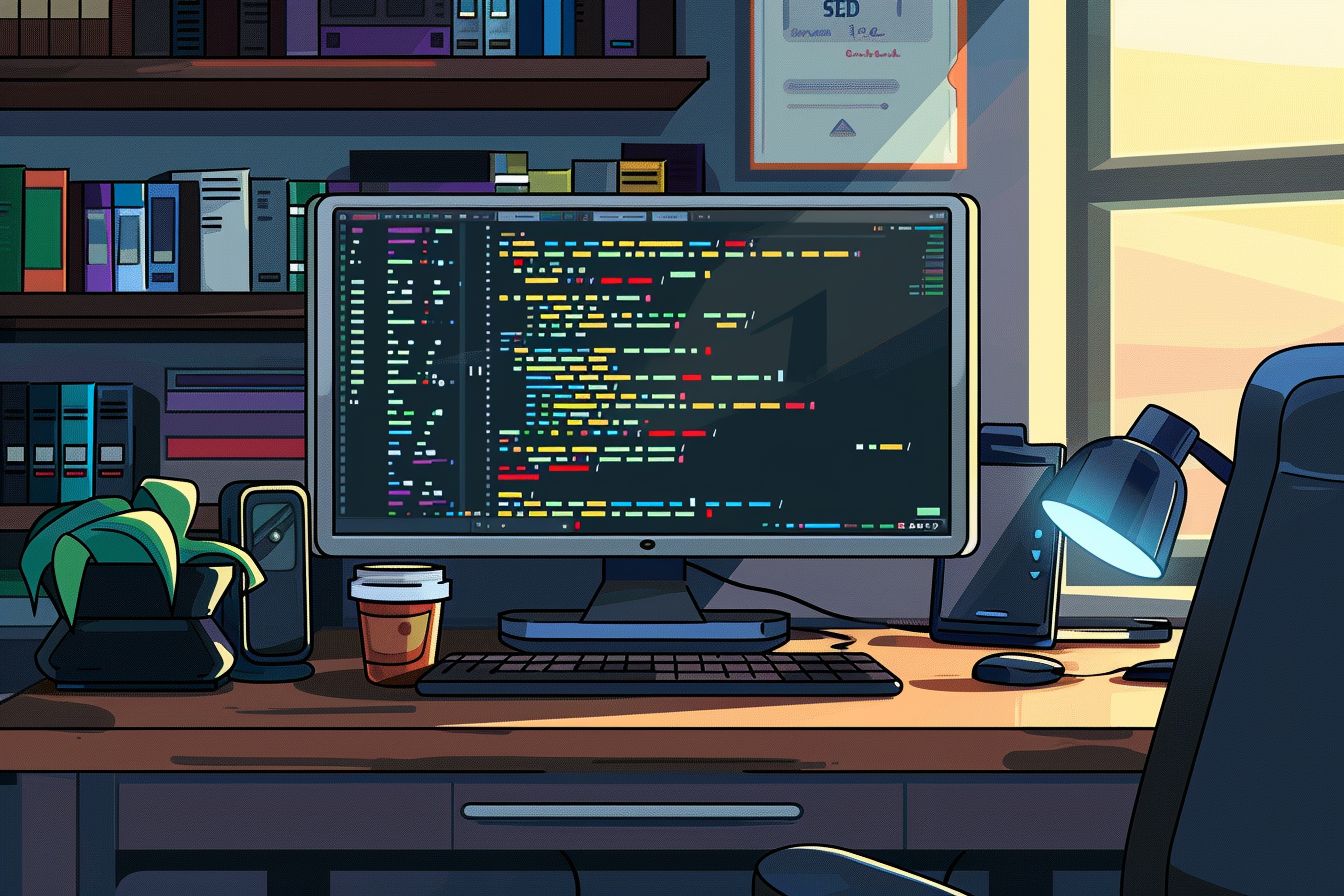
Using Custom Hooks for Efficient Data Fetching in React
Harness the power of custom hooks to simplify data management in your React applications.
Jul 29, 2024 - 14:42 • 5 min read
In modern web development, efficiently managing data fetching operations is crucial for creating high-performance React applications. Traditional approaches using lifecycle methods or plain state management often lead to complex, hard-to-maintain components. Custom hooks provide a powerful abstraction that lets developers encapsulate data fetching logic in a reusable way, optimizing performance while keeping the codebase clean. In this post, we'll explore the concept of creating custom hooks for data fetching in React, and we'll delve into best practices, common patterns, and some performance optimization techniques.
