.
Dynamic Data Updates
To make the bar chart dynamic, we can add a button that changes the data:
import React, { useState } from 'react';
import BarChart from './BarChart';
const App = () => {
const [data, setData] = useState([10, 20, 30, 40, 50]);
const updateData = () => {
const newData = data.map(d => Math.floor(Math.random() * 100));
setData(newData);
};
return (
<div>
<h1>Dynamic Bar Chart</h1>
<BarChart data={data} />
<button onClick={updateData}>Update Data</button>
</div>
);
};
export default App;
Evaluating Performance
While merging D3 with React, always remember performance implications. Here are best practices to consider:
- Selective Rendering: D3 should handle data-driven changes but try to let React manage component lifecycles.
- Minimize Direct DOM Manipulation: Avoid using D3 methods that manipulate the entire DOM if possible.
- Data Updates: Use
useEffect
to respond to prop changes efficiently.
Conclusion
Merging D3.js with React creates powerful visualizations while managing a clear data flow. This post outlined the integration step-by-step, starting from static data to dynamic updates. As your visualizations grow more complex, consider using D3 functionalities like transitions and animations for a better user experience. By adhering to functional programming principles and React's lifecycle, you can create maintainable, efficient, and stunning data visualizations.
Resources
In the future, we’ll explore advanced techniques, such as integrating React with other visualization libraries and incorporating responsive design principles. Feel free to experiment and build on these concepts in your projects!