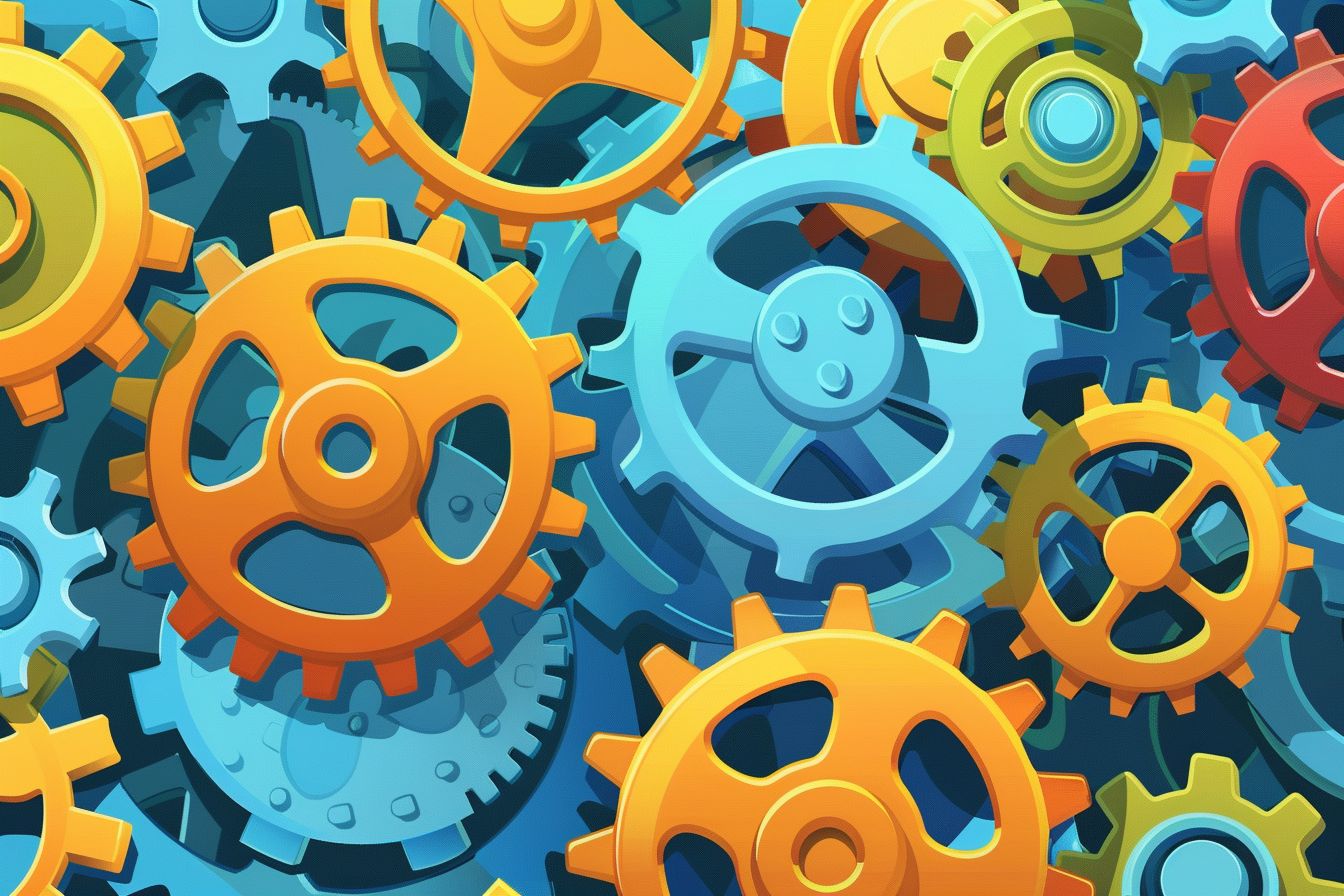
Advanced Optimizations with React Reconciliation
Mastering the inner workings of React's reconciliation process to build high-performance applications
Jul 05, 2024 - 20:40 • 4 min read
Advanced Optimizations with React Reconciliation
Understanding React's reconciliation process is key to optimizing performance in complex applications. Reconciliation is how React updates the DOM to match the virtual DOM. By mastering this process, you can ensure your applications run efficiently and smoothly.
