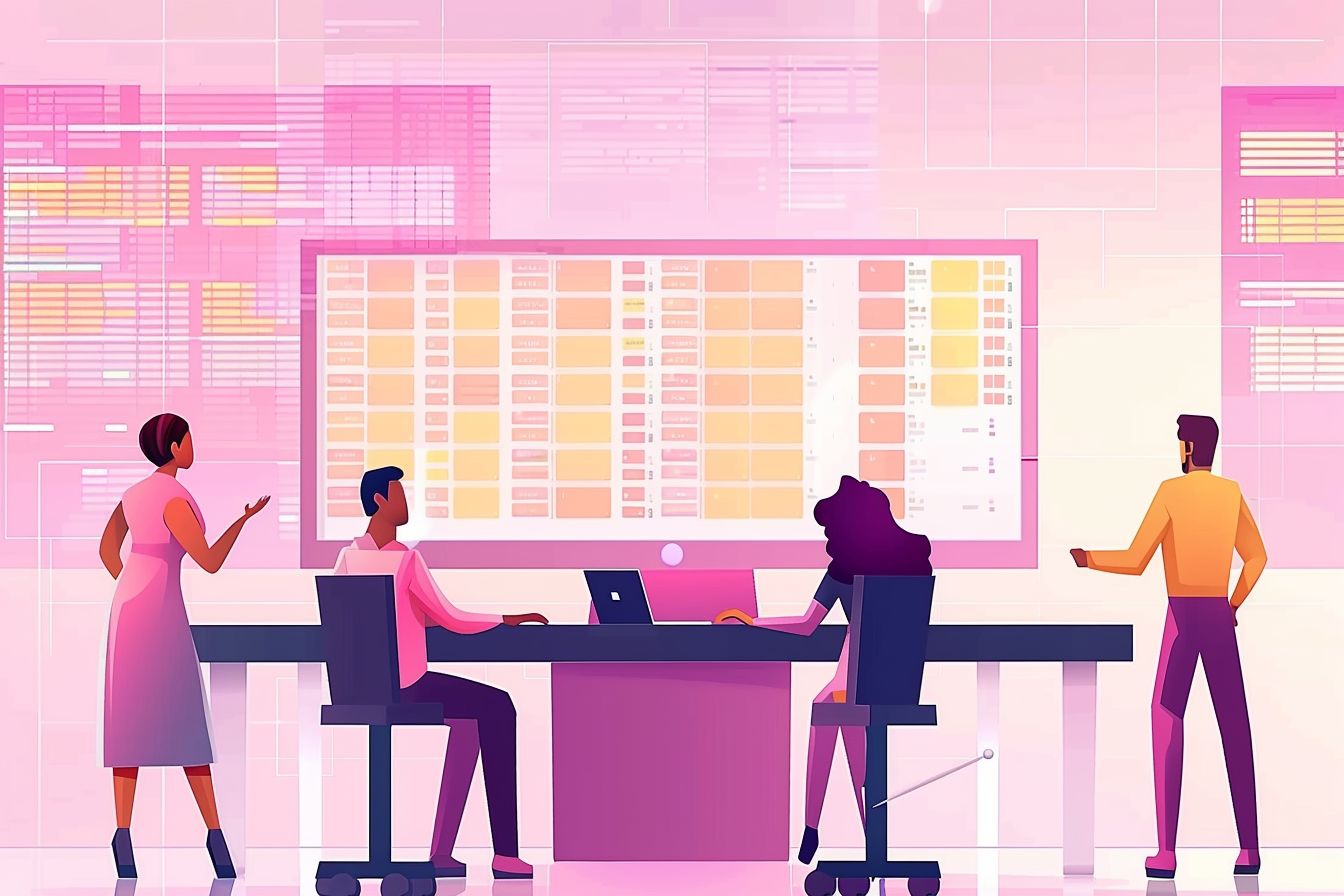
Deep Dive into Building Dynamic Data Tables with React and Ag-Grid
Harnessing the Power of Ag-Grid for Complex Data Visualization in React
Jul 09, 2024 - 23:39 • 4 min read
Deep Dive into Building Dynamic Data Tables with React and Ag-Grid
The ability to handle and display complex data sets efficiently is crucial in modern web development. Ag-Grid is one of the most powerful data grid libraries for React, providing a plethora of features for building interactive data tables. In this post, we'll explore advanced techniques for using Ag-Grid in React applications.
Why Ag-Grid?
Ag-Grid stands out because of its rich feature set, which includes sorting, filtering, pagination, inline editing, and custom cell rendering. These features can be customized extensively, making Ag-Grid a perfect choice for enterprise-level applications.
