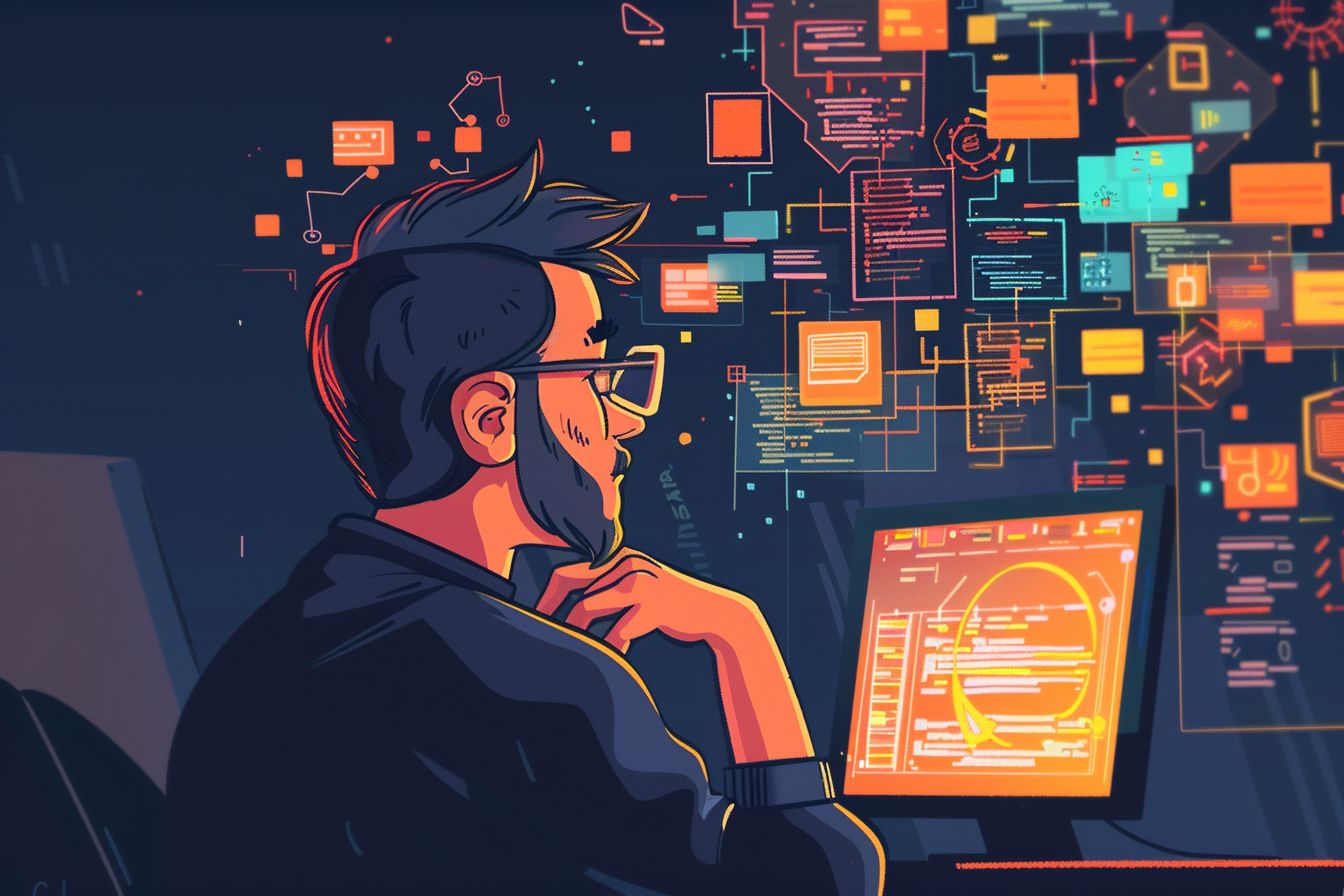
Harnessing TypeScript's Power with Advanced Utility Types in React
Mastering TypeScript utility types for cleaner, more maintainable React components
Jun 25, 2024 - 13:46 • 5 min read
Introduction
TypeScript has become an integral part of modern web development workflows, especially in large-scale applications where robust type checking can prevent numerous runtime errors. One of TypeScript"s most powerful features is its utility types which allow developers to transform types in ways that can greatly increase the maintainability and robustness of their code. In this blog post, we will deep dive into some advanced utility types and see how they can be effectively used in a React project.
Understanding Utility Types
TypeScript offers several built-in utility types that are incredibly powerful when dealing with complex type manipulations. Some of the most commonly used ones include Partial
, Required
, Readonly
, Record
, Pick
, and Omit
. However, there are more advanced utility types like Exclude
, Extract
, NonNullable
, ReturnType
, InstanceType
, and Parameters
that can redefine the way you handle types in your React projects.