The Basics of Reconciliation
Reconciliation is React's process of diffing the old virtual DOM with the new one and applying the necessary changes to the real DOM. This process is guided by two key principles:
- Minimal Updates: React strives to minimize updates by reusing as much of the existing DOM as possible.
- Predictable Performance: By breaking down updates into small, manageable chunks, React ensures that UI updates remain smooth and performance is predictable.
Let's start by understanding these fundamentals with an example:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return <h1 onClick={() => setCount(count + 1)}>{count}</h1>;
}
In this simple counter component, React updates the DOM efficiently by only changing the text content inside the <h1>
element when the state updates.
Deep Dive into Keys
Keys play a pivotal role in helping React identify which items have changed, are added, or are removed. Using keys properly can prevent React from unncessarily remounting components, which can be costly in terms of performance.
Consider the following list component:
function ItemList({ items }) {
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
By assigning a unique key to each list item based on item.id
, we help React's reconciliation algorithm match old and new elements more accurately.
Avoiding Unnecessary Re-renders
One of the most effective optimizations in React is avoiding unnecessary re-renders. This can be achieved through memoization techniques and ensuring that components only re-render when their props or state change.
React.memo
React.memo
is a higher order component that memoizes functional components. It prevents re-renders if the props have not changed.
const MyComponent = React.memo(function MyComponent(props) {
// Your component code
});
useCallback and useMemo
useCallback
and useMemo
are hooks that cache functions and computed values, respectively, to avoid recalculating them on every render.
import React, { useState, useCallback } from 'react';
function ExpensiveComponent({ onExpensiveOperation }) {
const handleClick = useCallback(() => {
onExpensiveOperation();
}, [onExpensiveOperation]);
return <button onClick={handleClick}>Run</button>;
}
In this example, handleClick
is only recreated if onExpensiveOperation
changes, reducing unnecessary renderings.
Leveraging the Profiler API
React provides the Profiler API to measure the performance of your components. It allows you to record the time each component takes to render, helping identify performance bottlenecks.
Enabling Profiler
You can enable the Profiler in your React components as follows:
import { Profiler } from 'react';
function MyComponent() {
const onRenderCallback = (
id, // the id of the Profiler tree that has just committed
phase, // either "mount" (if the tree just mounted) or "update" (if it re-rendered)
actualDuration, // time spent rendering the committed update
) => {
console.log({ id, phase, actualDuration });
};
return (
<Profiler id="MyComponent" onRender={onRenderCallback}>
<div>...</div>
</Profiler>
);
}
Analyzing Profiler Output
By analyzing the output data from the Profiler, you can pinpoint which components are causing performance issues and optimize them accordingly. Look for components that re-render too frequently or take a long time to render.
Concurrent Mode and Suspense
React's Concurrent Mode and Suspense provide even more powerful tools for optimizing performance by allowing React to interrupt rendering and focus on high-priority updates.
Using Concurrent Mode
Concurrent Mode enables React to work on multiple state updates simultaneously, making your app feel faster and more responsive. Enable it using ReactDOM.createRoot()
.
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
Suspense for Data Fetching
With Suspense, you can improve the user experience by showing loading states for asynchronous operations. Use it with React.lazy
and Suspense
components.
import React, { lazy, Suspense } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
Conclusion
By mastering the intricacies of React's reconciliation process and leveraging advanced optimization techniques, you can build high-performance applications. Remember to use keys effectively, avoid unnecessary re-renders, leverage React's Profiler API, and make use of Concurrent Mode and Suspense for the best performance and user experience.
Happy coding!