Setting Up Ag-Grid with React
npm install ag-grid-react ag-grid-community
Next, let's set up a basic Ag-Grid in a React component.
import React from 'react';
import { AgGridReact } from 'ag-grid-react';
import 'ag-grid-community/styles/ag-grid.css';
import 'ag-grid-community/styles/ag-theme-alpine.css';
const MyDataGrid = () => {
const columnDefs = [
{ headerName: 'ID', field: 'id' },
{ headerName: 'Name', field: 'name' },
{ headerName: 'Age', field: 'age' }
];
const rowData = [
{ id: 1, name: 'John', age: 25 },
{ id: 2, name: 'Doe', age: 30 },
{ id: 3, name: 'Smith', age: 35 }
];
return (
<div className="ag-theme-alpine" style={{ height: 400, width: 600 }}>
<AgGridReact columnDefs={columnDefs} rowData={rowData} />
</div>
);
};
export default MyDataGrid;
Custom Cell Renderers
Custom cell renderers allow you to customize how individual cells are displayed. This is especially useful for complex data types or when you need to include interactive elements within cells.
Creating a Custom Cell Renderer
const CustomRenderer = (props) => {
return (
<span>
<b>{props.value}</b>
</span>
);
};
const columnDefs = [
{ headerName: 'ID', field: 'id' },
{ headerName: 'Name', field: 'name', cellRendererFramework: CustomRenderer },
{ headerName: 'Age', field: 'age' }
];
Dynamic Data Loading with Infinite Scroll
Ag-Grid supports infinite scrolling, which is ideal for large datasets. You can implement infinite scrolling by interfacing with a server-side data source.
const MyInfiniteScrollGrid = () => {
const columnDefs = [
{ headerName: 'ID', field: 'id' },
{ headerName: 'Name', field: 'name' },
{ headerName: 'Age', field: 'age' }
];
const datasource = {
getRows: (params) => {
fetch('https://api.example.com/data') // Replace with your data source
.then((resp) => resp.json())
.then((data) =>
params.successCallback(data.rows, data.lastRow)
);
}
};
return (
<div className="ag-theme-alpine" style={{ height: 400, width: 600 }}>
<AgGridReact columnDefs={columnDefs} rowModelType="infinite" datasource={datasource} />
</div>
);
};
Best Practices for Infinite Scrolling
- Optimize API Calls: Ensure your API supports pagination and returns only the necessary data for each request.
- Throttle or Debounce Requests: To prevent overloading your server, consider throttling or debouncing the API requests triggered by scrolling.
Advanced Filtering and Sorting
Ag-Grid's filtering and sorting capabilities can be customized to fit specific needs. You can create custom filter components or use the built-in mechanisms.
Custom Filtering
const CustomFilter = (props) => {
const [filterText, setFilterText] = React.useState('');
const onFilterChanged = () => {
props.filterChangedCallback();
};
return (
<div>
<input
type="text"
value={filterText}
onChange={(e) => {
setFilterText(e.target.value);
onFilterChanged();
}}
/>
</div>
);
};
const columnDefs = [
{ headerName: 'ID', field: 'id' },
{ headerName: 'Name', field: 'name' , filterFramework: CustomFilter},
{ headerName: 'Age', field: 'age' }
];
Inline Editing
Ag-Grid provides powerful inline editing capabilities. You can use default editors or create custom editors.
Default Inline Editing
const columnDefs = [
{ headerName: 'ID', field: 'id', editable: true },
{ headerName: 'Name', field: 'name', editable: true },
{ headerName: 'Age', field: 'age', editable: true }
];
Custom Inline Editing
For more advanced scenarios, you can create custom cell editors.
const CustomEditor = (props) => {
const [value, setValue] = React.useState(props.value);
const onChange = (e) => {
setValue(e.target.value);
props.stopEditing();
};
return (
<input type="text" value={value} onChange={onChange} />
);
};
const columnDefs = [
{ headerName: 'ID', field: 'id' },
{ headerName: 'Name', field: 'name', editable: true, cellEditorFramework: CustomEditor },
{ headerName: 'Age', field: 'age' }
];
Performance Optimization
When dealing with large data sets and complex grid setups, performance can become a concern. Here are a few tips for optimizing Ag-Grid.
- Row Buffer: Increase the row buffer to enhance scrolling performance.
rowBuffer: 20
- Change Detection: Use
getRowNodeId
to let Ag-Grid track rows more efficiently.
getRowNodeId: data => data.id
- Lazy Loading: Load data only when needed, especially for large datasets.
Conclusion
Ag-Grid is an incredibly powerful tool for building dynamic, highly interactive data tables in React. By leveraging its extensive features and customization options, you can create user-friendly and performance-optimized data grids that meet complex requirements. Whether you're dealing with large data sets, need advanced customizations, or require real-time data updates, Ag-Grid has you covered.