Example Project Setup
Let"s consider a hypothetical React component that deals with user profiles:
import React from "react";
type User = {
id: string;
name: string;
email: string;
age?: number;
};
const UserProfile: React.FC<{ user: User }> = ({ user }) => {
return (
<div>
<h2>{user.name}</h2>
<p>Email: {user.email}</p>
{user.age && <p>Age: {user.age}</p>}
</div>
);
};
In the above snippet, we have a simple User
type and a UserProfile
component that accepts a prop of type User
. Now let"s start exploring how we can refine and use utility types to enhance this code.
Partial
Partial<Type>
constructs a type with all properties of Type set to optional. This is useful when you want to initialize an object with only a subset of its properties.
const userWithMissingProps: Partial<User> = {
id: "123",
name: "John Doe",
};
Required
Required<Type>
constructs a type consisting of all properties of Type set to required. This can be used to ensure that all properties are present in scenarios where they are required.
type FullUser = Required<User>;
const userWithAllProps: FullUser = {
id: "123",
name: "John Doe",
email: "[email protected]",
age: 25,
};
Readonly
Readonly<Type>
constructs a type with all properties of Type set to readonly
. This can be particularly useful in state management scenarios where immutability is desired.
const immutableUser: Readonly<User> = {
id: "123",
name: "John Doe",
email: "[email protected]",
age: 25,
};
Attempting to modify immutableUser
will now result in a type error:
// immutableUser.age = 26; // Error: Cannot assign to "age" because it is a read-only property.
Record
Record<Keys, Type>
constructs a type with a set of properties K of type T. This is useful for creating maps or dictionaries where keys and values both have specific types.
type UserRole = "admin" | "user" | "guest";
type Permissions = "read" | "write" | "delete";
type RolePermissions = Record<UserRole, Permissions[]>;
const rolePermissions: RolePermissions = {
admin: ["read", "write", "delete"],
user: ["read", "write"],
guest: ["read"],
};
Pick
Pick<Type, Keys>
constructs a type by picking a set of properties Keys from Type. This is useful for creating subsets of an interface.
type UserBaseInfo = Pick<User, "id" | "name">;
const userBase: UserBaseInfo = {
id: "123",
name: "John Doe",
};
Omit
Omit<Type, Keys>
constructs a type by picking all properties from Type and then removing Keys. This is the opposite of Pick
.
type UserWithoutEmail = Omit<User, "email">;
const userWithoutEmail: UserWithoutEmail = {
id: "123",
name: "John Doe",
age: 25,
};
Exclude
Exclude<Type, ExcludedUnion>
constructs a type by excluding from Type all unions that are assignable to ExcludedUnion. This is useful for creating types that contain subsets of other types.
type Status = "idle" | "loading" | "success" | "error";
type ErrorStatus = Exclude<Status, "idle" | "loading" | "success">; // "error"
Extract
Extract<Type, Union>
constructs a type that extracts from Type all unions that are assignable to Union. This is useful for creating types that contain unions of other types.
type ApiResponse = "success" | "error" | "pending";
type SuccessResponse = Extract<ApiResponse, "success">; // "success"
NonNullable
NonNullable<Type>
constructs a type by excluding null
and undefined
from Type. This is useful for ensuring that values are always present.
type NullableUser = User | null;
type NonNullableUser = NonNullable<NullableUser>;
Attempting to use null
or undefined
with NonNullableUser
will result in a type error:
const user: NonNullableUser = null; // Error: Type "null" is not assignable to type "NonNullable<User>".
Parameters
Parameters<Type>
constructs a tuple type that represents the types of the parameters of a function type. This is useful for inferring parameter types.
function updateUser(id: string, name: string, email: string): User {
return { id, name, email };
}
type UpdateUserParams = Parameters<typeof updateUser>; // [string, string, string]
ReturnType
ReturnType<Type>
constructs a type consisting of the return type of function Type. This is useful for inferring return types without explicitly defining them.
type UpdateUserReturnType = ReturnType<typeof updateUser>; // User
InstanceType
InstanceType<Type>
constructs a type consisting of the instance type of a constructor function Type. This is useful for creating object instances from classes.
class UserClass {
constructor(public id: string, public name: string, public email: string, public age?: number) {}
}
type UserInstance = InstanceType<typeof UserClass>;
const userInstance: UserInstance = new UserClass("123", "John Doe", "[email protected]", 25);
Conclusion
Advanced utility types in TypeScript provide us with a highly flexible and powerful toolbox for handling complex typing scenarios. By leveraging these utilities, you can write cleaner, more maintainable, and more robust React components. Understanding and using these types can significantly enhance your coding experience, making your applications more reliable and easier to manage.
If you haven"t yet explored the depths of TypeScript utility types, I highly encourage you to start incorporating them into your workflow. The benefits they bring can dramatically improve your code quality and developer experience.