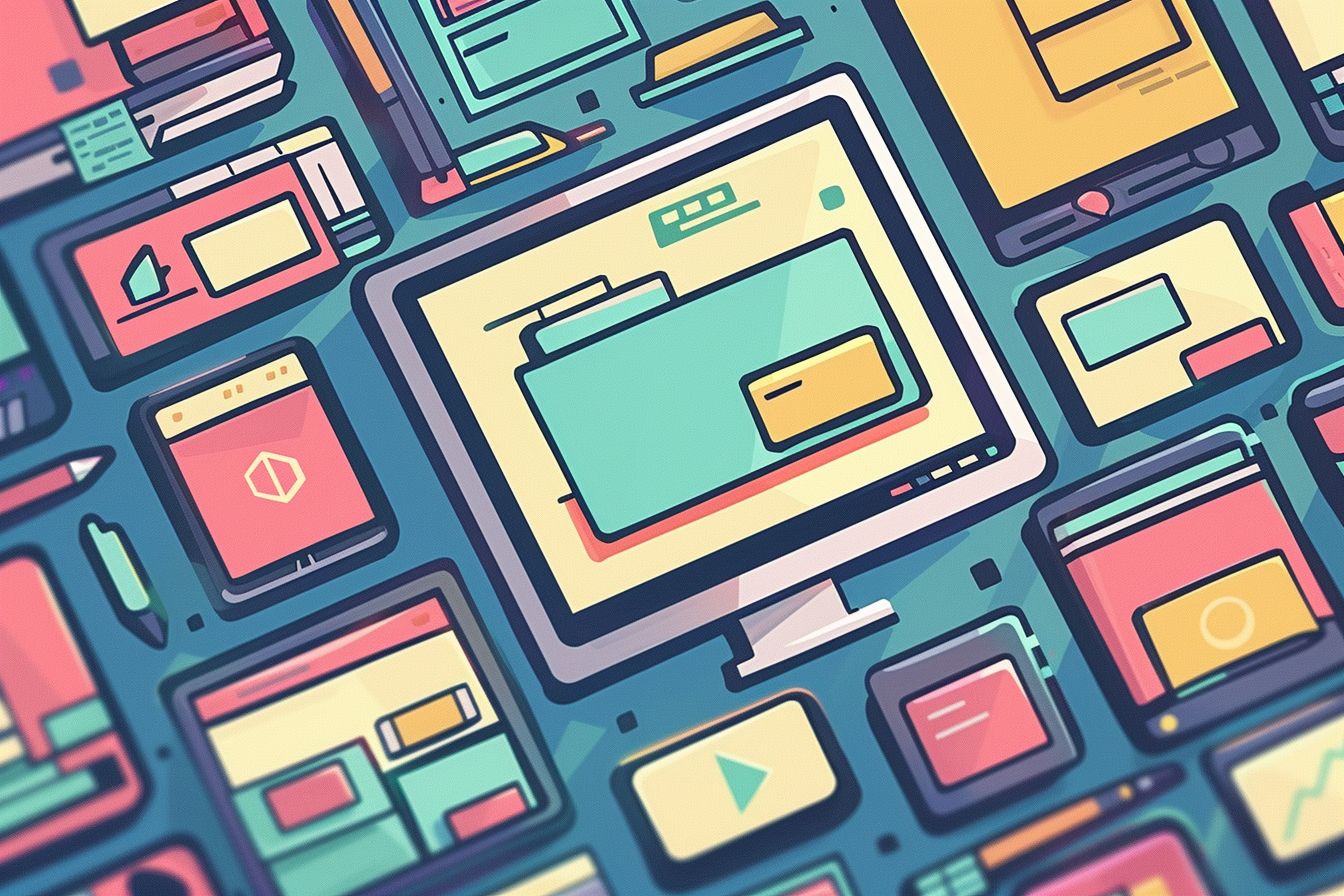
Harnessing the Power of Web Workers in React
Maximizing Efficiency with Background Processing
Jun 28, 2024 - 23:02 • 5 min read
Harnessing the Power of Web Workers in React
Web Workers have been part of the web landscape for quite some time, but their adoption in React projects has seen a steady rise, especially with the need for optimized and seamless user experiences. Web Workers enable us to run scripts in background threads, freeing up the main thread to keep our UI responsive. Let's dive into how you can leverage Web Workers in React applications.
Understanding Web Workers
Web Workers provide a simple means for web applications to run scripts in background threads. This is particularly useful for running heavy computations or IO-intensive tasks asynchronously without affecting the main execution thread.
