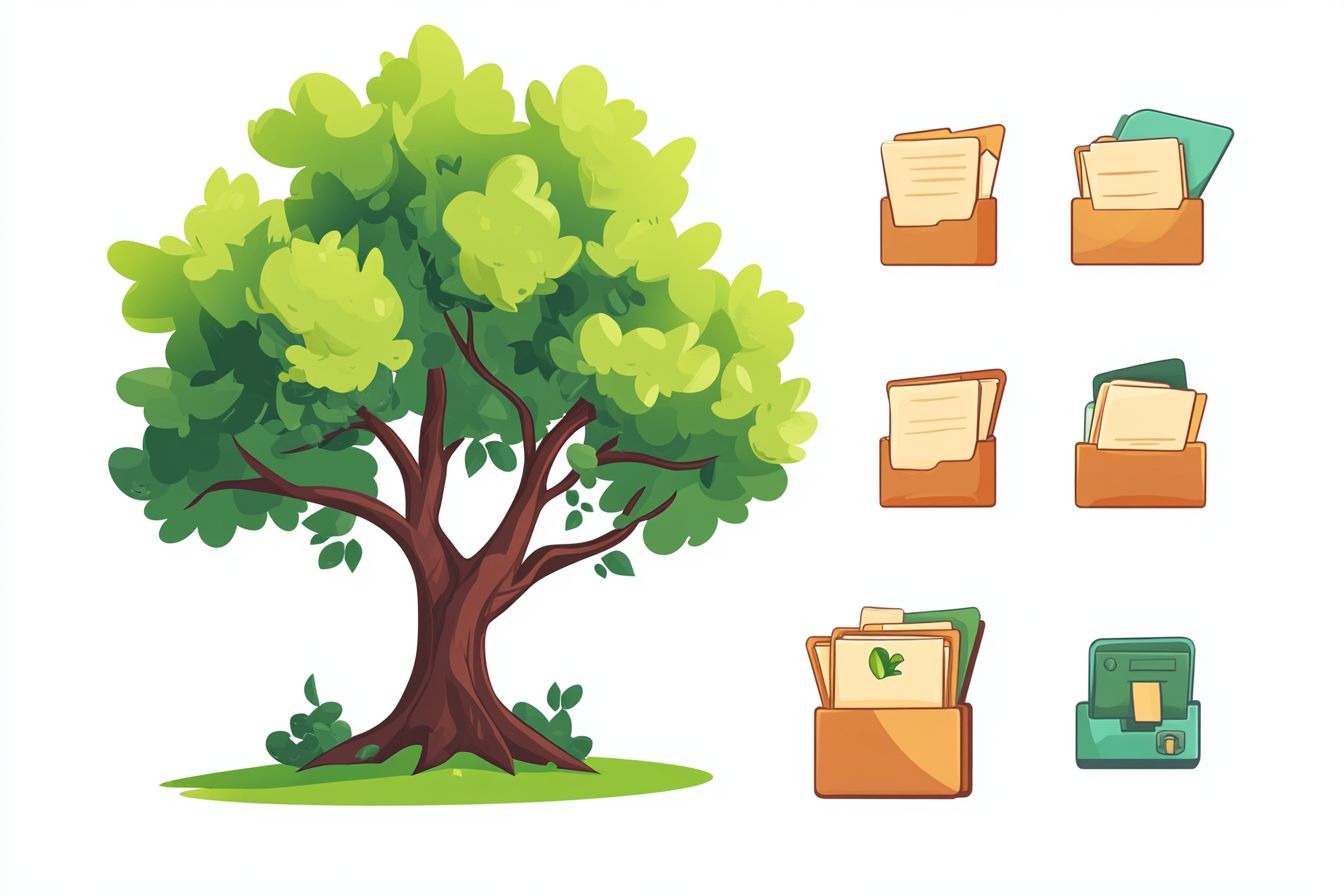
Advanced Recursive Components in React
Building complex UIs with self-referential components and hooks.
