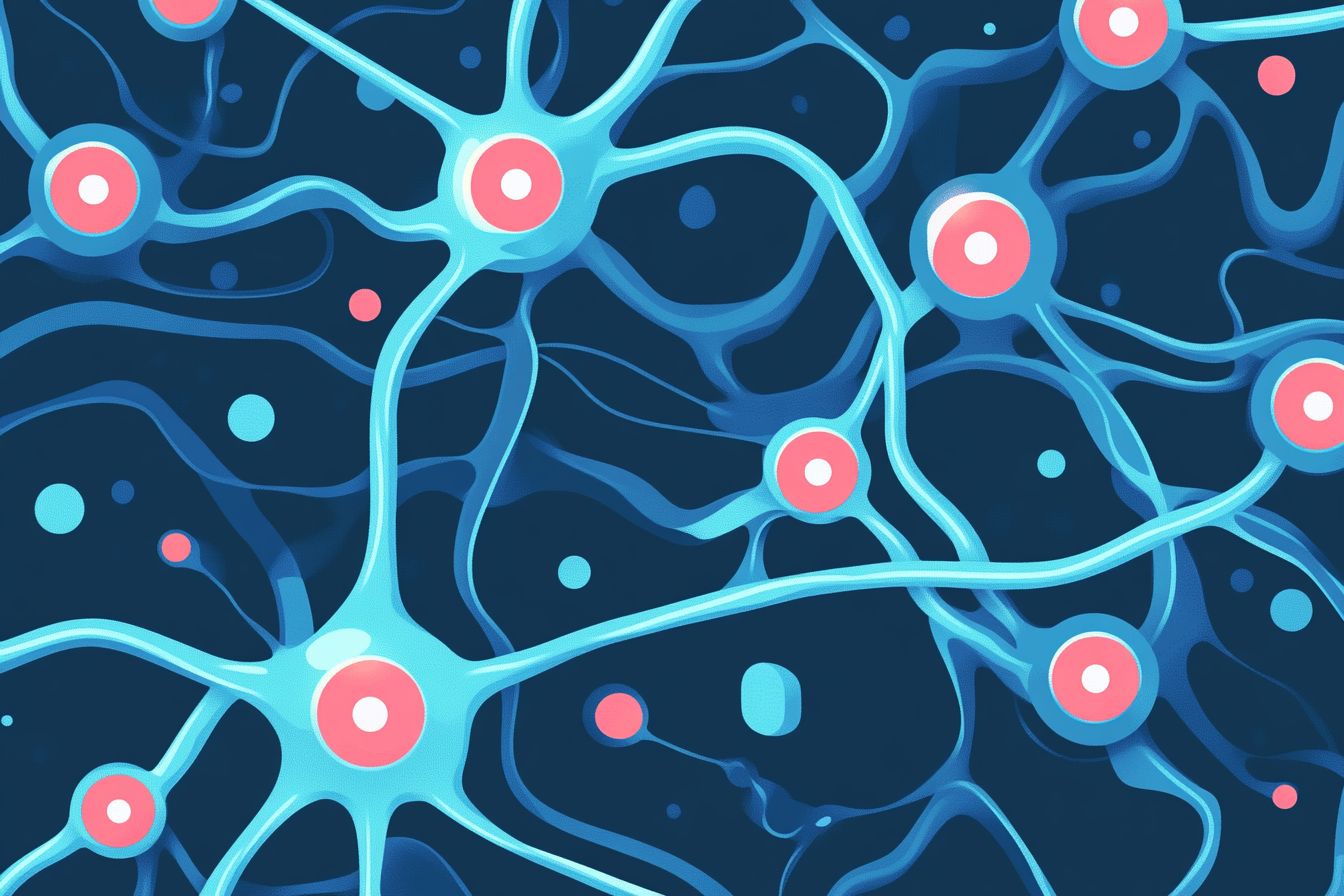
Understanding Reconciliation in React: The Key to Optimizing Component Updates
Dive deep into the inner workings of React's reconciliation process and discover advanced optimization strategies.
Aug 13, 2024 - 12:36 • 5 min read
In recent years, the React ecosystem has grown rapidly, continuously attracting developers seeking to build interactive UIs with minimal effort. Central to this success story is the concept of reconciliation, which is the process React uses to efficiently update the UI based on changes in the underlying data.
