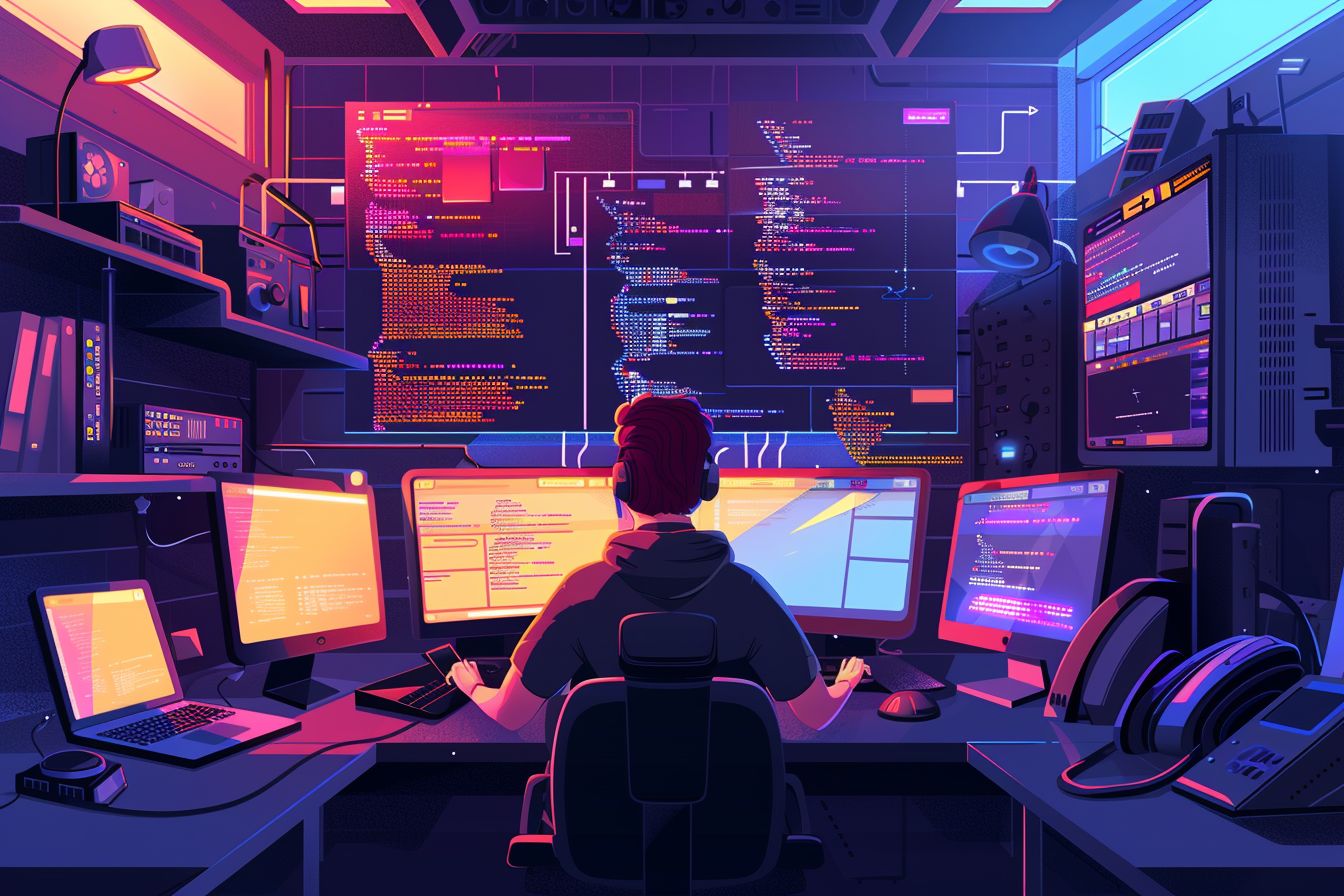
Rethinking CSS-in-JS: Advanced Techniques with Styled Components in React
Unlock the full potential of styled-components by mastering lesser-known yet powerful techniques.
Jun 30, 2024 - 15:54 • 3 min read
Web developers have long been debating the best way to manage styles in React applications. One approach that's popular these days is CSS-in-JS, and among the various libraries available, styled-components
has garnered significant attention. However, many developers only scratch the surface of its capabilities. In this post, we're diving deep into some advanced techniques that you can use to leverage styled-components
to its fullest potential.
Table of Contents
- The Basics Revisited
- Dynamic Styles with Props
- Theming with ThemeProvider
- Styled Component Inheritance
- Global Styles with injectGlobal
- Extending Styles by Overriding
- Optimizing Performance with
as
prop
The Basics Revisited
Before we delve into the more advanced features, let's briefly revisit the basics for those unfamiliar with styled-components
.
Example: Basic Usage
import styled from 'styled-components';
const Button = styled.button`
background: palevioletred;
border-radius: 3px;
border: none;
color: white;
`;
export default Button;
Dynamic Styles with Props
One of the primary reasons developers choose styled-components
is its support for dynamic styling through props.
Example: Dynamic Styles
const Button = styled.button`
background: ${props => props.primary ? 'palevioletred' : 'white'};
color: ${props => props.primary ? 'white' : 'palevioletred'};
`;
<Button primary>Primary Button</Button>
<Button>Default Button</Button>
By utilizing props, you can make any CSS property dynamic, enabling more flexible and reusable components.
Theming with ThemeProvider
If you've ever had to implement dark mode or support multiple themes, you'll love the ThemeProvider
utility in styled-components
.
Example: ThemeProvider
import { ThemeProvider } from 'styled-components';
const theme = {
primary: 'palevioletred',
secondary: 'white',
};
const Button = styled.button`
background: ${props => props.theme.primary};
color: ${props => props.theme.secondary};
`;
<ThemeProvider theme={theme}>
<Button>Button with Theme</Button>
</ThemeProvider>
With ThemeProvider
, you can manage complex theme structures and make your components fully theme-aware.
Styled Component Inheritance
Inheritance in styled-components
can be achieved through styled extensions. This allows you to create a base component and extend it to change styles.
Example: Styled Inheritance
const Button = styled.button`
background: palevioletred;
color: white;
`;
const LargeButton = styled(Button)`
font-size: 20px;
`;
This way, LargeButton
inherits all styles from Button
but also allows for modifications.
Global Styles with injectGlobal
Sometimes you need to inject global styles directly into your application. styled-components
offers the injectGlobal
utility for this purpose.
Example: injectGlobal
import { createGlobalStyle } from 'styled-components';
const GlobalStyle = createGlobalStyle`
body {
margin: 0;
padding: 0;
font-family: 'Arial, sans-serif';
}
`;
<GlobalStyle />
createGlobalStyle
enables you to define and inject global styles conveniently.
Extending Styles by Overriding
Style extension isn't only limited to inheritance. You can override styles on-the-fly by simply nesting the styled component within another styled component.
Example: Overriding Styles
const Button = styled.button`
background: palevioletred;
color: white;
`;
const SpecialButton = styled(Button)`
background: lightgreen;
border: 2px solid green;
`;
SpecialButton
here will have the base styles of Button
but with added styles.
as
prop
Optimizing Performance with Last but not least, styled-components
provides an as
prop that allows you to change the rendered element type dynamically.
as
prop
Example: export const Button = styled.button`
background: palevioletred;
border-radius: 3px;
`;
<Button as="a" href="#">Link styled as Button</Button>
This can improve performance and simplify component structures substantially.
By understanding these advanced techniques, you can become more proficient in using styled-components
, unlocking its full potential and creating more flexible, maintainable codebases.