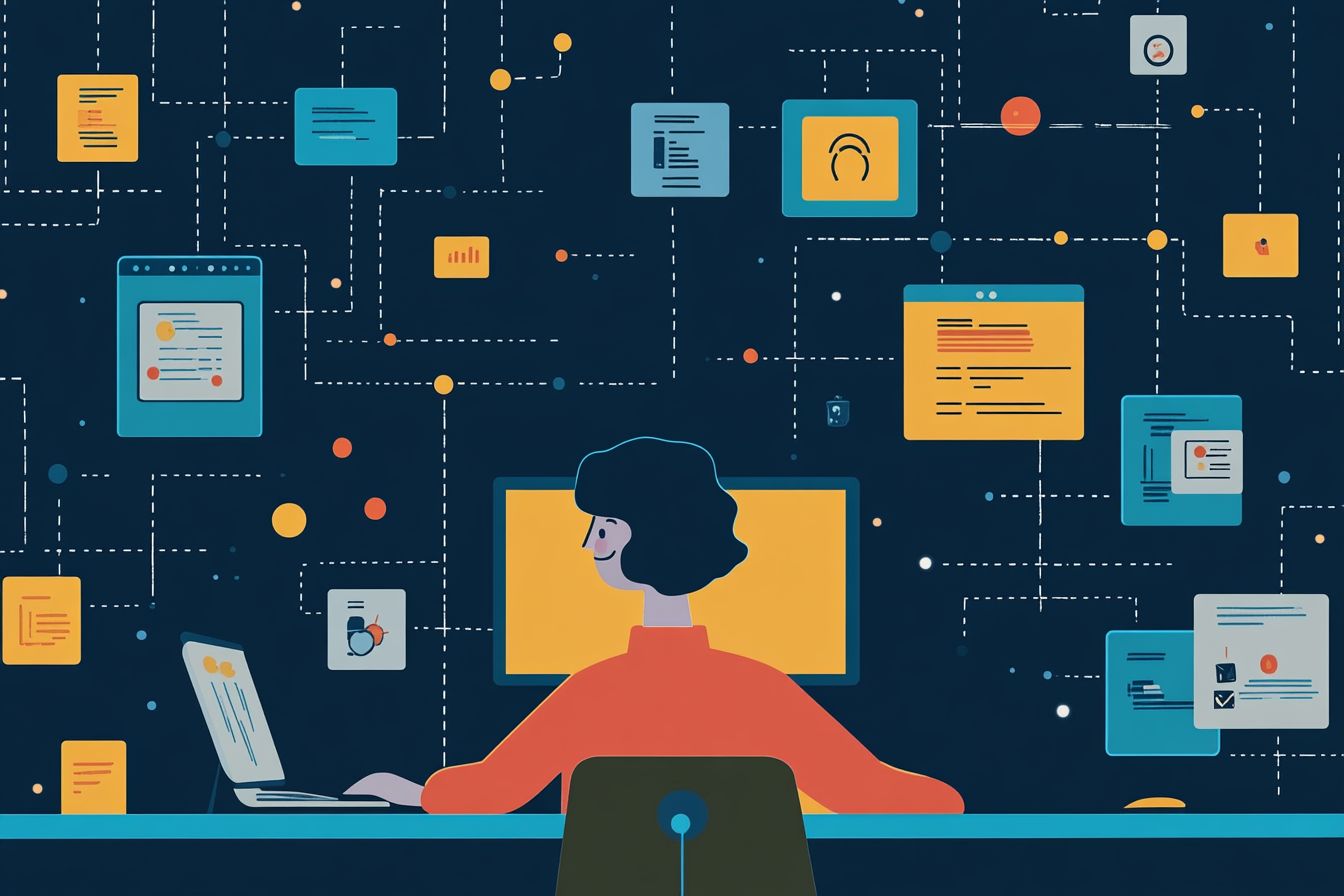
Understanding React's Unique Key Prop
Master the key prop to optimize rendering and performance in your React applications.