Basic Structure
A web worker script can be created and executed independently of the main thread. Here's an example of a basic Web Worker script:
// worker.js
self.addEventListener("message", function (e) {
const result = longRunningTask(e.data);
self.postMessage(result);
}, false);
function longRunningTask(data) {
// Perform some long running operations
return data * 2;
}
To use this Web Worker in our main JavaScript file, we would instantiate it and set up message passing:
// main.js
const worker = new Worker("worker.js");
worker.postMessage(10);
worker.onmessage = function (e) {
console.log("Result: ", e.data);
};
Integrating Web Workers with React
Now that we understand the basics, let's integrate Web Workers in a React application to handle a computationally intensive task.
Setting Up the Worker
First, let's create a worker script that will handle our intensive task. Save it as worker.js
:
// worker.js
self.addEventListener("message", function (e) {
// Simulate a heavy task
const result = e.data.map(item => item * 2);
self.postMessage(result);
}, false);
Creating the React Component
We need to integrate this worker into our React component. Here's a simple setup where we leverage the Web Worker to process a large array of numbers:
// App.js
import React, { useState, useEffect } from "react";
// Assuming the worker is compiled and available via worker-loader
import Worker from "./worker.js";
const App = () => {
const [inputArray, setInputArray] = useState([]);
const [outputArray, setOutputArray] = useState([]);
useEffect(() => {
if (inputArray.length) {
const worker = new Worker();
worker.postMessage(inputArray);
worker.onmessage = function (e) {
setOutputArray(e.data);
};
return () => worker.terminate();
}
}, [inputArray]);
const handleClick = () => {
const arr = Array.from({ length: 1000000 }, (_, i) => i + 1);
setInputArray(arr);
};
return (
<div>
<button onClick={handleClick}>Process Array</button>
<div>Output Length: {outputArray.length}</div>
</div>
);
};
export default App;
This component sets up a Web Worker to process an array of one million numbers, doubling each element. Note that the worker is terminated after use to free up resources.
Handling Web Worker Communication Efficiently
Managing multiple messages and handling errors gracefully is crucial for robust Web Worker integration. Here's an improved version of our worker setup in React:
// App.js
import React, { useState, useEffect, useCallback } from "react";
import Worker from "./worker.js";
const useWorker = (inputArray) => {
const [result, setResult] = useState(null);
const [error, setError] = useState(null);
useEffect(() => {
if (inputArray.length) {
const worker = new Worker();
worker.postMessage(inputArray);
worker.onmessage = function (e) {
setResult(e.data);
};
worker.onerror = function (err) {
setError(err.message);
};
return () => worker.terminate();
}
}, [inputArray]);
return { result, error };
};
const App = () => {
const [inputArray, setInputArray] = useState([]);
const { result, error } = useWorker(inputArray);
const handleClick = () => {
const arr = Array.from({ length: 1000000 }, (_, i) => i + 1);
setInputArray(arr);
};
return (
<div>
<button onClick={handleClick}>Process Array</button>
{error && <div>Error: {error}</div>}
{result && <div>Output Length: {result.length}</div>}
</div>
);
};
export default App;
In this version, we encapsulate the Web Worker logic within a custom hook, useWorker
. This custom hook not only handles the messaging but also manages error handling, making the React component cleaner and more maintainable.
Optimizing Performance
While Web Workers significantly improve performance for extensive computations, there are a few best practices to consider:
Use Shared Memory Wisely: Web Workers operate in a different context. Avoid passing large objects directly; use transferable objects for better performance.
Avoid Overhead: Initialize a single Web Worker for recurring tasks rather than spawning a new one each time, which can be resource-intensive.
Error Handling: Always set up error handling to catch and manage unexpected behavior in worker scripts.
Consider Pooling: For tasks requiring multiple workers, consider using a worker pool. This ensures efficient resource management and prevents overwhelming the browser's capacity.
Real-World Applications
Web Workers are versatile and can be applied in various scenarios:
1. Real-Time Data Processing:
For applications that require real-time data manipulation, such as live financial dashboards or gaming apps, Web Workers ensure the main thread remains responsive.
2. Image and Video Processing:
Performing operations such as filters or transformations on large media files can be offloaded to Web Workers to maintain a smooth user experience.
3. Complex Calculations:
Scientific applications, simulations, or any domain requiring heavy computational tasks can benefit from Web Workers, allowing the user interface to remain snappy and responsive.
Navigating Limitations
Despite their advantages, Web Workers come with specific limitations:
No DOM Access: Web Workers cannot manipulate the DOM directly. They must communicate with the main thread to perform any UI updates.
Security Constraints: Workers are subject to the same-origin policy, which can restrict cross-domain requests.
Browser Compatibility: While most modern browsers support Web Workers, it's essential to check for compatibility and provide fallbacks for unsupported environments.
Conclusion
Web Workers offer a powerful tool for optimizing performance in React applications. By offloading intensive tasks to background threads, you can ensure a seamless, responsive user experience. Integrating Web Workers might seem complex initially, but with careful planning and implementation, the benefits greatly outweigh the initial setup efforts. As always, consider the specific needs of your project and leverage Web Workers to keep your React applications running smoothly.
Happy coding!