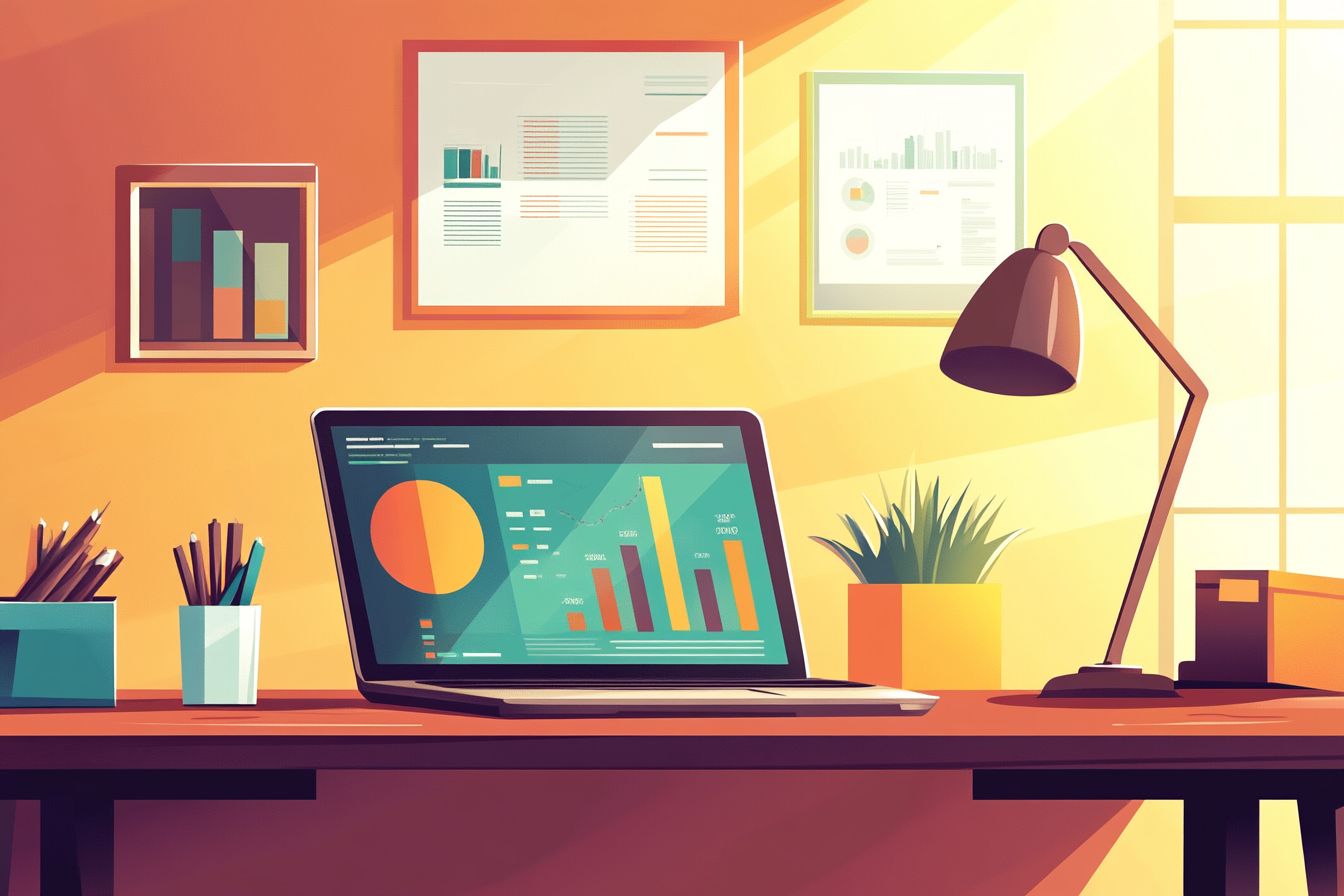
Dynamic Component Generation in React
Unlocking the Power of Dynamic Components for Flexible UI Design
Aug 13, 2024 - 14:32 • 5 min read
Introduction
In the world of React, component reusability is a core tenet that developers strive to achieve. But what happens when you need components that can change dynamically based on user interaction or data? In this blog post, we will explore how to effectively generate dynamic components in React, going beyond just creating static components to embracing a fully dynamic UI.
Understanding Dynamic Components
Dynamic components in React are those that can be created or modified at runtime based on user actions or other stimuli. This goes beyond traditional component props or state; instead, it dives into the heart of React's compositional model.
Several use cases necessitate dynamic components:
- Rendering forms based on backend data.
