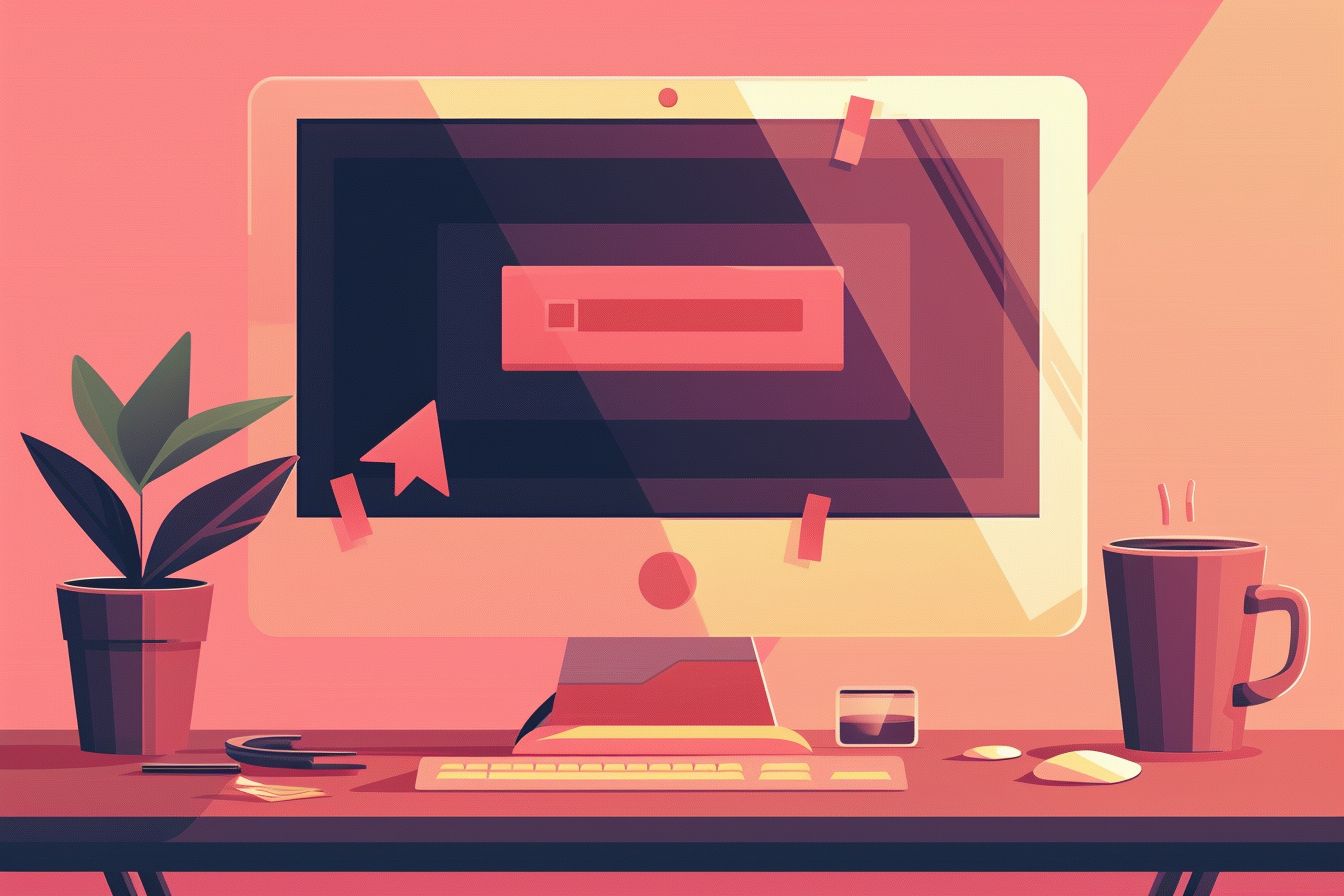
Advanced Patterns with React Error Boundaries
Taking Error Handling to the Next Level
Jul 04, 2024 - 12:58 • 4 min read
Error handling in React applications can often become a tricky affair, especially when dealing with unpredictable runtime errors. React provides a special component called Error Boundary to handle errors gracefully in the UI. However, many developers only scratch the surface of what Error Boundaries can achieve. This post will dive deep into advanced patterns with React Error Boundaries, covering everything from conditional error handling to retry mechanisms and integration with popular libraries.
The Basics of Error Boundaries
React Error Boundaries were introduced in React 16 to catch JavaScript errors in a part of the UI and display a fallback UI. A simple example of an Error Boundary looks like this:
import React, { Component } from 'react';
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// You can also log the error to an error reporting service
console.log(error, errorInfo);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
export default ErrorBoundary;
To use it, simply wrap it around any component that might throw an error during rendering:
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
Conditional Error Handling
In some cases, you may want to handle errors conditionally, depending on the type of error or the context in which it occurred. You can achieve this by extending the getDerivedStateFromError
and componentDidCatch
methods.
For example, if you want to handle network errors differently, you can do something like this:
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false, errorType: null };
}
static getDerivedStateFromError(error) {
// Check error type and update state accordingly
if (error.message.includes('Network Error')) {
return { hasError: true, errorType: 'network' };
}
return { hasError: true, errorType: 'general' };
}
componentDidCatch(error, errorInfo) {
// You can also log the error to an error reporting service
console.log(error, errorInfo);
}
render() {
if (this.state.hasError) {
if (this.state.errorType === 'network') {
return <h1>Network Error: Please check your connection.</h1>;
}
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
Retry Mechanisms
Another advanced pattern involves implementing retry mechanisms for certain types of errors, such as transient network errors. You can extend the Error Boundary to include a retry button and logic to reset the state:
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false, errorType: null };
}
static getDerivedStateFromError(error) {
if (error.message.includes('Network Error')) {
return { hasError: true, errorType: 'network' };
}
return { hasError: true, errorType: 'general' };
}
componentDidCatch(error, errorInfo) {
console.log(error, errorInfo);
}
retry = () => {
this.setState({ hasError: false, errorType: null });
};
render() {
if (this.state.hasError) {
return (
<div>
<h1>{this.state.errorType === 'network' ? 'Network Error: Please check your connection.' : 'Something went wrong.'}</h1>
<button onClick={this.retry}>Retry</button>
</div>
);
}
return this.props.children;
}
}
Integration with Popular Libraries
Error boundaries can be integrated with popular libraries like Sentry or LogRocket for advanced error reporting. Here’s how you can integrate Sentry with an Error Boundary:
import * as Sentry from '@sentry/react';
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
Sentry.captureException(error, { extra: errorInfo });
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
Improving User Experience
Beyond just displaying a generic error message, you can enhance user experience by providing more context or actions. For instance, you can show a detailed error message or provide links to relevant support resources.
render() {
if (this.state.hasError) {
return (
<div>
<h1>Something went wrong.</h1>
<p>{this.state.errorType === 'network' ? 'Please check your connection.' : 'The application encountered an unexpected error. Please try again later.'}</p>
<a href="/support">Contact Support</a>
</div>
);
}
return this.props.children;
}
Error Logging and Analysis
To get the most out of Error Boundaries, it’s crucial to log and analyze errors effectively. Tools like Sentry and LogRocket provide dashboards and insights that can help you understand the root cause of errors and prioritize fixes.
Wrapping Up
Advanced patterns with React Error Boundaries can significantly enhance the reliability and user experience of your applications. By implementing conditional error handling, retry mechanisms, and integrating with error reporting tools, you can ensure that your application gracefully handles unexpected errors and provides a better user experience.
Experiment with these patterns and see how they can fit into your projects. Happy coding!