Aug 12, 2024 - 13:34 • 4 min read
Understanding React's Unique Key Prop
In the world of React, the key
prop is a fascinating yet often misunderstood concept. Developers frequently overlook the importance of how keys influence re-rendering, reconciliation, and performance optimization. This post delves into advanced use cases and best practices for effectively utilizing the key
prop in React.
What is the Key Prop?
The key
prop is a special attribute in React used to identify uniquely elements in a list. When rendering lists of components, a key provides a way for React to distinguish between each item, facilitating a more efficient reconciliation process during updates.
Here's a simple example:
const items = ['apple', 'banana', 'orange'];
const MyList = () => (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
In this example, we use the array index as the key. While this may seem straightforward, it can lead to performance issues and unexpected behaviors when items are reordered, added, or removed from the list.
Why Keys Matter
React uses keys to identify which items have changed, are added, or are removed. This helps optimize rendering. By providing a stable identity for each component, React minimizes the number of updates required, significantly enhancing performance. Consider this scenario:
const App = () => {
const [items, setItems] = useState(['apple', 'banana', 'orange']);
const removeItem = (item) => {
setItems(items.filter(i => i !== item));
};
return (
<ul>
{items.map(item => (
<li key={item} onClick={() => removeItem(item)}>{item}</li>
))}
</ul>
);
};
Using the item itself as a key ensures that the click-to-remove functionality works as expected without any issues related to index-based keys.
Common Pitfalls
- Using Index as Key: When the list order changes, React might incorrectly apply changes leading to incorrect UI updates.
- Duplicate Keys: Two elements with the same key can cause issues where changes in one element may inadvertently affect another.
- Static vs Dynamic Lists: For dynamic lists that may change in structure, prefer stable identities (such as database IDs).
Best Practices for Keys
- Use Unique IDs: Always opt for unique identifiers from your data source whenever possible:
const MyList = ({ items }) => ( <ul> {items.map(item => ( <li key={item.id}>{item.name}</li> ))} </ul> );
- Avoid Index as Key: Reserve index keys for static lists that will not change over time.
- Ensure Keys Are Predictable: Keys should remain consistent between renders to facilitate efficient updates.
- Nested Lists: When dealing with nested lists, ensure keys for each level are handled appropriately.
Leveraging Functional Updates
React encourages functional updates when updating state. This is particularly useful when maintaining a reference to item identifiers:
const [list, setList] = useState([
{ id: 1, name: 'Apple' },
{ id: 2, name: 'Banana' }
]);
const updateItem = (id) => {
setList(prevList =>
prevList.map(item => (item.id === id ? {...item, renamed: true} : item))
);
};
In this manner, the operation relies on the current state to avoid any stale closures tied to the list
state directly.
The Impact of Keys on Performance
Understanding how keys affect rendering performance is crucial. Nascent React devs may not be aware that optimizing key usage can significantly impact unnecessary renders:
- Correct Keys Reduce Remounts: Utilizing proper keys minimizes the need for React to remount elements during state updates.
- Profiling with React Profiler: Developers should regularly profile their components to observe how key changes impact performance metrics.
Testing React Key Implementation
When implementing keys, testing often helps ensure correctness. Consider using React Testing Library
or Jest
to validate key functionality within lists:
test('List items render with correct keys', () => {
const { getByText } = render(<MyList items={[{id: 1, name: 'apple'}, {id: 2, name: 'banana'}]} />);
expect(getByText('apple')).toBeInTheDocument();
expect(getByText('banana')).toBeInTheDocument();
});
Conclusion
The key
prop is a powerful feature facilitating React's reconciliation process. By understanding its implications, developers create more efficient and error-free applications. As you design your application, keep in mind the best practices discussed and continually assess how key usage shapes your component's performance. With appropriate implementation of keys, your React applications will not only perform better but become increasingly resilient to future changes.
Midjourney Prompt
Create a cartoon representation of a person happily using a computer, surrounded by colorful icons representing data flow, lists, and optimizations in a simple 2D cartoon style.