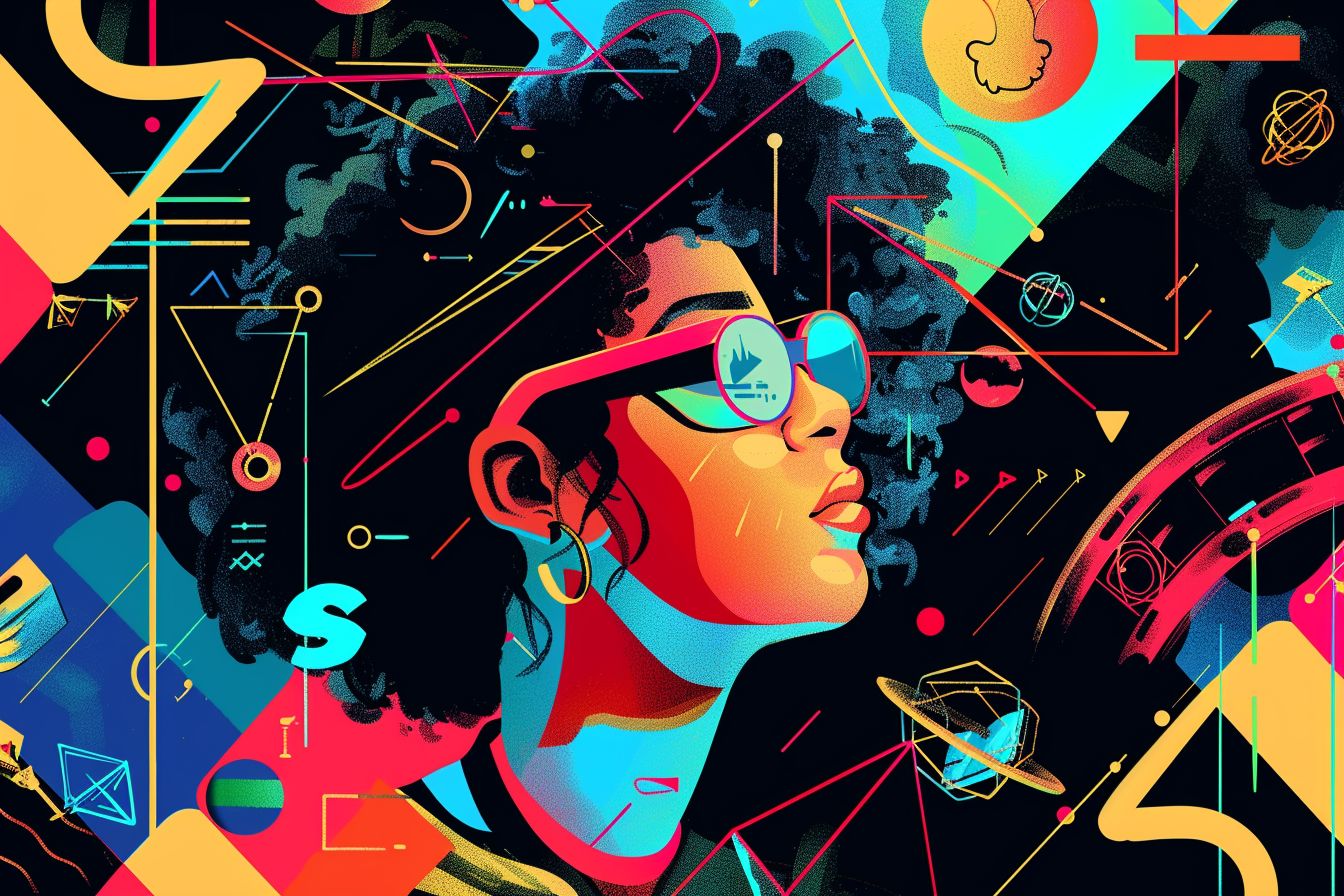
Leveraging Intersection Observer API with React for Enhanced Performance
Efficient Scrolling and Lazy Loading with Modern Web API
Jul 01, 2024 - 01:24 • 5 min read
Web development is continuously evolving, with new APIs and tools constantly emerging to improve performance and user experience. One such powerful tool is the Intersection Observer API, designed to detect when elements intersect with the viewport or a parent element. In this post, we'll dive deep into leveraging the Intersection Observer API within a React application, exploring practical use cases, best practices, and implementing advanced features.
Overview of Intersection Observer API
The Intersection Observer API provides a way to asynchronously observe changes in the intersection of a target element with an ancestor element or with a top-level document's viewport. This capability is instrumental in implementing features like infinite scrolling, lazy loading of images, and triggering animations as elements come into view.
Basic Syntax
Here's a basic example of how the Intersection Observer API can be used:
const observer = new IntersectionObserver((entries, observer) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
// Element is in view
}
});
}, {
root: null, // Use the viewport as the root
rootMargin: '0px',
threshold: 1.0 // Trigger when 100% of the element is in view
});
const targetElement = document.querySelector('.target');
observer.observe(targetElement);
Integrating Intersection Observer with React
Integrating this API with React involves creating custom hooks and providing a seamless, declarative experience for our components.
