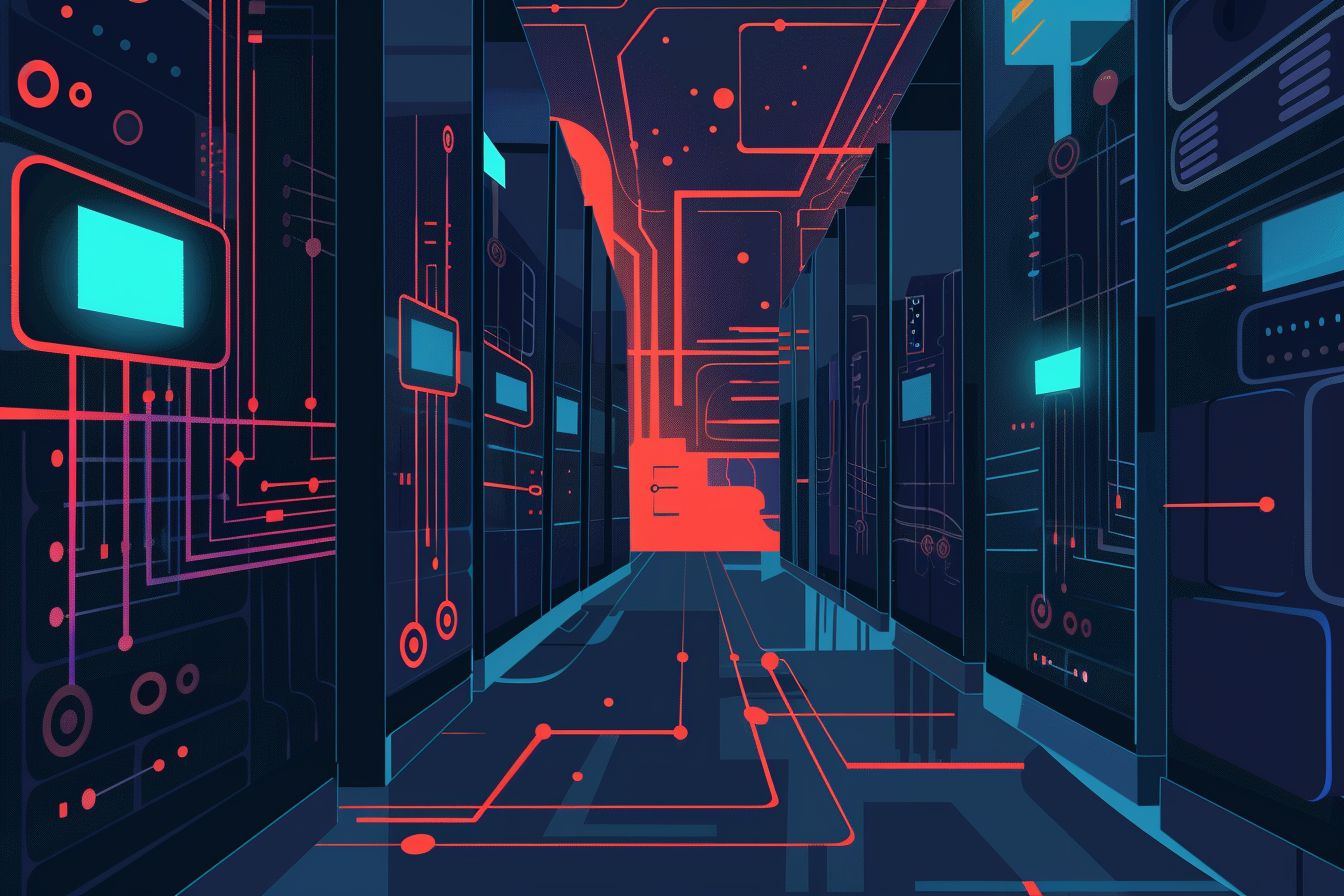
Harnessing the Power of Proxies in JavaScript
Supercharge Your JavaScript Code with Advanced Proxy Techniques
Jul 04, 2024 - 20:22 • 4 min read
Harnessing the Power of Proxies in JavaScript
JavaScript proxies are a powerful feature that allows you to intercept and redefine fundamental operations for objects. This provides opportunities for various advanced techniques, including object property validations, dynamic method injections, performance enhancements, and more. Let's dive deep into how you can harness the power of proxies to supercharge your JavaScript code.
