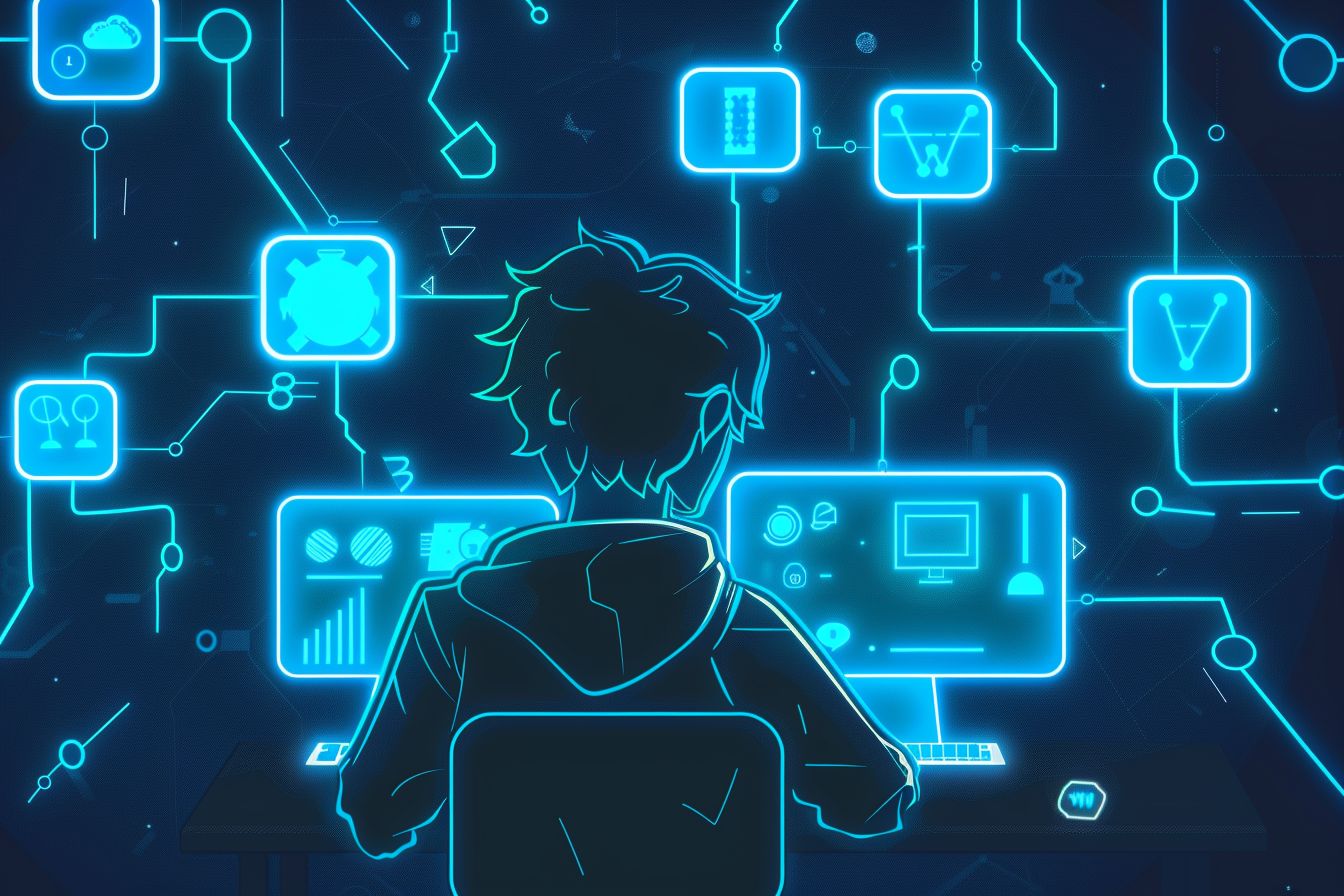
Efficient Data Fetching Patterns with React Query and Suspense
Dive deep into advanced data fetching techniques using React Query and React's Suspense for better performance and code simplicity
Jul 05, 2024 - 10:05 • 4 min read
Transforming Data Fetching with React Query and Suspense
When it comes to managing asynchronous data in React applications, the landscape has become much more sophisticated. With libraries such as React Query and new features like Suspense, we can transform our approach to data fetching into an efficient, streamlined process.
In this post, we'll deploy advanced techniques using React Query combined with React's Suspense to optimize performance and enhance code simplicity. This will cover some intricate aspects often overlooked but crucial for large-scale applications.
Why React Query?