Creating a Custom Hook
Let's start by creating a custom React Hook to encapsulate the Intersection Observer logic:
import { useEffect, useRef } from 'react';
function useIntersectionObserver(callback, options) {
const ref = useRef();
useEffect(() => {
const observer = new IntersectionObserver((entries, observer) => {
entries.forEach(entry => callback(entry, observer));
}, options);
if (ref.current) {
observer.observe(ref.current);
}
return () => {
if (ref.current) {
observer.unobserve(ref.current);
}
};
}, [callback, options]);
return ref;
}
Usage Example in a Component
Now, let's use this custom hook within a React component to lazy load images:
import React, { useState } from 'react';
import useIntersectionObserver from './useIntersectionObserver';
function LazyImage({ src, alt }) {
const [isVisible, setIsVisible] = useState(false);
const ref = useIntersectionObserver((entry) => {
if (entry.isIntersecting) {
setIsVisible(true);
}
}, { threshold: 0.1 });
return <img ref={ref} src={isVisible ? src : ''} alt={alt} />;
}
Advanced Use Cases
Infinite Scrolling
Infinite scrolling is a popular technique where more content is loaded as the user scrolls down the page, without requiring explicit pagination actions. The Intersection Observer API can be leveraged to detect when the user has scrolled to the bottom of the page and trigger a fetch for more content:
import React, { useState, useEffect, useCallback } from 'react';
import useIntersectionObserver from './useIntersectionObserver';
function InfiniteScrollList({ fetchMoreData }) {
const [items, setItems] = useState([]);
const loadMoreRef = useIntersectionObserver((entry) => {
if (entry.isIntersecting) {
fetchMoreData().then(newItems => setItems([...items, ...newItems]));
}
}, { threshold: 1.0 });
useEffect(() => {
// Initial fetch
fetchMoreData().then(initialItems => setItems(initialItems));
}, [fetchMoreData]);
return (
<div>
{items.map(item => (
<div key={item.id}>{item.content}</div>
))}
<div ref={loadMoreRef} style={{ height: '20px' }}></div>
</div>
);
}
Animations on Scroll
Triggering animations as elements come into view enhances user engagement and interaction on the webpage. By using the Intersection Observer API with React, we can add scroll-triggered animations effortlessly:
import React, { useState } from 'react';
import useIntersectionObserver from './useIntersectionObserver';
import { CSSTransition } from 'react-transition-group';
function AnimatedComponent() {
const [isInView, setIsInView] = useState(false);
const ref = useIntersectionObserver((entry) => {
if (entry.isIntersecting) {
setIsInView(true);
}
}, { threshold: 0.5 });
return (
<CSSTransition in={isInView} classNames="fade" timeout={500}>
<div ref={ref} className="fade">
I animate into view!
</div>
</CSSTransition>
);
}
Best Practices and Advanced Tips
Throttling and Debouncing
When observing multiple elements, the callback can fire frequently and impact performance. Implementing throttling or debouncing mechanisms can mitigate this issue. You can utilize lodash's throttle
or debounce
functions:
import { throttle } from 'lodash';
const throttledCallback = throttle((entry) => {
if (entry.isIntersecting) {
// Handle intersection
}
}, 200);
const observer = new IntersectionObserver(throttledCallback, { threshold: 0.5 });
Observing Multiple Elements
Observing multiple elements of the same type can be handled efficiently by modifying our hook:
function useMultipleIntersectionObserver(callback, options, targets) {
useEffect(() => {
const observer = new IntersectionObserver((entries, observer) => {
entries.forEach(entry => callback(entry, observer));
}, options);
targets.forEach(target => observer.observe(target));
return () => {
targets.forEach(target => observer.unobserve(target));
};
}, [callback, options, targets]);
}
Usage:
function App() {
const targets = document.querySelectorAll('.observe');
useMultipleIntersectionObserver((entry) => {
if (entry.isIntersecting) {
// Handle intersection
}
}, { threshold: 0.1 }, targets);
return (
<div>
<div className="observe">Element 1</div>
<div className="observe">Element 2</div>
<div className="observe">Element 3</div>
</div>
);
}
Intersection Observer Polyfill
While widely supported, it's always good to account for older browsers. A polyfill can be used for broader compatibility:
npm install intersection-observer
Then, import it at the entry point of your application:
import 'intersection-observer';
Testing Your Intersection Observer Implementation
Testing Intersection Observer logic can be tricky but is crucial for ensuring robustness, especially for features like lazy loading and infinite scrolling. React Testing Library and Jest can be employed for this purpose. Mocking the Intersection Observer is essential for unit testing:
beforeEach(() => {
global.IntersectionObserver = class IntersectionObserver {
constructor() {}
observe() {}
unobserve() {}
disconnect() {}
};
});
// Your test cases...
Conclusion
The Intersection Observer API, combined with React, offers a powerful toolset for enhancing web performance and creating engaging user experiences. By converting the imperative API into a more declarative approach using custom hooks, we can seamlessly integrate advanced scrolling and lazy loading features into our applications. Embrace the Intersection Observer API and elevate your web development skills to new heights.
Happy coding!