Understanding JavaScript Proxies
Introduced in ECMAScript 2015 (ES6), a proxy object wraps an existing object and allows you to intercept and redefine some operations for that object. The Proxy
object is used to define custom behavior for fundamental operations such as property access, assignment, enumeration, function invocation, etc.
Here’s a basic example of how a proxy works:
const target = {};
const handler = {
get: function(target, property, receiver) {
console.log(`Getting ${property}`);
return target[property];
}
};
const proxy = new Proxy(target, handler);
proxy.name = "JavaScript";
console.log(proxy.name); // Logs: 'Getting name' then 'JavaScript'
In this example, every time we access a property on the proxy, our custom get
function defined in the handler intercepts the operation.
Advanced Proxy Techniques
Proxies can be used to implement various advanced techniques. Let's explore some intriguing applications and their implementation.
Auto-Binding Functions
In JavaScript, managing the scope of this
in functions can be tricky. With proxies, you can automatically bind methods of an object to ensure they have the correct this
context.
function autoBindMethods(obj) {
return new Proxy(obj, {
get(target, property, receiver) {
const value = target[property];
if (typeof value === 'function') {
return value.bind(target);
}
return value;
}
});
}
const obj = {
name: 'Proxied Object',
getName() {
return this.name;
}
};
const proxiedObj = autoBindMethods(obj);
const getName = proxiedObj.getName;
console.log(getName()); // Logs: 'Proxied Object'
Validation Layers
You can use proxies to add validation layers to your objects, ensuring certain rules are followed when properties are set or accessed.
const createValidatedObject = obj => new Proxy(obj, {
set(target, property, value) {
if (typeof value !== 'string') {
throw new TypeError('Property must be a string');
}
target[property] = value;
return true;
}
});
const user = createValidatedObject({});
user.name = 'John'; // Works fine
console.log(user.name); // Logs: 'John'
try {
user.name = 123; // Throws: Property must be a string
} catch (e) {
console.error(e.message);
}
Dynamic Property Addition
Proxies can be used to dynamically create properties on the fly, based on the rules you define.
const createDynamicProperties = (obj, propertyMapper) => new Proxy(obj, {
get(target, property, receiver) {
if (!(property in target) && property in propertyMapper) {
target[property] = propertyMapper[property]();
}
return target[property];
}
});
const dynamicObj = createDynamicProperties({}, {
dynamicProp: () => 'I was dynamically added!'
});
console.log(dynamicObj.dynamicProp); // Logs: 'I was dynamically added!'
Performance Monitoring
Proxies can also be utilized to monitor performance by intercepting and timing function calls.
const createPerformanceMonitor = obj => new Proxy(obj, {
get(target, property, receiver) {
const origMethod = target[property];
if (typeof origMethod === 'function') {
return function (...args) {
console.time(property);
const result = origMethod.apply(this, args);
console.timeEnd(property);
return result;
};
}
return origMethod;
}
});
const objWithMethods = {
slowMethod() {
for (let i = 0; i < 1e6; i++);
},
fastMethod() {
return true;
}
};
const monitoredObj = createPerformanceMonitor(objWithMethods);
monitoredObj.slowMethod(); // Logs: slowMethod: <time taken>
monitoredObj.fastMethod(); // Logs: fastMethod: <time taken>
Best Practices and Considerations
While proxies are incredibly powerful, they should be used judiciously. Here are some best practices and considerations to keep in mind:
Avoid Overhead: Although proxies can elegantly solve many problems, they add a layer of overhead. Use them judiciously in performance-critical paths.
Readability: Proxies can make code behavior non-obvious. Make sure to document the use of proxies well so that other developers (or yourself in the future) understand what is happening.
Compatibility: Proxies are not supported in older environments (e.g., IE11). Ensure you don't rely heavily on proxies in environments where support is not guaranteed.
Conclusion
JavaScript proxies offer a powerful way to add dynamic behavior to your objects. Whether you need to bind methods automatically, validate properties, dynamically add properties, or monitor performance, proxies provide a flexible and elegant solution. However, as with any powerful tool, it's important to use proxies thoughtfully and judiciously to ensure they enhance your code rather than complicate it.