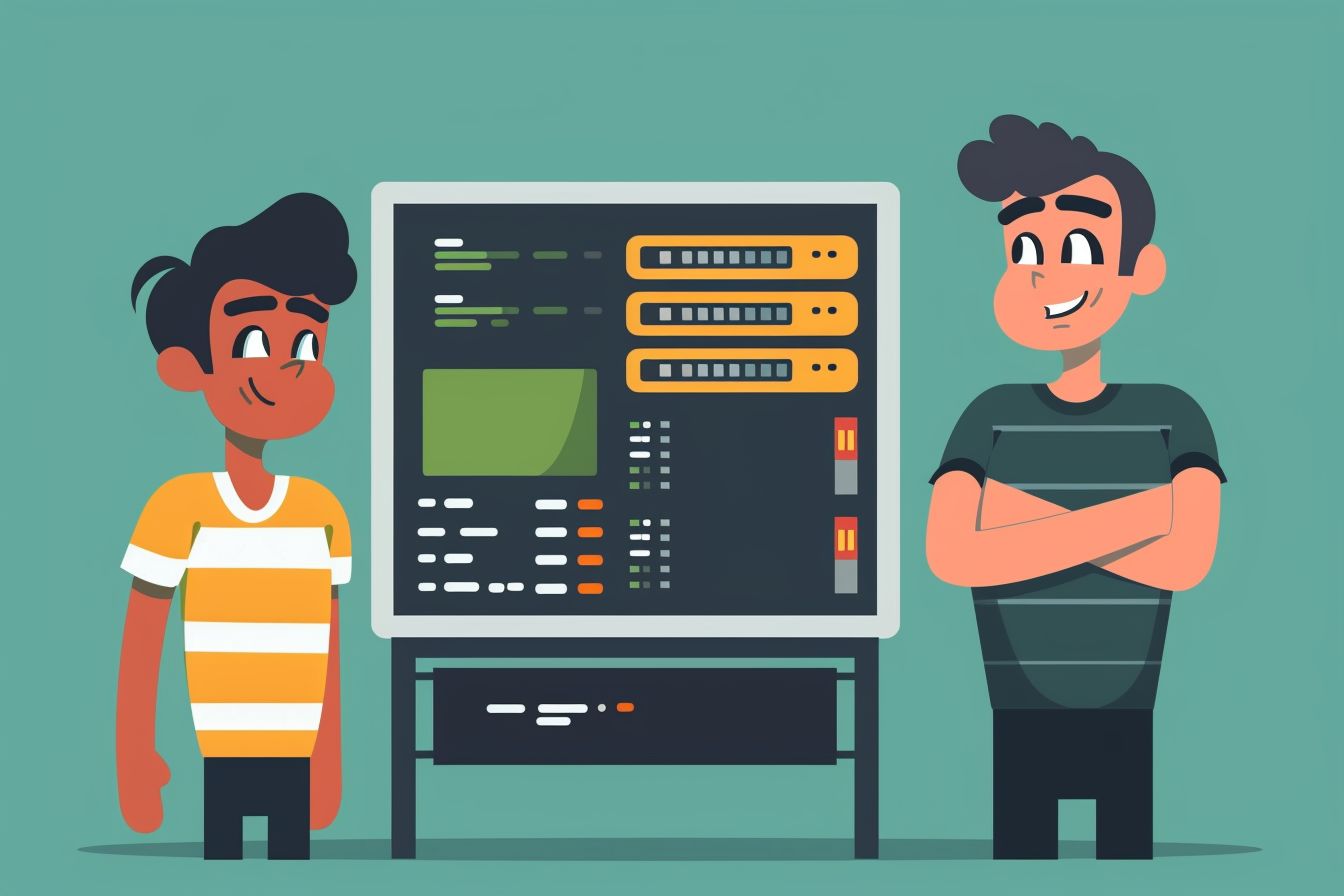
The Rise of React Server Components
Understanding SSR and Its Impact on Web Development
Jul 28, 2024 - 11:43 • 4 min read
Server Rendering vs Client Rendering
As web developers, we often find ourselves debating the merits of server-side rendering (SSR) against client-side rendering (CSR) in our applications. With the advent of frameworks like React Server Components, this conversation has gained renewed momentum.
What are Server Components?
React Server Components (RSC) are a new type of React component that render on the server and send serialized JSON to the client, enabling fast page loads and enhanced interactivity. By deferring rendering esoteric data until it's actually requested, React Server Components redefine the user- and developer-experience.
In contrast to traditional Client Components which execute entirely in the browser, Server Components promote a hybrid model where some elements of your application can remain on the server. This approach harnesses the best of both worlds by allowing SSR for critical components while offloading less important ones to the client.
Key Benefits of Server Components
- Performance: RSC promotes performance by only sending essential components to the browser. This not only leads to faster initial loads but also reduces the overall size of your JavaScript bundles.
- Data-fetching: Seamless server-side data-fetching prevents additional network round-trips and optimizes data requests based on rendered states. RSC allows you to retrieve data necessary for the components without sending additional fetch requests from the client.
- Accessibility to Server Resources: Server Components can access server resources directly, like databases and APIs, improving security and efficiency by reducing the risk of exposing sensitive endpoints to the client.
Challenges with Server Components
While RSC presents a significant advancement for React applications, it is crucial to approach its implementation with care. Here are some considerations:
- Component Structure: You can't use state, effects, or lifecycle methods in Server Components. Your component logic needs to shift from what you're accustomed to in Client Components. This shift may require substantial refactoring.
- Interop with Existing Components: There might be challenges when interoperating Server and Client Components. Careful planning of how data flows and rendering decisions are made is necessary to avoid pitfalls during composition.
How to Integrate Server Components with Your React App
Here’s a quick example to illustrate how to utilize Server Components in a React application:
Setting Up a New Environment: If you're starting a new React application, you may want to use frameworks like Next.js that provide built-in support for React Server Components. If you're adding RSC to an existing project, you might want to check compatibility first.
Creating a Server Component: Here's a basic outline of what a Server Component might look like:
// src/MyServerComponent.server.js export default async function MyServerComponent() { const data = await fetchDataFromAPI(); return <div>{data}</div>; }
This example shows a simplistic approach to fetching data directly in a server component, promoting the seamless data-fetching experience RSC allows.
Using the Component in Your App:
// src/App.js import MyServerComponent from './MyServerComponent.server'; function App() { return ( <div> <h1>Hello from the React App!</h1> <MyServerComponent /> </div> ); } export default App;
Combining with Client Components: When combining server and client components, keep your state in Client Components, while Server Components handle data-fetching:
// src/ClientComponent.js import MyServerComponent from './MyServerComponent.server'; function ClientComponent() { return ( <div> <MyServerComponent /> </div> ); }
Conclusion
React Server Components are a powerful solution reshaping how we think about rendering in React applications. By strategically implementing RSC, we can achieve faster performance, improved data handling, and a better user experience. Challenges accompany this promising shift but with careful consideration and thoughtful design, developers can leverage RSC to create robust, efficient applications.
Final Thoughts
By navigating the transition into this new paradigm, we can fundamentally improve our understanding of the full-stack capabilities of React. Stay tuned for more updates on React Server Components as they continue to evolve!