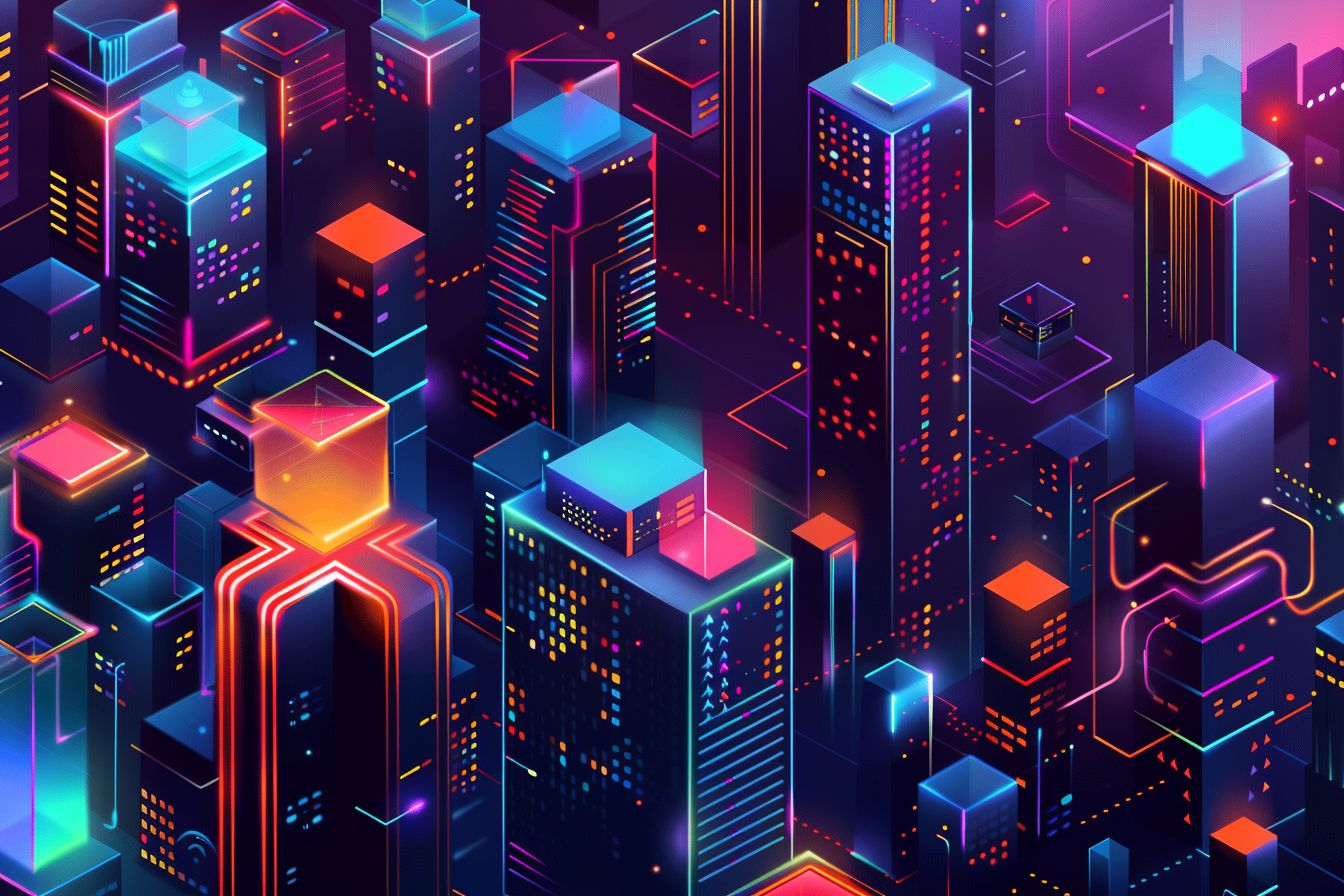
Unlocking the Full Potential of ES Module Import Statements for Advanced Code Splitting
A Deep Dive into Dynamic Imports and Lazy Loading in React
Jul 04, 2024 - 01:23 • 5 min read
Unlocking the Full Potential of ES Module Import Statements for Advanced Code Splitting
In the ever-evolving world of web development, efficient code management is crucial for optimizing performance and user experience. Among the many techniques available to developers, code splitting and lazy loading are pivotal for reducing initial load times and improving overall application scalability. This article delves into advanced use cases of ES module import statements, dynamic imports, and lazy loading in React.
Why Code Splitting and Lazy Loading Matter
Before diving into the technical details, let's recap why code splitting and lazy loading are important:
- Improved Performance: By splitting code into smaller bundles, you ensure that the browser only downloads what's necessary for the current view, leading to faster load times.
- Optimized Resource Use: Dynamic imports allow resources to be loaded only when they are needed, reducing unnecessary bandwidth consumption.
- Enhanced User Experience: Faster load times and smoother interactions result in a more seamless and enjoyable user experience.