Understanding reconciliation is not just about tweaking performance; it opens doors to advanced optimization techniques that can significantly enhance the user experience. In this comprehensive guide, we will explore the nuances of reconciliation, how it impacts our applications, and strategies to leverage it for maximum efficiency.
What is Reconciliation?
Reconciliation in React refers to the algorithm that determines how the UI should update when the state or props change. When a component's state changes, React creates a new Virtual DOM tree and compares it to the previous one, identifying what changes need to be made to reflect the updated state in the real DOM.
This is crucial because manipulating the real DOM is relatively slow, and minimizing direct interactions with it is important for maximizing performance. React's reconciliation process allows it to perform updates in batches, reduce unnecessary rendering, and ensure that only the necessary components are updated.
Here's a simple comparison to illustrate how React handles reconciliation:
function App() {
const [count, setCount] = useState(0);
return (
<div>
<h1>{count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
When the button is clicked, the state changes, leading React to evaluate how the UI should change. The reconciliation process begins, resulting in an efficient update to the DOM.
The Reconciliation Algorithm
React uses a heuristic algorithm called the "Diffing Algorithm" to compare the current and previous Virtual DOM trees. Here are some key points about this algorithm:
- Element Type Comparison: If two elements have different types (e.g., a
div
vs. ah1
), React will remove the old DOM node and replace it with the new one. - Key Prop Efficiency: When rendering lists, providing a unique key prop for each element helps React identify which items have changed, added, or removed. This prevents the entire list from needing to be re-rendered.
- Component-Level Updates: React maintains separate trees for class and functional components. This means that a change in a parent component will trigger reconciliation for its child components, but adjustments in sibling components will not cause unnecessary re-renders.
Here’s a simple example illustrating the correct use of the key prop:
const items = ['Apple', 'Banana', 'Cherry'];
function ItemList() {
return (
<ul>
{items.map(item => <li key={item}>{item}</li>)}
</ul>
);
}
Knowing when to use the key prop effectively allows React to minimize updates through the reconciliation process.
Factors Affecting Reconciliation Performance
While React’s reconciliation is designed for performance, there are certain best practices and pitfalls to keep in mind:
- Avoid Anonymous Functions in Render: Wrapping event handlers directly in the render method can contribute to performance deterioration. Anonymous function creation triggers new renders and interferes with reconciliation.
function App() {
const [count, setCount] = useState(0);
return (
<button onClick={() => setCount(count + 1)}>Increment</button>
);
}
Should be refactored to:
function App() {
const [count, setCount] = useState(0);
const handleIncrement = () => setCount(prev => prev + 1);
return (
<button onClick={handleIncrement}>Increment</button>
);
}
- Component Pure vs. Memoized: Optimize your components with
React.memo
for functional components orPureComponent
for class components. They only re-render when their props change while aiding the reconciliation process.
const MemoizedComponent = React.memo(({ count }) => {
return <div>{count}</div>;
});
- Batch State Updates: Use React’s batching of state updates through the setState function or when using hooks to minimize re-renders.
setState(prev => ({
count: prev.count + 1,
other: newValue,
}));
Advanced Techniques for Optimization
Now that we understand the fundamental principles of reconciliation, let’s explore more advanced techniques that can be utilized for optimal reconciliation performance:
- Code Splitting: Implement code-splitting strategies using
React.lazy
andSuspense
, optimizing how components are loaded and rendered. This enhances app load times and enables a smoother user experience.
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
- Windowing/Lazy Rendering: Utilize libraries like ‘react-window’ or ‘react-virtualized’ for rendering long lists conditionally based on viewport, minimizing the number of DOM nodes and subsequent overhead for the reconciliation process.
import { FixedSizeList as List } from 'react-window';
<List
height={150}
itemCount={1000}
itemSize={35}
width={300}
>
{({ index }) => <div>Row {index}</div>}
</List>;
- Profiling Performance: Utilize the React Profiler tool to measure the performance of your components. It will help you understand where bottlenecks might exist during reconciliation.
import { Profiler } from 'react';
<Profiler id="App" onRender={callback}>
<App />
</Profiler>;
Wrapping Up
Understanding and mastering the reconciliation process in React is crucial in building high-performance applications. This process doesn’t just enhance performance but also positively influences the overall user experience. Tightly coupled techniques such as memoization, key props, and batch updates empower developers to write efficient and declarative code.
As React continues to evolve, keeping abreast of the changes and best practices related to reconciliation will pave the way towards creating blazing-fast applications that engage users effectively. Happy coding!