React Query, also known as TanStack Query, offers a plethora of features for complex data fetching scenarios, including caching, synchronization, pagination, and background updates. Its declarative nature fits nicely with React’s component structure, making it a favorite among developers.
// Basic example of React Query fetching
import { useQuery } from 'react-query';
function fetchTodos() {
return fetch('/api/todos').then(res => res.json());
}
function TodoList() {
const { status, data, error } = useQuery('todos', fetchTodos);
if (status === 'loading') return <p>Loading...</p>;
if (status === 'error') return <p>Error: {error.message}</p>;
return (
<ul>
{data.map(todo => (
<li key={todo.id}>{todo.title}</li>
))}
</ul>
);
}
The Power of Suspense
React’s Suspense lets you tune the experience of your application finely. By suspending the rendering of a component tree until a condition is met, usually the resolution of a Promise, you can manage loading states cleanly.
Combine Suspense with React Query to improve user experience and manage async states more efficiently. Let’s look at how to integrate Suspense with React Query.
useTransition
Optimizing with Using useTransition
, we can orchestrate complex UI transitions. It provides a pending state to handle transitions, making your UI responsive and smooth.
import { Suspense, useTransition } from 'react';
function App() {
const [startTransition, isPending] = useTransition();
return (
<div>
{isPending && <Spinner />}
<Suspense fallback={<Loading />}>
<TodoList />
</Suspense>
<button onClick={() => startTransition(() => {/* some state update */})}>
Update state
</button>
</div>
);
}
Combining Suspense with React Query
With React Query, you can specify a suspense: true
option to enable Suspense mode. This way, when the query promise is pending, React will suspend the component tree.
import { QueryClient, QueryClientProvider } from 'react-query';
const queryClient = new QueryClient();
function App() {
return (
<QueryClientProvider client={queryClient}>
<Suspense fallback={<Loading />}>
<TodoList />
</Suspense>
</QueryClientProvider>
);
}
Here's how to configure a query to work with Suspense:
import { useQuery } from 'react-query';
function fetchTodos() {
return fetch('/api/todos').then(res => res.json());
}
function TodoList() {
const { data } = useQuery('todos', fetchTodos, { suspense: true });
return (
<ul>
{data.map(todo => (
<li key={todo.id}>{todo.title}</li>
))}
</ul>
);
}
Advanced Techniques
Prefetching Queries
Prefetching can significantly improve performance by loading data before it's requested. React Query offers queryClient.prefetchQuery
to accomplish this.
import { useEffect } from 'react';
import { useQueryClient } from 'react-query';
function App() {
const queryClient = useQueryClient();
useEffect(() => {
queryClient.prefetchQuery('todos', fetchTodos);
}, []);
return <TodoList />;
}
Invalidate Queries on Mutation
Data often changes due to mutations. React Query caters to this by invalidating queries, ensuring the data stays fresh.
import { useMutation, useQueryClient } from 'react-query';
function TodoForm() {
const queryClient = useQueryClient();
const mutation = useMutation(newTodo => fetch('/api/todos', {
method: 'POST',
body: JSON.stringify(newTodo),
}), {
onSuccess: () => {
queryClient.invalidateQueries('todos');
},
});
return (
<form onSubmit={e => {
e.preventDefault();
mutation.mutate({ title: e.target.elements.title.value });
}}>
<input name="title" />
<button type="submit">Add Todo</button>
</form>
);
}
Paginated and Infinite Queries
Paginated and infinite queries are essential for handling large datasets. React Query simplifies their management.
import { useInfiniteQuery } from 'react-query';
function fetchTodos({ pageParam = 0 }) {
return fetch(`/api/todos?cursor=${pageParam}`).then(res => res.json());
}
function TodoList() {
const { data, fetchNextPage, hasNextPage, isFetchingNextPage } = useInfiniteQuery('todos', fetchTodos, {
getNextPageParam: (lastPage, allPages) => lastPage.nextCursor,
});
return (
<div>
{data.pages.map(page => (
page.todos.map(todo => <p key={todo.id}>{todo.title}</p>)
))}
<button onClick={() => fetchNextPage()} disabled={!hasNextPage || isFetchingNextPage}>
{isFetchingNextPage ? 'Loading more...' : 'Load More'}
</button>
</div>
);
}
Conclusion
By combining React Query’s powerful features with React’s Suspense, we can enhance data fetching in our applications significantly. From handling intricate aspects like caching and synchronization to creating a responsive UI with useTransition
, these tools provide a robust framework to build efficient, scalable applications. Go ahead and integrate these patterns into your projects and experience the seamless data-fetching experience!