Basics of ES Module Import Statements
ES module import statements have become the standard for modular JavaScript. They allow you to import JavaScript functions, objects, or primitives that have been exported from another module. Here's a quick refresher on the syntax:
import { myFunction } from './myModule';
While this static import approach works well for many scenarios, it lacks the flexibility needed for advanced code splitting.
Dynamic Imports in ES Modules
To unlock the full potential of code splitting, we need to understand dynamic imports. Unlike static imports, dynamic imports are executed at runtime and return a promise. This enables on-demand loading of modules:
const module = await import('./myModule');
const myFunction = module.myFunction;
Dynamic imports are particularly useful in React applications that leverage components, as they can be loaded asynchronously.
Code Splitting in React
React provides built-in support for code splitting through dynamic imports and the React.lazy
function. This approach allows you to split the code at the component level:
import React, { lazy, Suspense } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function MyApp() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
The Suspense
component is used to wrap the lazy-loaded component, displaying a fallback UI while the component is being loaded.
Advanced Code Splitting Techniques
Route-Based Code Splitting
In larger applications, route-based code splitting can significantly reduce initial load times. React Router makes this process straightforward. Instead of importing all route components statically, use React.lazy
to load them dynamically:
import React, { lazy, Suspense } from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
const Home = lazy(() => import('./Home'));
const About = lazy(() => import('./About'));
function App() {
return (
<Router>
<Suspense fallback={<div>Loading...</div>}>
<Switch>
<Route exact path='/' component={Home} />
<Route path='/about' component={About} />
</Switch>
</Suspense>
</Router>
);
}
Component-Level Code Splitting
Sometimes, you may want to dynamically load only a specific part of a component. This is particularly useful for heavy libraries or features that are not used frequently.
Consider the following example, where a heavy charting library is loaded only when the user interacts with a specific part of the UI:
import React, { useState, lazy, Suspense } from 'react';
const Chart = lazy(() => import('./Chart'));
function Dashboard() {
const [showChart, setShowChart] = useState(false);
return (
<div>
<button onClick={() => setShowChart(true)}>Show Chart</button>
{showChart && (
<Suspense fallback={<div>Loading chart...</div>}>
<Chart />
</Suspense>
)}
</div>
);
}
Lazy Loading with React-Query
React-Query is a powerful library for managing server-state in React applications. It pairs exceptionally well with lazy loading, allowing you to fetch data only when needed and perform background updates.
import { useQuery } from 'react-query';
import React, { lazy, Suspense } from 'react';
const UserComponent = lazy(() => import('./UserComponent'));
function Users() {
const { data, error, isLoading } = useQuery('users', fetchUsers);
if (isLoading) return <div>Loading...</div>;
if (error) return <div>Error loading data</div>;
return (
<Suspense fallback={<div>Loading user...</div>}>
{data.map(user => (
<UserComponent key={user.id} user={user} />
))}
</Suspense>
);
}
In this example, UserComponent
is loaded lazily and rendered only when the data fetch is complete.
Code Splitting with Webpack
Webpack is a popular bundler that supports code splitting out of the box. Here's how you can leverage Webpack's import()
syntax for dynamic imports:
const modulePromise = import('./module');
modulePromise.then(module => {
const myFunction = module.myFunction;
myFunction();
});
You can also use Webpack's SplitChunksPlugin
to achieve more granular control over code splitting:
module.exports = {
optimization: {
splitChunks: {
chunks: 'all',
minSize: 20000,
maxSize: 70000,
minChunks: 1,
maxAsyncRequests: 6,
maxInitialRequests: 4,
automaticNameDelimiter: '-',
cacheGroups: {
vendors: {
test: /[\\/]node_modules[\\/]/,
priority: -10
},
default: {
minChunks: 2,
priority: -20,
reuseExistingChunk: true
}
}
}
}
};
Best Practices for Code Splitting
Implementing code splitting and lazy loading requires adhering to best practices to ensure optimal performance:
- Analyze Bundle Sizes: Use tools like
webpack-bundle-analyzer
to visualize the size of your bundles and identify areas for improvement. - Minimal Fallbacks: Keep fallback UIs minimal to prevent excessive rendering times.
- Granular Module Division: Divide your application into granular modules, ensuring that each bundle is manageable and justified by its usage.
- Organize Routes: Carefully organize routes and group components based on common usage patterns.
- Lazy Load Non-critical Resources: Only lazy load non-critical resources that are not needed immediately on page load.
- Prefetching and Preloading: Use
React.Lazy
withReact.Suspense
for components that are likely to be used soon, ensuring they are pre-fetched in the background.
Conclusion
Advanced code splitting techniques and the dynamic import capabilities of ES modules can significantly optimize the performance and scalability of your React applications. By implementing these strategies, you can achieve faster load times, efficient resource utilization, and an enhanced user experience.
Stay ahead of the curve by embracing these advanced techniques and continuously analyzing and refining your bundling strategies. Happy coding!