Aug 14, 2024 - 10:43 • 4 min read
Understanding recursive components can significantly enhance your skills in React development, allowing you to build intricate UIs with elegance and minimal code. In this post, we will explore advanced techniques for creating recursive components that can be essential in scenarios like tree structures, file explorers, or nested lists.
What are Recursive Components?
Recursive components are components that render themselves based on certain conditions. They are particularly useful in the context of data structures that exhibit hierarchical or self-similar characteristics. In simpler terms, a recursive component can render itself within its own output.
Why Use Recursive Components?
In situations where data is nested, like comments left on a blog post or categories in an e-commerce app, one of the best ways to represent this is through recursive components. They allow for clean and concise rendering logic without repetitive code, making your components scalable and easier to maintain.
Example Use Case
Imagine creating a file directory structure where each folder can contain files and other folders. We can design our components to reflect this hierarchy.
Setting Up the Recursive Component
Let’s create a FileExplorer
component that can recursively render files and folders. The structure of our data might look something like this:
const data = {
name: 'Root',
children: [
{ name: 'Folder1', children: [] },
{ name: 'Folder2', children: [
{ name: 'Subfolder1', children: [] }
] },
{ name: 'File1.txt', children: [] }
]
};
Here is the FileExplorer
component:
import React from 'react';
const FileExplorer = ({ node }) => {
return (
<div>
<div>{node.name}</div>
{node.children && node.children.length > 0 && (
<div style={{ paddingLeft: '20px' }}>
{node.children.map((child, index) => (
<FileExplorer key={index} node={child} />
))}
</div>
)}
</div>
);
};
How it Works
- The
FileExplorer
component accepts anode
prop, which represents either a file or folder. - It displays the name of the file or folder and checks if it has any children.
- If children exist, the component calls itself again to render each child node, creating a recursive effect.
This pattern reduces redundancy and keeps our code clean, instead of implementing separate components for each level of the hierarchy.
Advanced Features
Now, let's consider a few advanced features we can add:
- Dynamic Item Rendering: Use hooks to fetch data asynchronously or handle real-time updates within a file system.
- Animation Effects: Apply animations when folders open or close, adding to the user experience.
- Contextual Interfaces: Use Context API or state management solutions (such as Redux) to manage the state across recursive layers.
Using React Hooks with Recursive Components
When incorporating hooks within recursive components, we need to pay attention to component lifecycles to avoid unintended behaviors. One common use case is tracking and updating expanded states for folders. This can be done using the useState
hook:
const FileExplorer = ({ node }) => {
const [isOpen, setIsOpen] = React.useState(false);
const toggleOpen = () => setIsOpen(!isOpen);
return (
<div>
<div onClick={toggleOpen} style={{ cursor: 'pointer' }}>
{isOpen ? '[-]' : '[+]'} {node.name}
</div>
{isOpen && node.children && node.children.length > 0 && (
<div style={{ paddingLeft: '20px' }}>
{node.children.map((child, index) => (
<FileExplorer key={index} node={child} />
))}
</div>
)}
</div>
);
};
Performance Considerations
While recursive components are elegant, we must consider performance. Heavy recursive rendering might lead to performance issues if not optimized properly. To mitigate this, consider the following techniques:
- Memoization: Utilize
React.memo
oruseMemo
to memoize components when the properties do not change. This way, React can skip re-rendering when appropriate. - Virtualization: For large datasets, tools like
react-window
orreact-virtualized
can help to render only the visible items in a list, greatly enhancing performance.
Conclusion
Recursive components are a powerful pattern in React that can simplify the handling of hierarchical data structures. As you expand your knowledge of React, experimenting with and implementing recursive components will give you a deeper understanding of state management and component design. To further enhance your recursive components, consider integrating advanced techniques like memoization and dynamic rendering based on user interactions.
As with any advanced topics, practice is key. Start simple, gradually incorporate complexity, and you’ll find yourself creating sophisticated interfaces with ease. Happy coding!