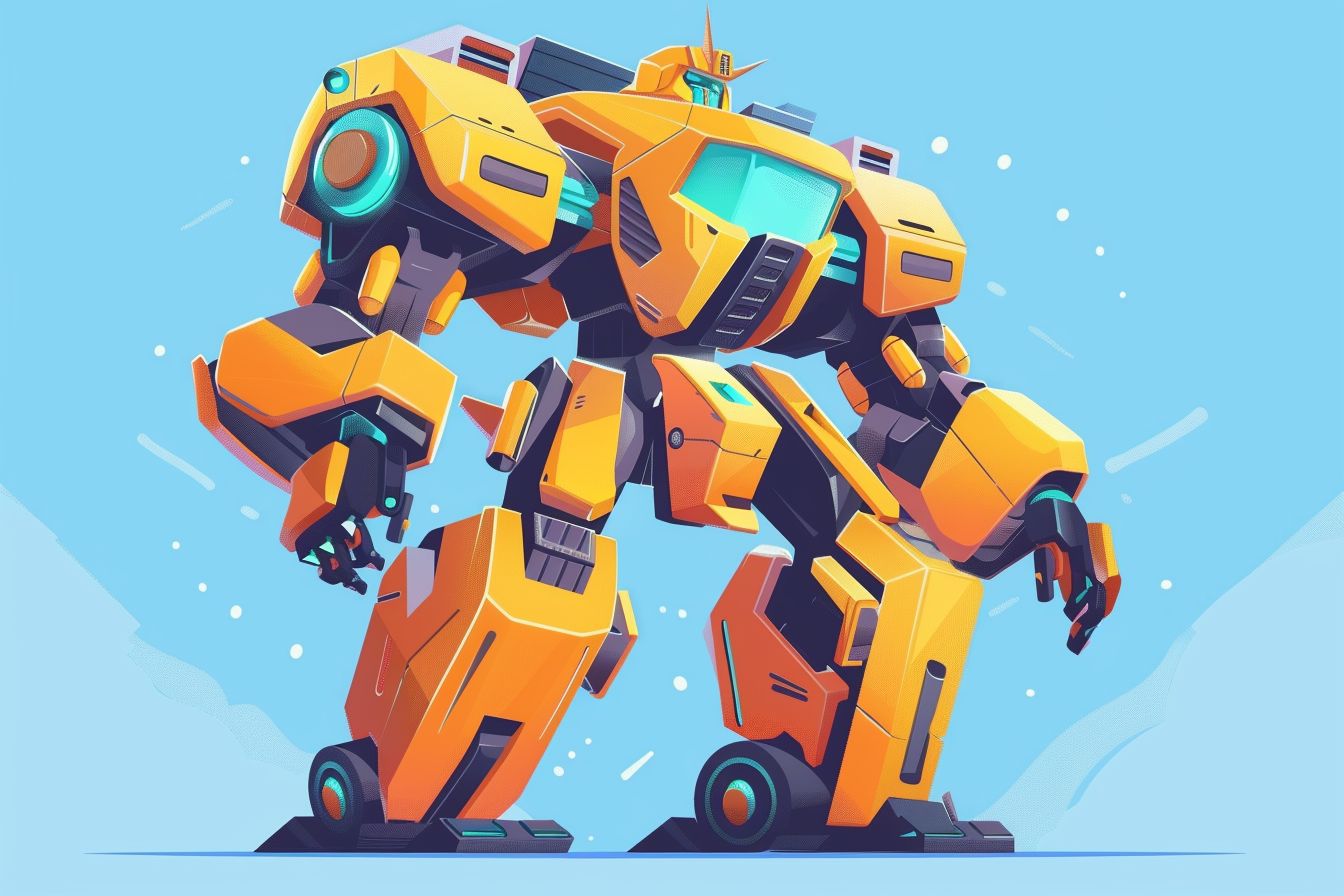
Crafting Polymorphic Components in React
Exploring Advanced Polymorphism Patterns for Scalable React Components
Jun 30, 2024 - 21:21 • 4 min read
Crafting Polymorphic Components in React
Component reusability is at the heart of React development. One of the advanced patterns you might encounter in systematizing your components is polymorphic components. Polymorphic components are designed to render different elements based on the provided props, achieving higher flexibility and reusability.
In this article, we will dive deep into creating polymorphic components, illustrate why they are useful, and discuss best practices. As a practical example, we'll build a polymorphic button component and explore its potential.
What Are Polymorphic Components?
In essence, polymorphic components are components that can render different HTML elements or even other React components based on the props they receive. This concept leverages React's powerful composability and facilitates creating components that are more flexible and reusable.
Why Use Polymorphic Components?
- Consistency: Ensures a uniform appearance across various UI elements.
- Reusability: Promotes the reuse of logic and styles in different contexts.
- Flexibility: Allows components to adapt to different requirements without redundant code.
Building a Polymorphic Button Component
Let’s start by defining the types for our polymorphic component using TypeScript. While JavaScript alone can certainly handle polymorphism, TypeScript enhances the development experience with type safety and better tooling.
Step 1: Define the Component Types
We need a base type for the as
prop, which determines what element will be rendered. Let’s create a utility type for managing our polymorphic component’s props.
import { ElementType, ComponentPropsWithoutRef, ReactNode } from 'react';
interface PolymorphicComponentProps<E extends ElementType> {
as?: E;
children?: ReactNode;
}
Step 2: Extend the Type for Props
We need to extend our props type to include attributes specific to the element type provided through the as
prop.
export type PolymorphicProps<
E extends ElementType,
P = {}
> = P & PolymorphicComponentProps<E> & Omit<ComponentPropsWithoutRef<E>, keyof PolymorphicComponentProps<E>>;
Step 3: Implement the Polymorphic Component
With the types defined, let’s implement our polymorphic button component. This component will render the element specified by the as
prop while passing along any additional props.
import React from 'react';
function PolymorphicButton<E extends ElementType = 'button'>(
{ as, children, ...props }: PolymorphicProps<E>
) {
const Component = as || 'button';
return <Component {...props}>{children}</Component>;
}
export default PolymorphicButton;
Step 4: Usage Examples
Let’s look at how you can use the polymorphic button component in different scenarios.
Example 1: As a Button
import PolymorphicButton from './PolymorphicButton';
const App = () => (
<PolymorphicButton onClick={() => alert('Clicked!')}>Click Me</PolymorphicButton>
);
Example 2: As an Anchor Tag
import PolymorphicButton from './PolymorphicButton';
const App = () => (
<PolymorphicButton as='a' href="https://example.com">Go to Example</PolymorphicButton>
);
Step 5: Adding Default Props and Prop Types
To make our component more user-friendly, we can add default props and use PropTypes for non-TypeScript projects.
PolymorphicButton.defaultProps = {
as: 'button',
};
Customizing Styles with Tailwind CSS
Now, let’s look at how you can integrate Tailwind CSS for scalable and maintainable styling.
Example 3: Styled Button with Tailwind CSS
import './tailwind.output.css';
import PolymorphicButton from './PolymorphicButton';
const App = () => (
<PolymorphicButton className="bg-blue-500 text-white p-2 rounded">
Click Me
</PolymorphicButton>
);
Wrapping Up
Polymorphic components can significantly improve the flexibility and reusability of your UI. They allow you to write more adaptable and maintainable components, streamlining the development process and enhancing consistency across your application.
With TypeScript, you get additional safety and tooling advantages, making the development experience even better. Combined with utility-first CSS frameworks like Tailwind, you can effortlessly manage styles and extend the look and feel of your component with ease.
Stay tuned for more advanced React techniques and happy coding!