Understanding Custom Hooks
Custom hooks are JavaScript functions whose names start with "use" that can call other hooks. They provide a way to extract component logic so that it can be reused across multiple components. When it comes to data fetching, this means that we can create a dedicated hook to handle all aspects of retrieving data, including error handling and loading states.
Building a Basic Fetch Hook
Let’s start with a simple fetch hook. Here’s how you can create a reusable useFetch
hook for data fetching:
import { useState, useEffect } from 'react';
const useFetch = (url) => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const result = await response.json();
setData(result);
} catch (error) {
setError(error);
} finally {
setLoading(false);
}
};
fetchData();
}, [url]);
return { data, loading, error };
};
How to Use the Hook
You can utilize the useFetch
hook inside your functional components as follows:
import React from 'react';
import useFetch from './useFetch';
const DataDisplay = () => {
const { data, loading, error } = useFetch('https://api.example.com/data');
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h1>Data fetched:</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
};
Handling Multiple Fetch Requests
In scenarios where you need to fetch data from multiple APIs simultaneously, you can extend the useFetch
hook to handle this. It involves managing an array of URLs and fetching each one independently:
const useMultipleFetch = (urls) => {
const [data, setData] = useState([]);
const [loading, setLoading] = useState(true);
const [error, setError] = useState([]);
useEffect(() => {
const fetchData = async (url) => {
try {
const response = await fetch(url);
if (!response.ok) throw new Error(`HTTP error! status: ${response.status}`);
return await response.json();
} catch (err) {
throw err;
}
};
const fetchDataFromUrls = async () => {
setLoading(true);
const results = [];
for (const url of urls) {
try {
results.push(await fetchData(url));
} catch (err) {
setError((prev) => [...prev, err.message]);
}
}
setData(results);
setLoading(false);
};
fetchDataFromUrls();
}, [urls]);
return { data, loading, error };
};
Performance Optimization Techniques
When setting up data fetching in your React applications, performance is of utmost importance. Below are some strategies to optimize data fetching using custom hooks:
1. Debouncing Fetch Requests
If you’re dealing with user input (like search), consider debouncing the API calls to minimize unnecessary fetch requests:
const useDebouncedFetch = (url, delay) => {
const [debouncedUrl, setDebouncedUrl] = useState(url);
useEffect(() => {
const handler = setTimeout(() => {
setDebouncedUrl(url);
}, delay);
return () => {
clearTimeout(handler);
};
}, [url, delay]);
return useFetch(debouncedUrl);
};
2. Caching Responses
Utilize caching strategies (with tools like react-query
or a simple local state) to avoid fetching the same data repeatedly:
const dataCache = new Map();
const useCachedFetch = (url) => {
const [data, setData] = useState(dataCache.get(url) || null);
const [loading, setLoading] = useState(!data);
const [error, setError] = useState(null);
useEffect(() => {
if (data) return;
const fetchData = async () => {
setLoading(true);
try {
const response = await fetch(url);
const result = await response.json();
dataCache.set(url, result);
setData(result);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchData();
}, [url, data]);
return { data, loading, error };
};
Error Handling Strategies
Proper error management not only improves user experience but also aids in debugging. Utilize the error state from the hook efficiently:
const { error } = useFetch('https://api.example.com/data');
if (error) {
console.error('Data Fetch Error: ', error);
// Display a user-friendly error message
}
Conclusion
Custom hooks present an elegant approach to handling data fetching in React applications. They encourage code reuse, promote cleanliness, and enhance performance through advanced patterns and practices. By creating tailored hooks for specific data needs, you can significantly improve code maintainability and reduce boilerplate code. As you explore more about React's hooks, consider the potential of combining them with libraries like react-query
for even finer control.
In this post, we've reviewed the fundamentals of creating a fetch hook, discussed advanced patterns for multiple requests, performance optimizations, and error handling strategies. As the React ecosystem continues to evolve, custom hooks remain a core part of building elegant, maintainable applications.
With this understanding of custom hooks for data fetching, you can now create a cleaner, more efficient codebase, and provide a better experience for users by minimizing loading times and improving error handling.