- Displaying different UI elements based on user preferences.
- Modifying a layout based on responsive design.
React.createElement
Method
The To construct components dynamically, we can use the React.createElement
method. This function allows you to create a React element of a specific type using JavaScript instead of JSX. Here's a simple example:
const DynamicComponent = ({ type, props }) => {
return React.createElement(type, props);
};
In this code snippet, DynamicComponent
takes a type and props as arguments and creates an element of the given type with the specified props at runtime.
Building a Dynamic Form Example
Let's build a practical example of a dynamic form generator that renders input fields based on a configuration object.
const DynamicForm = ({ config }) => {
return (
<form>
{config.map(({ type, name, placeholder }, index) => (
<DynamicField key={index} type={type} name={name} placeholder={placeholder} />
))}
</form>
);
};
const DynamicField = ({ type, name, placeholder }) => {
return <input type={type} name={name} placeholder={placeholder} />;
};
const formConfig = [
{ type: 'text', name: 'username', placeholder: 'Enter your username' },
{ type: 'password', name: 'password', placeholder: 'Enter your password' },
];
<DynamicForm config={formConfig} />
In this example, the DynamicForm
component accepts a config
prop that tells it what fields to render. Each field is represented by the DynamicField
component, which uses the type
, name
, and placeholder
properties to generate an input element.
Handling Dynamic State with Hooks
When dealing with dynamic components, tracking state becomes crucial. With the form example, we can integrate the use of hooks to manage the dynamic values of the inputs:
import React, { useState } from 'react';
const DynamicFormWithState = ({ config }) => {
const [formData, setFormData] = useState({});
const handleChange = (e) => {
const { name, value } = e.target;
setFormData((prev) => ({ ...prev, [name]: value }));
};
return (
<form>
{config.map(({ type, name, placeholder }, index) => (
<DynamicField
key={index}
type={type}
name={name}
placeholder={placeholder}
onChange={handleChange}
/>
))}
</form>
);
};
const DynamicField = ({ type, name, placeholder, onChange }) => {
return <input type={type} name={name} placeholder={placeholder} onChange={onChange} />;
};
Now, every time a user types in an input field, handleChange
updates the formData
state with the current value of the input. This pattern is foundational in forms that require dynamic user input.
Performance Considerations
When building components dynamically, it's essential to consider performance and rendering efficiency. Much like the key
prop in lists, helping React know which items are changed, added, or removed can keep your app performant.
If you’re creating multiple dynamic components, ensure that you handle keys carefully to avoid unnecessary re-renders:
{config.map((field, index) => (
<DynamicField key={`${field.name}-${index}`} {...field} onChange={handleChange} />
))}
This ensures that React can uniquely identify each field based on its name
and index, helping with efficient re-renders.
React.lazy
and Code Splitting
Leveraging Another sophisticated approach is utilizing React.lazy
for dynamic imports and rendering components only when needed. This can optimally reduce the initial loading time of your app:
const LoadableComponent = React.lazy(() => import('./SomeComponent'));
const ParentComponent = () => {
return (
<React.Suspense fallback={<div>Loading...</div>}>
<LoadableComponent />
</React.Suspense>
);
};
This method is quite powerful for large applications where components may not be needed immediately, allowing for effective code-splitting.
Utilizing Render Props for Dynamic UI
Render Props is another powerful pattern for generating dynamic components. This allows developing components that can inject behavior into other components dynamically:
const DynamicComponent = ({ render }) => {
return <div>{render()}</div>;
};
const App = () => {
return (
<DynamicComponent render={() => <h1>Hello Dynamic Component</h1>} />
);
};
In this example, DynamicComponent
accepts a render prop, which allows complete flexibility in what gets rendered.
Best Practices to Consider
When implementing dynamic component generation, consider following best practices:
- Keep Components Small: Create smaller reusable components. It makes it easier to manage complexity.
- Memoization: Use
React.memo
for components that do not require frequent updates, preventing unnecessary re-renders. - Prop Validation: Employ prop-type validation (
prop-types
library) for better maintainability and debugging. - Effective Error Handling: Implement good error boundaries for rendering dynamic components, particularly if they fetch data conditionally.
Conclusion
Dynamic component generation in React reshapes how we think about building UIs. By leveraging techniques like React.createElement
, hooks, and render props, we create flexible, reusable, and efficient components tailored to user needs. As the demand for dynamic applications grows, understanding these patterns primes developers to build safer and more engaging user experiences. Review your component architecture and consider how you can integrate dynamic approaches into your React applications.